Laravel Debugging: Troubleshooting Techniques and Tools
Debugging is an essential aspect of the development process, helping developers identify and fix issues within their code. When working with Laravel, a popular PHP framework, effective debugging techniques and tools are crucial for maintaining a smooth and efficient development workflow. In this blog post, we’ll delve into various approaches to debugging Laravel applications, exploring troubleshooting techniques and highlighting essential tools that can aid developers in diagnosing and resolving issues effectively.
Table of Contents
1. Introduction
Debugging involves the process of identifying and rectifying errors, bugs, and unexpected behaviors within a software application. Laravel, known for its elegant syntax and powerful features, is no exception when it comes to needing debugging. By mastering debugging techniques and utilizing appropriate tools, developers can save time, improve code quality, and enhance the overall user experience.
2. Setting Up a Debugging Environment
Before diving into debugging techniques, it’s essential to establish a suitable environment for effective troubleshooting. Here are two fundamental steps to get you started:
2.1. Enabling Debug Mode
In Laravel, the debug configuration option should be set to true within the config/app.php file when working in a local development environment. This enables more detailed error messages and stack traces, aiding in pinpointing issues.
php 'local' => [ 'debug' => true, // ... ],
2.2. Leveraging Development Tools
Modern browsers come equipped with powerful developer tools that can assist in debugging JavaScript, CSS, and network-related issues. Additionally, Laravel’s integration with these tools allows developers to inspect network requests, view console logs, and monitor application performance.
3. Common Laravel Debugging Techniques
3.1. Debugging with dd() and dump()
The dd() and dump() functions are invaluable for inspecting variables and their values at specific points in your code. dump() provides a simple output of variable contents, while dd() goes a step further by halting the script’s execution and providing a more detailed view of the variable.
php $name = 'John Doe'; dump($name); // Output: "John Doe" $age = 25; dd($age); // Script execution halted, variable details displayed
3.2. Utilizing Laravel Debugbar
Laravel Debugbar is a third-party package that offers a comprehensive toolbar for debugging Laravel applications. It provides insights into queries, views, routes, and more. Installation is straightforward using Composer:
bash composer require barryvdh/laravel-debugbar --dev
After installation, the Debugbar will be accessible when running your application locally. It offers detailed information about the executed queries, loaded views, and more, helping you identify performance bottlenecks and other issues.
3.3. Logging and Viewing Log Files
Laravel’s built-in logging mechanism allows you to record information, warnings, errors, and other messages. By default, logs are stored in the storage/logs directory. Using log levels, you can categorize messages based on their severity.
php Log::info('This is an informational message.'); Log::warning('This is a warning message.'); Log::error('This is an error message.');
Viewing log files can be done through the command line or by utilizing third-party log viewers for a more user-friendly experience. This approach helps trace application flow and identify issues that might not be immediately apparent during development.
3.4. Exception Handling
Laravel’s exception handling mechanism simplifies error management by providing a centralized location for handling exceptions. The app/Exceptions/Handler.php file contains methods for different exception types. You can customize how exceptions are reported and rendered to suit your application’s needs.
php public function render($request, Throwable $exception) { if ($exception instanceof NotFoundHttpException) { return response()->view('errors.404', [], 404); } return parent::render($request, $exception); }
4. Advanced Debugging Tools
4.1. Xdebug
Xdebug is a powerful PHP extension that facilitates advanced debugging capabilities. It allows you to set breakpoints, step through code, inspect variables, and profile code execution. To use Xdebug with Laravel, you’ll need to install the extension and configure your environment.
4.2. Telescope
Laravel Telescope is an official debugging and profiling tool provided by Laravel. It offers a detailed view of requests, exceptions, database queries, and more. Telescope’s elegant dashboard provides real-time insights into your application’s behavior, making it an excellent choice for diagnosing complex issues.
To install Telescope, use Composer:
bash composer require laravel/telescope --dev php artisan telescope:install
4.3. Clockwork
Clockwork is a developer tool for debugging and profiling PHP applications, including Laravel projects. It provides insights into HTTP requests, database queries, and more, helping developers identify performance bottlenecks and optimization opportunities. Clockwork’s user interface is intuitive and easy to navigate.
To integrate Clockwork, install the package via Composer:
bash composer require itsgoingd/clockwork
5. Profiling and Performance Optimization
Effective debugging not only involves identifying and fixing bugs but also optimizing your application’s performance. Profiling tools like Laravel Telescope and Clockwork can help pinpoint areas of your code that require optimization. By analyzing database queries, response times, and memory usage, you can fine-tune your application for better efficiency.
Additionally, thorough testing and monitoring of your application in various scenarios can uncover performance bottlenecks that might not be apparent during development.
Conclusion
Debugging Laravel applications is a crucial skill that every developer should master. By employing techniques such as using dd() and dump(), leveraging Laravel Debugbar, and integrating advanced tools like Xdebug, Telescope, and Clockwork, you can efficiently troubleshoot and resolve issues within your codebase. Remember that effective debugging not only leads to smoother development but also results in high-quality applications that provide an exceptional user experience. So, embrace debugging as an integral part of your development process and watch your Laravel projects thrive.
In this blog post, we’ve explored various debugging techniques and tools for Laravel applications. Whether you’re a beginner or an experienced developer, integrating these practices into your workflow can save you time and frustration while ensuring the reliability and performance of your projects. Happy debugging!
Table of Contents
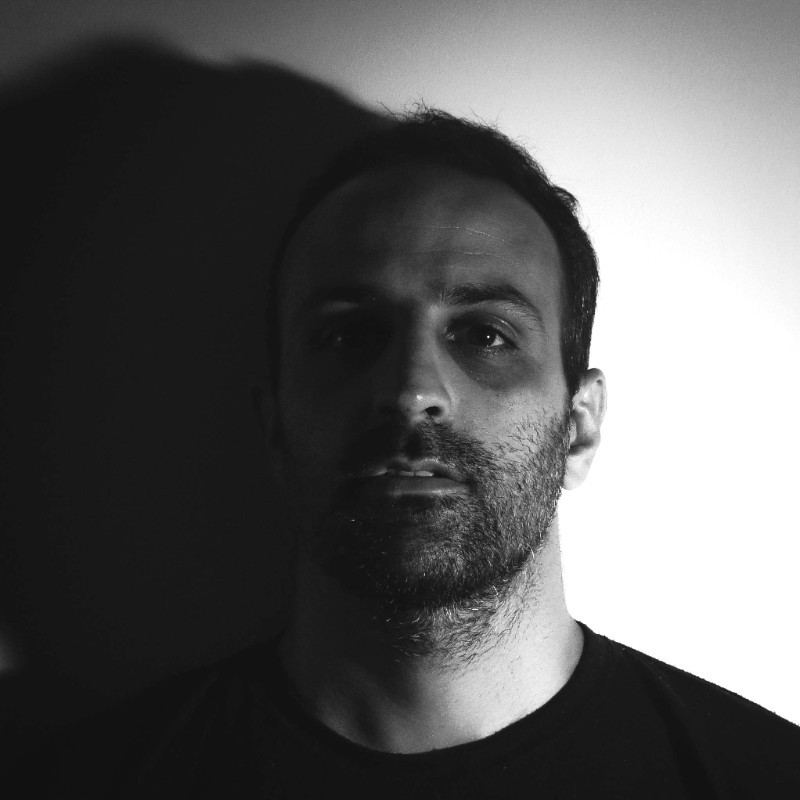
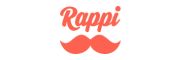