Decoding Laravel: Expert Insights into Debugbar and App Performance
One of the perks of using Laravel, a widely adopted PHP framework, is the abundance of tools available for debugging and profiling. One of the most popular among these tools is Laravel Debugbar. Designed to provide insightful information about your application’s performance, Laravel Debugbar offers a real-time look into your application’s inner workings.
In this post, we’ll dive deep into the Laravel Debugbar, exploring its features and showing how you can leverage them to profile and debug your applications effectively.
1. Installation and Configuration
To get started, you need to install the package via Composer:
```bash composer require barryvdh/laravel-debugbar --dev ```
After installation, the package will automatically be discovered by Laravel. For older versions of Laravel (before 5.5), you need to manually add the ServiceProvider and Facade alias:
```php 'providers' => [ //... Barryvdh\Debugbar\ServiceProvider::class, ] 'aliases' => [ //... 'Debugbar' => Barryvdh\Debugbar\Facade::class, ] ```
2. Basic Usage
Once installed, Debugbar will automatically inject itself at the bottom of your HTML pages when you’re in a local environment. You’ll see a bar offering various insights, including:
– Routes – Currently matched route details.
– Views – Views loaded for the page.
– Queries – Database queries executed.
– Memory – Memory usage.
– Timeline – Execution time of various parts of your application.
3. Digging Deeper with Examples
3.1. Monitoring Database Queries
One of the most powerful features is monitoring DB queries. For instance, if you have the following Eloquent query:
```php $users = User::where('name', 'John')->get(); ```
Debugbar will display:
– The SQL statement: `SELECT * FROM users WHERE name = ‘John’`.
– The execution time.
– If there are any duplicate queries, they will be highlighted, prompting you to optimize.
3.2 Tracking Views
Loading too many views or nesting them inefficiently can slow down an application. Debugbar shows you each loaded view and its data, allowing you to pinpoint problematic views.
3.3. Messaging & Alerts
You can add custom messages to Debugbar:
```php Debugbar::info($object); Debugbar::error('Error!'); Debugbar::warning('Watch out…'); Debugbar::addMessage('Another message', 'mylabel'); ```
This is especially handy when you want to trace the flow of code or check variable contents without stopping execution.
3.4 Profiling
Debugbar has a built-in profiler. Just wrap the code you want to profile with:
```php Debugbar::startMeasure('render','Time for rendering'); // Code to profile Debugbar::stopMeasure('render'); ```
You’ll get an accurate time of execution, helping you pinpoint bottlenecks.
4. Advanced Features
4.1 Custom Data Collectors
While Laravel Debugbar already provides tons of data, sometimes you might want to track specific data unique to your application. Debugbar allows you to add custom data collectors.
```php Debugbar::putCollector(new CustomCollector()); ```
4.2. AJAX Requests
Debugbar automatically captures AJAX requests and their responses, which is essential for SPAs or applications relying heavily on AJAX.
4.3 Exceptions and Stack Traces
When an exception is thrown, Debugbar captures it, showing the message, code, file, and stack trace, making it easier to debug.
5. Turning Off Debugbar
While Debugbar is a great tool for local development, you certainly don’t want it running in production. By default, Debugbar will be turned off when your APP_DEBUG is set to false. However, you can control its visibility by using:
```php Debugbar::enable(); Debugbar::disable(); ```
Conclusion
Laravel Debugbar is an indispensable tool for Laravel developers, offering a clear view into the application’s performance and inner workings. By making the most of its features, you can quickly identify bottlenecks, redundant queries, or other issues that might be slowing down your application. With Debugbar by your side, profiling and debugging Laravel applications has never been easier. Happy coding!
Table of Contents
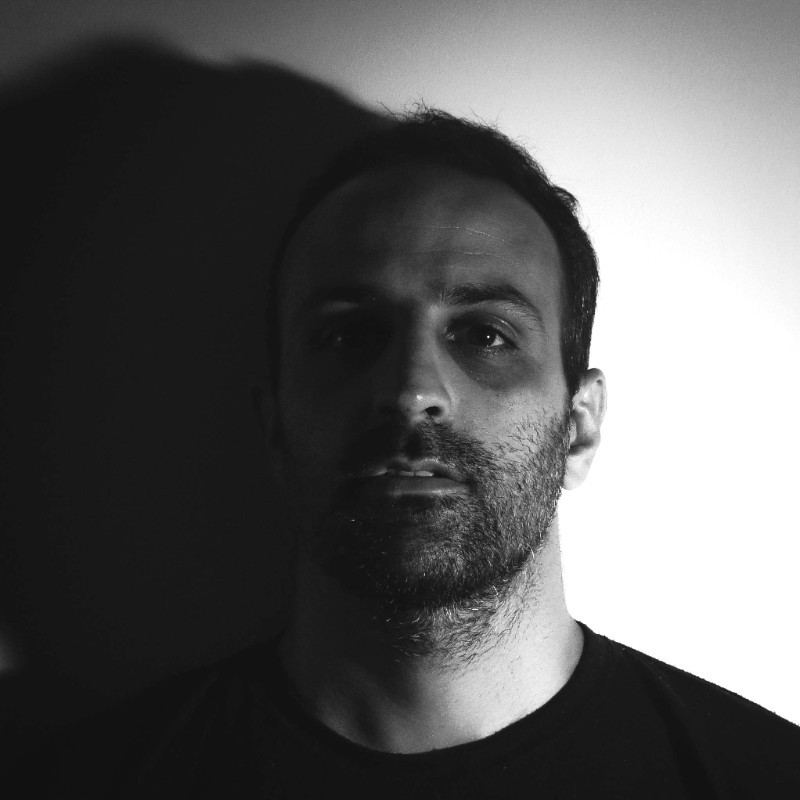
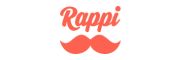