Taming Database Schemas with Laravel Migrations: An In-depth Exploration
Laravel, a highly expressive PHP framework, has greatly simplified the process of web application development. This is why businesses increasingly opt to hire Laravel developers for their projects. One of the powerful features of Laravel that developers appreciate is Laravel Migrations, a tool that makes database schema management a breeze.
In this blog post, we will dissect Laravel Migrations, explore its capabilities, and show you why you might want to hire Laravel developers to effectively leverage this tool. You’ll gain insights through practical examples, showing you how Laravel Migrations can simplify and enhance your web application development process.
What are Laravel Migrations?
Laravel Migrations are like version control for your database. They allow you to manage your database structure in a programmatic way, enabling you to create, update, or drop tables and add, update, or delete columns in existing tables. The benefit is that this process is done using PHP code rather than writing SQL queries manually.
Migrations are especially useful in a team environment where you need to share the database schema changes among team members. Instead of sharing SQL queries, you share migration files which are versioned and can be rolled back if something goes wrong.
Let’s start with the basics.
Setting Up Laravel Migrations
Migrations are usually located in the `database/migrations` directory. Laravel provides a simple command-line utility called Artisan to generate migration files.
Assume we want to create a table called ‘users’, we can use the following command:
```php php artisan make:migration create_users_table ```
This command creates a new migration file, prefixed with a timestamp, inside the `database/migrations` directory.
Anatomy of a Migration File
A migration file contains two methods – `up` and `down`. The `up` method is used to add new tables, columns, or indexes to your database, while the `down` method is used to reverse the operations done by the `up` method.
```php <?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateUsersTable extends Migration { public function up() { Schema::create('users', function (Blueprint $table) { $table->id(); $table->string('name'); $table->string('email')->unique(); $table->timestamp('email_verified_at')->nullable(); $table->string('password'); $table->rememberToken(); $table->timestamps(); }); } public function down() { Schema::dropIfExists('users'); } } ```
In the `up` method, we use the `Schema` facade’s `create` method to create a new table. We pass in the name of the table (‘users’ in this case) and a closure that receives a `Blueprint` object used to define the new table.
Running Migrations
To run all of your outstanding migrations, you may use the migrate command:
```php php artisan migrate ```
When you run the `migrate` command, Laravel will execute the `up` method in your migration classes.
Rolling Back Migrations
If you need to rollback the latest migration operation, you can use the `migrate:rollback` command:
```php php artisan migrate:rollback ```
This command will execute the `down` method in your migration classes, effectively reversing the actions performed in the `up` method.
Modifying Existing Tables
Let’s suppose you want to add a new column `phone_number` to the `users` table. You can generate a new migration with:
```php php artisan make:migration add_phone_number_to_users_table ```
Then in the newly created migration file:
```php <?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class AddPhoneNumberToUsersTable extends Migration { public function up() { Schema::table('users', function (Blueprint $table) { $table->string('phone_number')->nullable(); }); } public function down() { Schema::table('users', function (Blueprint $table) { $table->dropColumn('phone_number'); }); } } ```
Run the migration with `php artisan migrate`, and Laravel will add the `phone_number` column to the `users` table.
Managing Migrations In Different Environments
Laravel offers you the flexibility of managing migrations in different environments. Suppose you have migrations that you want to run only in the local development environment and not in production. Laravel’s migrate command includes a `–path` option that you can leverage:
```php php artisan migrate --path=/database/migrations/my_local_migrations ```
With this command, only migrations in the specified directory will be executed.
Conclusion
Laravel Migrations provide an efficient way to manage database schema changes and versioning in your application. This feature is one reason why many companies choose to hire Laravel developers. The framework not only frees developers from writing SQL statements manually but also helps in maintaining consistency across different environments. Laravel Migrations are easy to understand and use, thereby streamlining the application development process.
Always remember, with great power comes great responsibility. While Laravel Migrations make it easy to change database schemas, improper use can result in data loss or corruption. Therefore, hiring experienced Laravel developers becomes even more crucial. It’s always essential to back up your database before running migrations, especially in production.
With the powerful toolset that Laravel provides, there are no limits to what you can build. Hiring expert Laravel developers can help you leverage these tools to their maximum potential. Happy coding!
Table of Contents
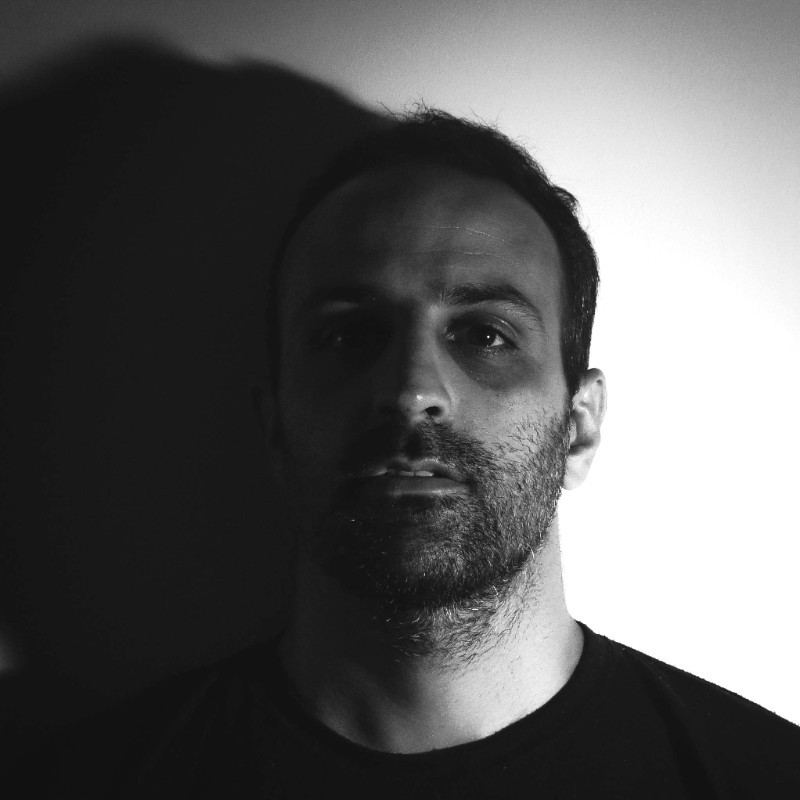
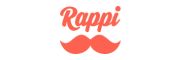