Laravel Deployment Strategies: From Development to Production
Deploying a Laravel application from development to production requires careful planning and implementation. A smooth deployment process ensures that your application is delivered efficiently and effectively to end-users. In this comprehensive guide, we will explore various deployment strategies for Laravel applications, providing code samples and step-by-step instructions to help you streamline the transition from development to production.
Continuous Integration and Continuous Deployment (CI/CD)
One of the most effective strategies for Laravel deployment is utilizing Continuous Integration and Continuous Deployment (CI/CD) practices. CI/CD allows for automated building, testing, and deployment of your Laravel application, ensuring that changes are thoroughly validated before reaching production.
With tools like Jenkins, GitLab CI/CD, or Travis CI, you can set up pipelines that automatically trigger the deployment process whenever changes are pushed to the main branch of your Git repository. Here’s an example of a simple .gitlab-ci.yml file for GitLab CI/CD:
yaml stages: - build - test - deploy build: stage: build script: - composer install --no-interaction --no-ansi --no-progress --no-suggest --no-scripts - npm ci - cp .env.example .env - php artisan key:generate test: stage: test script: - php artisan test deploy: stage: deploy script: - php artisan migrate --force - php artisan config:cache - php artisan route:cache - php artisan optimize
In this example, the pipeline performs the necessary build steps, such as installing dependencies, generating the environment file, and running tests. Finally, it deploys the application by running migrations and caching configuration, routes, and optimizations.
Git-Based Deployments
Deploying your Laravel application directly from a Git repository simplifies the deployment process and ensures version control. By using Git-based deployments, you can easily roll back to a previous working version if issues arise in production.
Here’s an example of a deployment script that pulls the latest changes from a Git repository and sets up the Laravel application:
bash # Assuming you have SSH access to the server ssh user@server 'cd /path/to/laravel && git pull origin main' cd /path/to/laravel composer install --no-dev --optimize-autoloader php artisan migrate --force php artisan config:cache php artisan route:cache php artisan optimize
This script connects to the server via SSH, navigates to the Laravel project directory, pulls the latest changes from the Git repository, and then runs the necessary commands for setting up the application.
Server Configuration Management
Managing server configurations using tools like Ansible, Puppet, or Chef simplifies the deployment process and ensures consistency across multiple environments. These tools allow you to define and automate the configuration of servers, making it easier to set up and deploy Laravel applications.
Consider the following Ansible playbook snippet for setting up a Laravel application:
yaml - hosts: production tasks: - name: Clone Git repository git: repo: git@example.com:username/repo.git dest: /path/to/laravel version: main - name: Install Composer dependencies composer: command: install working_dir: /path/to/laravel no_dev: true - name: Run migrations command: php artisan migrate --force args: chdir: /path/to/laravel - name: Cache configuration command: php artisan config:cache args: chdir: /path/to/laravel - name: Cache routes command: php artisan route:cache args: chdir: /path/to/laravel - name: Optimize application command: php artisan optimize args: chdir: /path/to/laravel
This Ansible playbook automates the process of cloning the Git repository, installing Composer dependencies, running migrations, caching configuration and routes, and optimizing the Laravel application.
Environment-Based Configuration
Laravel’s environment-based configuration is crucial for maintaining separation between development, staging, and production environments. By utilizing environment variables, you can easily configure your application based on the environment it’s deployed to.
In Laravel, you can define environment-specific configuration variables in the .env file. Here’s an example of a .env file:
makefile APP_ENV=production APP_DEBUG=false APP_URL=https://example.com DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=myapp DB_USERNAME=myuser DB_PASSWORD=mypassword
By modifying the .env file on each environment, you can specify different database connections, cache drivers, logging settings, and more.
Using environment variables allows for easy deployment across multiple environments without the need to modify the application’s codebase for each environment-specific configuration.
Containerization with Docker
Containerization with Docker provides a lightweight and consistent environment for deploying Laravel applications. Docker allows you to package your application, its dependencies, and configuration into a portable container, ensuring consistent deployment across different environments.
Here’s an example of a Dockerfile for a Laravel application:
Dockerfile FROM php:7.4-fpm WORKDIR /var/www/html COPY . . RUN apt-get update && apt-get install -y \ git \ zip \ unzip \ && docker-php-ext-install pdo_mysql \ && curl -sS https://getcomposer.org/installer | php -- --install-dir=/usr/local/bin --filename=composer RUN composer install --no-dev --optimize-autoloader RUN chown -R www-data:www-data storage bootstrap/cache RUN php artisan config:cache && php artisan route:cache && php artisan optimize CMD php-fpm EXPOSE 9000
In this example, we start from a PHP-FPM base image, copy the Laravel application into the container, install dependencies, cache configuration and routes, and expose the container’s port. This Dockerfile can be used to build a Docker image for the Laravel application.
Containerization simplifies the deployment process by providing a consistent environment and making it easier to scale and manage resources.
Load Balancing and Scaling
As your Laravel application grows, load balancing and scaling become essential to ensure high availability and performance. Load balancing distributes incoming traffic across multiple instances of your application, while scaling allows you to add or remove instances based on demand.
Using tools like Nginx or HAProxy as a load balancer in front of your Laravel application servers can significantly improve performance and handle increased traffic.
Here’s an example Nginx configuration for load balancing multiple Laravel application servers:
nginx upstream laravel { server app-server1:9000; server app-server2:9000; } server { listen 80; server_name example.com; location / { proxy_pass http://laravel; proxy_set_header Host $host; proxy_set_header X-Real-IP $remote_addr; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; proxy_set_header X-Forwarded-Proto $scheme; } }
In this example, the Nginx server listens on port 80 and proxies requests to the Laravel application servers defined in the upstream block. Load balancing distributes the requests across the specified servers.
Zero-Downtime Deployments
Zero-downtime deployments are crucial to ensure uninterrupted service during the deployment process. By utilizing techniques such as rolling deployments, blue-green deployments, or canary deployments, you can minimize or eliminate downtime during the deployment phase.
- Rolling Deployments
Rolling deployments involve gradually updating the instances of your application one by one, ensuring that there’s always a healthy instance serving requests. Here’s an example deployment script for a rolling deployment:
bash # Assuming you have SSH access to the server ssh user@server1 'cd /path/to/laravel && git pull origin main' ssh user@server1 'cd /path/to/laravel && composer install --no-dev --optimize-autoloader' ssh user@server1 'cd /path/to/laravel && php artisan migrate --force' ssh user@server1 'cd /path/to/laravel && php artisan config:cache' ssh user@server1 'cd /path/to/laravel && php artisan route:cache' ssh user@server1 'cd /path/to/laravel && php artisan optimize' ssh user@server2 'cd /path/to/laravel && git pull origin main' ssh user@server2 'cd /path/to/laravel && composer install --no-dev --optimize-autoloader' ssh user@server2 'cd /path/to/laravel && php artisan migrate --force' ssh user@server2 'cd /path/to/laravel && php artisan config:cache' ssh user@server2 'cd /path/to/laravel && php artisan route:cache' ssh user@server2 'cd /path/to/laravel && php artisan optimize'
In this example, the deployment script updates one server at a time, ensuring that there’s always a functioning instance available to handle requests.
- Blue-Green Deployments
Blue-green deployments involve maintaining two identical environments, one serving as the production environment (blue) and the other as the staging environment (green). When a new version of the application is ready for deployment, it is deployed to the green environment. After thorough testing and validation, traffic is routed from the blue environment to the green environment, making it the new production environment.
Here’s an example deployment process for blue-green deployments:
- Set up the blue environment as the production environment and ensure it’s fully functional.
- Create an identical green environment and deploy the new version of the application to it.
- Perform thorough testing and validation on the green environment to ensure everything works as expected.
- Route traffic to the green environment, making it the new production environment.
- Optionally, keep the blue environment running for a while to ensure a smooth transition and the ability to roll back if necessary.
Blue-green deployments offer the advantage of minimizing downtime and providing a controlled environment for testing and validation before releasing the new version to end-users
- Canary Deployments
Canary deployments involve gradually rolling out a new version of the application to a subset of users or servers, allowing for testing and monitoring before fully deploying it to the entire user base or server fleet. This strategy helps identify potential issues or performance bottlenecks in the new version while minimizing the impact on users.
Here’s an example process for implementing canary deployments:
- Deploy the new version of the application to a small percentage of users or a specific set of servers.
- Monitor the performance, errors, and user feedback on the canary group.
- If the new version performs well and shows no major issues, gradually increase the percentage of users or servers on which it is deployed.
- Continuously monitor and analyze the application’s performance and user feedback during the canary deployment phase.
- If any issues arise, roll back the deployment or make necessary adjustments before proceeding with a full rollout.
Canary deployments provide a controlled and monitored approach to deploying new versions, allowing for early detection and mitigation of issues before affecting the entire user base.
Monitoring and Error Handling
Monitoring your Laravel application in production is crucial to ensure its health, performance, and availability. Effective monitoring allows you to identify and resolve issues proactively, ensuring a seamless user experience. Here are some essential monitoring and error handling strategies:
- Implement a centralized logging system to collect and analyze logs from your application and infrastructure.
- Utilize performance monitoring tools like New Relic, Datadog, or Prometheus to track key metrics, such as response times, CPU usage, memory consumption, and database performance.
- Set up alerts and notifications to be informed of critical issues or performance degradation.
- Implement error tracking and reporting using tools like Sentry or Bugsnag to identify and resolve application errors in real-time.
- Monitor server health and resource utilization to identify potential bottlenecks or performance issues.
- Regularly review and analyze application and server logs to gain insights into user behavior, error patterns, and performance bottlenecks.
By implementing robust monitoring and error handling practices, you can quickly identify and address issues, ensuring a reliable and high-performing Laravel application in production.
Conclusion
In this comprehensive guide, we’ve explored various deployment strategies for Laravel applications, from Continuous Integration and Continuous Deployment (CI/CD) to Git-based deployments, server configuration management, environment-based configuration, containerization with Docker, load balancing and scaling, zero-downtime deployments, blue-green deployments, canary deployments, and monitoring/error handling.
By implementing these strategies, you can ensure a smooth and efficient transition from development to production, with minimal downtime and maximum reliability. Choose the strategies that best fit your application’s requirements and leverage the power of Laravel to deliver robust and scalable applications to your end-users. Remember to continually evaluate and improve your deployment process to adapt to changing needs and technologies. Happy deploying!
Table of Contents
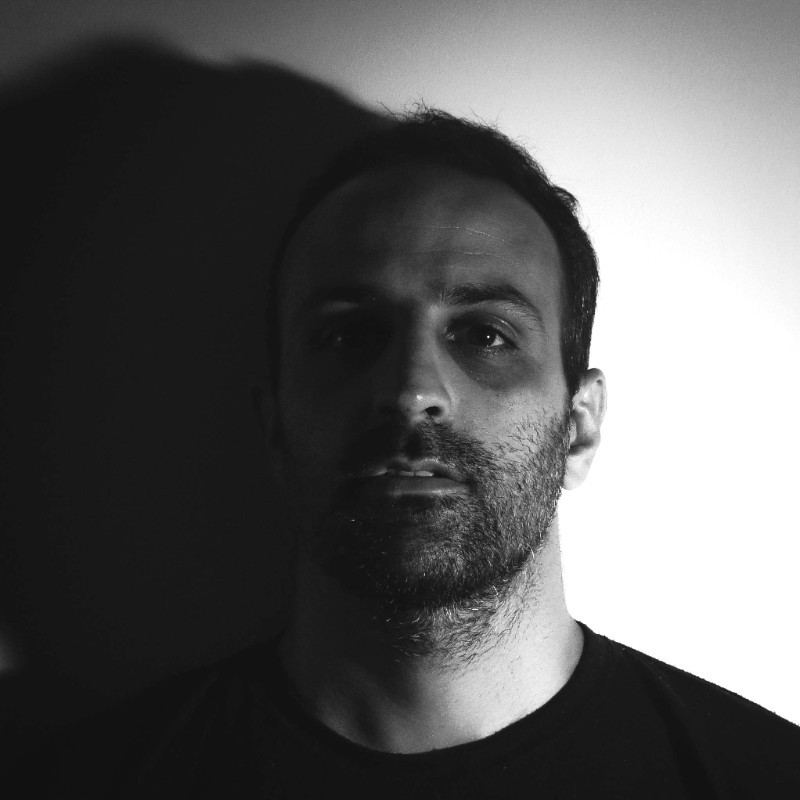
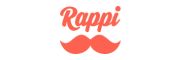