How Laravel Dusk Revolutionizes Browser Testing: Tips, Tricks & Examples
Laravel, an open-source PHP framework, has always prioritized developer convenience. While the framework takes care of backend functionalities with ease, it also extends its support to frontend testing with Laravel Dusk. Laravel Dusk is a browser testing tool tailored for the Laravel ecosystem, providing an expressive and easy-to-use API for browser automation and testing.
1. Why Use Laravel Dusk?
- Real Browser Testing: Unlike some other PHP testing tools, Dusk doesn’t simulate a browser’s behavior. Instead, it uses a real browser, giving developers a precise picture of user interactions.
- Expressive API: Dusk’s API is both simple and expressive, making it easy to write browser tests.
- Supports JavaScript: Dusk leverages ChromeDriver, allowing developers to test JavaScript-enhanced applications without breaking a sweat.
2. Setting Up Laravel Dusk
Before diving into examples, let’s understand the setup:
- Install Dusk via Composer:
``` composer require --dev laravel/dusk ```
- Register the service provider:
In `app/Providers/AppServiceProvider.php`, add:
```php use Laravel\Dusk\DuskServiceProvider; public function register() { if ($this->app->environment('local', 'testing')) { $this->app->register(DuskServiceProvider::class); } } ```
- Publish the Dusk assets:
``` php artisan dusk:install ```
This command creates a `Browser` directory under `tests` and sets up `DuskTestCase.php`.
3. Laravel Dusk Testing Examples
3.1. Basic Authentication Test
In real-world scenarios, checking if authentication is working as expected is crucial. Here’s a simple Dusk test to check login functionality:
```php namespace Tests\Browser; use Tests\DuskTestCase; use Laravel\Dusk\Browser; use Illuminate\Foundation\Testing\DatabaseMigrations; class AuthenticationTest extends DuskTestCase { /** * Test user login. * * @return void */ public function testUserLogin() { $this->browse(function (Browser $browser) { $browser->visit('/login') ->type('email', 'user@example.com') ->type('password', 'secret') ->press('Login') ->assertPathIs('/home'); }); } } ```
3.2. Form Submission
Let’s consider a simple contact form with fields `name`, `email`, and `message`:
```php public function testContactForm() { $this->browse(function (Browser $browser) { $browser->visit('/contact') ->type('name', 'John Doe') ->type('email', 'john@example.com') ->type('message', 'This is a test message.') ->press('Submit') ->assertSee('Thank you for contacting us!'); }); } ```
3.3. JavaScript Interactions
If you have a button that reveals a hidden message using JavaScript, you can test it with Dusk:
```php public function testRevealMessage() { $this->browse(function (Browser $browser) { $browser->visit('/page-with-hidden-message') ->press('Reveal Message') ->waitForText('Hidden Message Revealed!') ->assertSee('Hidden Message Revealed!'); }); } ```
3.4. URL Assertions
Verify redirections or URL updates:
```php public function testRedirection() { $this->browse(function (Browser $browser) { $browser->visit('/old-url') ->assertPathIs('/new-url'); }); } ```
Dusk integrates seamlessly with Laravel’s database testing features:
```php use Illuminate\Foundation\Testing\DatabaseMigrations; class DatabaseTest extends DuskTestCase { use DatabaseMigrations; public function testDatabaseInteraction() { $this->browse(function (Browser $browser) { // ... perform some browser interactions ... $this->assertDatabaseHas('users', [ 'email' => 'john@example.com' ]); }); } } ```
4. Running Dusk Tests
To run your Dusk tests, use:
``` php artisan dusk ```
Conclusion
Laravel Dusk offers an intuitive, robust, and developer-friendly environment to test web applications end-to-end. By leveraging real browsers and integrating effortlessly into the Laravel ecosystem, Dusk becomes a game-changer for developers who prioritize quality assurance.
Remember, while Dusk is potent for browser testing, it complements other types of tests like unit and integration tests. Maintain a balanced testing pyramid to ensure your Laravel applications are stable, reliable, and bug-free.
Table of Contents
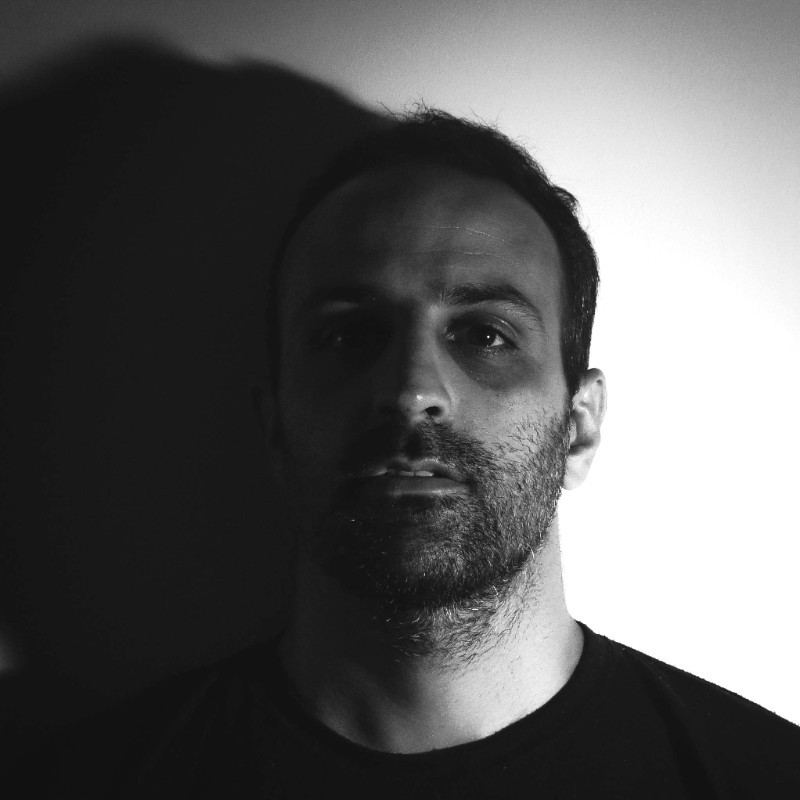
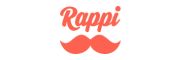