Laravel E-commerce: Building Online Stores with Laravel
In the digital age, establishing an online presence for your business is not just an option – it’s a necessity. With the rapid growth of e-commerce, having a well-functioning online store can significantly boost your sales and brand visibility. If you’re looking to create a dynamic and feature-rich online store, Laravel, the popular PHP framework, can be your ultimate tool. In this guide, we will delve into the world of Laravel e-commerce and learn how to construct powerful online stores from the ground up.
Table of Contents
1. Why Choose Laravel for E-commerce?
- Laravel has gained a reputation as one of the most elegant and developer-friendly PHP frameworks available today. Its intuitive syntax, extensive documentation, and an active community make it an excellent choice for building complex web applications like e-commerce platforms. Here are a few reasons why Laravel stands out for e-commerce development:
- Modular Architecture: Laravel’s modular architecture allows developers to break down complex functionalities into smaller, manageable components. This not only promotes code reusability but also simplifies the development and maintenance of e-commerce platforms.
- Eloquent ORM: Laravel’s Eloquent ORM (Object-Relational Mapping) streamlines database interactions by providing a simple and expressive syntax. This is especially beneficial for e-commerce platforms that involve complex data relationships.
- Robust Routing System: E-commerce platforms often require dynamic routing to handle various pages like product listings, product details, and shopping carts. Laravel’s routing system makes it easy to define custom routes and handle different URL structures.
2. Setting Up Your Laravel E-commerce Project
Let’s get started by setting up a new Laravel project for your e-commerce venture. Ensure you have Composer installed, as it’s a fundamental tool for managing Laravel dependencies.
Step 1: Install Laravel
Open your terminal and execute the following command to create a new Laravel project:
bash composer create-project --prefer-dist laravel/laravel my-ecommerce-store
Step 2: Configure Database
Navigate to the project’s root directory and open the .env file. Configure your database connection settings to match your server credentials:
dotenv DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=my_ecommerce_db DB_USERNAME=my_username DB_PASSWORD=my_password
Step 3: Create Models and Migrations
In Laravel, models represent database tables and their relationships. Migrations are used to define the structure of the database tables. Let’s create a model and migration for our products:
bash php artisan make:model Product -m
This command generates a Product model and its corresponding migration file. Open the migration file (database/migrations/YYYY_MM_DD_create_products_table.php) and define the columns for your products.
Step 4: Run Migrations
Execute the following command to run the migrations and create the products table in your database:
bash php artisan migrate
Your Laravel e-commerce project is now ready to start handling product data!
3. Building Product Management
A crucial aspect of any e-commerce platform is product management. Let’s explore how to create, read, update, and delete products using Laravel’s powerful features.
3.1. Creating Products:
To add a new product to your e-commerce platform, you need to create a controller, views, and routes. Run the following commands to generate the necessary components:
bash php artisan make:controller ProductController php artisan make:views products
In your ProductController, define methods to handle product creation and validation:
php public function create() { return view('products.create'); } public function store(Request $request) { $validatedData = $request->validate([ 'name' => 'required', 'price' => 'required|numeric', 'description' => 'required', ]); Product::create($validatedData); return redirect()->route('products.index') ->with('success', 'Product created successfully'); }
Create the corresponding views for product creation and listing.
3.2. Reading Products:
To display a list of products, modify the index method in your ProductController:
php public function index() { $products = Product::all(); return view('products.index', compact('products')); }
In your products/index.blade.php view, iterate through the products and display their details.
3.3. Updating and Deleting Products:
Implementing the ability to update and delete products follows a similar pattern. In the ProductController, add methods for editing and updating products:
php public function edit(Product $product) { return view('products.edit', compact('product')); } public function update(Request $request, Product $product) { $validatedData = $request->validate([ 'name' => 'required', 'price' => 'required|numeric', 'description' => 'required', ]); $product->update($validatedData); return redirect()->route('products.index') ->with('success', 'Product updated successfully'); } For deleting products, add the following method: php Copy code public function destroy(Product $product) { $product->delete(); return redirect()->route('products.index') ->with('success', 'Product deleted successfully'); }
By incorporating these methods, you’ll empower your e-commerce platform with robust product management capabilities.
4. Implementing Shopping Cart Functionality
No e-commerce platform is complete without a shopping cart feature that allows users to select and manage items before checkout. Let’s see how Laravel makes it easy to implement this functionality.
4.1. Creating the Cart:
Start by creating a new controller for managing the shopping cart:
bash php artisan make:controller CartController
In your CartController, define methods to add and remove items from the cart:
php public function add(Product $product) { Cart::add($product->id, $product->name, 1, $product->price); return redirect()->back()->with('success', 'Product added to cart'); } public function remove(Product $product) { Cart::remove($product->id); return redirect()->back()->with('success', 'Product removed from cart'); }
4.2. Displaying the Cart:
Create a view to display the contents of the cart. In your cart.blade.php view, iterate through the cart items and calculate the total price:
php @foreach(Cart::content() as $item) <div> <h4>{{ $item->name }}</h4> <p>Price: ${{ $item->price }}</p> <a href="{{ route('cart.remove', $item->model) }}">Remove</a> </div> @endforeach <div> <p>Total: ${{ Cart::total() }}</p> </div>
Remember to define the necessary routes for adding and removing items from the cart.
5. Securing Your Laravel E-commerce Platform
Security is paramount when it comes to e-commerce platforms, as they handle sensitive customer information and financial transactions. Laravel provides several security features to help you safeguard your platform.
5.1. Authentication and Authorization:
Laravel’s built-in authentication scaffolding allows you to quickly implement user registration, login, and password reset functionalities. Additionally, you can use Laravel’s authorization features to control user access to specific parts of your e-commerce platform.
5.2. Validation:
Laravel’s validation rules ensure that the data entered by users is in the correct format and meets your requirements. Implement validation for forms that involve user input, such as registration, login, and checkout.
5.3. CSRF Protection:
Laravel automatically generates a CSRF token for each active user session. This token is used to protect against cross-site request forgery attacks, ensuring that requests to your platform are legitimate.
5.4. Secure Payment Gateways:
When integrating payment gateways, ensure that you choose trusted and secure options. Laravel’s robust architecture makes it relatively straightforward to integrate popular payment gateways while adhering to security best practices.
Conclusion
Building an e-commerce platform with Laravel opens up a world of possibilities. From product management to shopping cart functionality and security measures, Laravel’s elegant syntax and comprehensive features make the development process efficient and enjoyable. Whether you’re a seasoned Laravel developer or new to the framework, creating your online store using Laravel can be a rewarding experience. So, take the leap, dive into the world of Laravel e-commerce, and watch your online store flourish in the digital marketplace.
Table of Contents
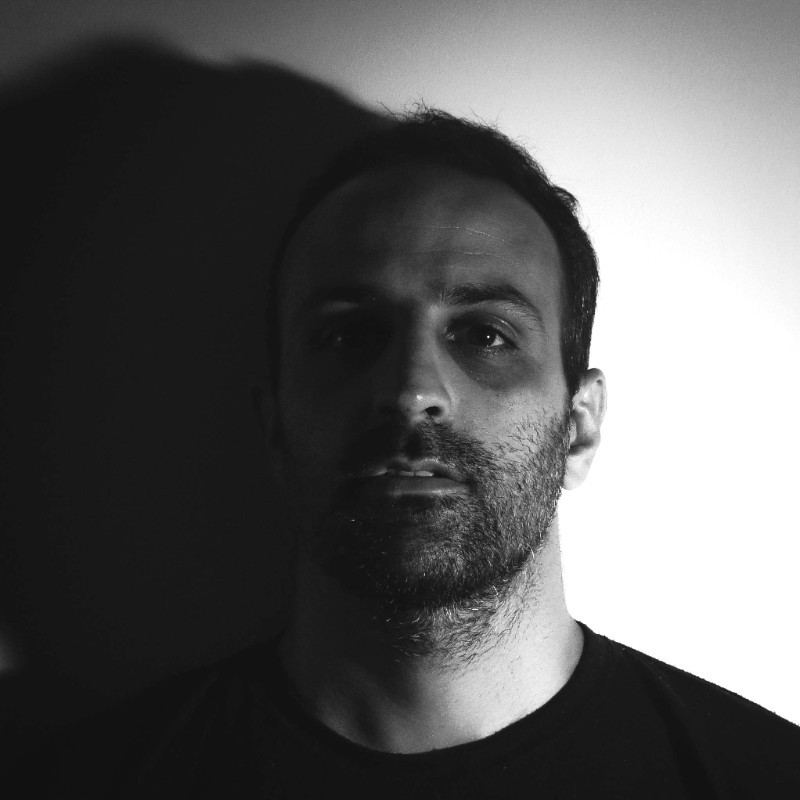
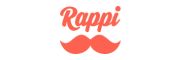