End-to-End Browser Testing Made Easy with Laravel Dusk
In the ever-evolving landscape of web development, testing is key. It’s crucial to hire Laravel developers to ensure that the app you’re building performs as expected, delivering an exceptional user experience. Laravel, a popular PHP framework, makes testing a breeze with Laravel Dusk, a browser automation and testing API. With a team of skilled Laravel developers, you can efficiently navigate through this process. In this blog post, we’ll dig into Laravel Dusk, illustrating how it’s used for end-to-end browser testing with practical examples.
Table of Contents
1. What is Laravel Dusk?
Laravel Dusk is a powerful tool developed by the creators of Laravel for browser-based testing of web applications. It provides an expressive and easy-to-use API for interacting with your application in a real-world, user-focused manner. Dusk can be used for end-to-end testing, making assertions about pages, clicking links, filling out forms, and even testing JavaScript-driven behaviors.
2. Setting Up Laravel Dusk
Before we start exploring Laravel Dusk, we need to install and configure it. First, you should have a Laravel application ready. You can install Dusk using composer:
```bash composer require --dev laravel/dusk ```
After the package has been installed, you should register the `Laravel\Dusk\DuskServiceProvider` service provider. You can do this in the `register` method of your `AppServiceProvider`:
```php use Laravel\Dusk\DuskServiceProvider; public function register() { if ($this->app->environment('local', 'testing')) { $this->app->register(DuskServiceProvider::class); } } ```
Then, run the `dusk:install` Artisan command:
```bash php artisan dusk:install ```
Now that we’ve successfully installed Laravel Dusk, we can start creating tests!
3. Writing Your First Laravel Dusk Test
Laravel Dusk tests are typically stored in the `tests/Browser` directory. To generate a new test, you can use the `dusk:make` command. For instance, if we want to generate a test for a user login page, we can use the command:
```bash php artisan dusk:make LoginTest ```
This will create a new test file in the `tests/Browser` directory. We can now start writing our test.
```php <?php namespace Tests\Browser; use Laravel\Dusk\Browser; use Tests\DuskTestCase; class LoginTest extends DuskTestCase { /** * A basic browser test example. * * @return void */ public function testBasicExample() { $this->browse(function (Browser $browser) { $browser->visit('/login') ->type('email', 'user@domain.com') ->type('password', 'password') ->press('Login') ->assertPathIs('/dashboard'); }); } } ```
In the test above, we are directing the browser to visit the `/login` route of our application, type into the email and password fields, and press the login button. If the login is successful, we expect to be redirected to the `/dashboard` route.
4. Advanced Usage: Working with JavaScript and AJAX
Dusk shines when dealing with JavaScript and AJAX-heavy applications. Since it runs tests in a real browser, it can interact with and test JavaScript behaviors.
Let’s consider a simple example where a user can add items to a shopping cart, and the total price is updated asynchronously:
```php public function testAddToCart() { $this->browse(function (Browser $browser) { $browser->visit('/shop') ->click('@add-to-cart-button') ->waitForText('$49.99', 5); }); } ```
In this test, we visit the shop page, click the ‘add to cart’ button, and then wait for the total price to update to ‘$49.99’. The `waitForText` function will wait for up to 5 seconds for the text to appear.
5. Page Objects and Components
Laravel Dusk offers an elegant way to abstract repetitive actions using Page objects and Components.
5.1. Page Objects
Page objects allow you to define a page’s behaviors in a single location, enhancing readability and maintainability. For instance, consider a `Login` page:
```php class LoginPage extends Page { public function url() { return '/login'; } public function logIn(Browser $browser, $email, $password) { return $browser->type('@email', $email) ->type('@password', $password) ->press('@login'); } } ```
We can then use the `LoginPage` in our tests like so:
```php public function testLogin() { $this->browse(function (Browser $browser) { $loginPage = new LoginPage; $browser->visit($loginPage) ->on($loginPage) ->logIn('user@domain.com', 'password') ->assertPathIs('/dashboard'); }); } ```
5.2. Components
Components allow you to encapsulate parts of a page that are used across multiple pages. Let’s say you have a common datepicker component:
```php class Datepicker extends Component { public function selector() { return '.datepicker'; } public function setDate(Browser $browser, $date) { $browser->type('@datepicker-input', $date); } } ```
You can use this component in your tests:
```php public function testDatepicker() { $this->browse(function (Browser $browser) { $datepicker = new Datepicker; $browser->visit('/events') ->within($datepicker, function ($browser) { $browser->setDate('2023-08-02'); }) ->press('@submit') ->assertSee('Event on 2023-08-02'); }); } ```
Conclusion
Laravel Dusk is a powerful tool, developed by expert Laravel developers, that provides an elegant and easy-to-use API for browser testing. It stands out remarkably for end-to-end testing and performs exceptionally well with JavaScript and AJAX.
Keep in mind, an application that is well-tested is an application that can evolve without fear of breaking existing features. This is where hiring skilled Laravel developers can truly make a difference. With their proficiency in Laravel Dusk, they ensure your application’s integrity, providing you with peace of mind and assurance that your application will behave as expected, no matter how intricate it becomes.
Table of Contents
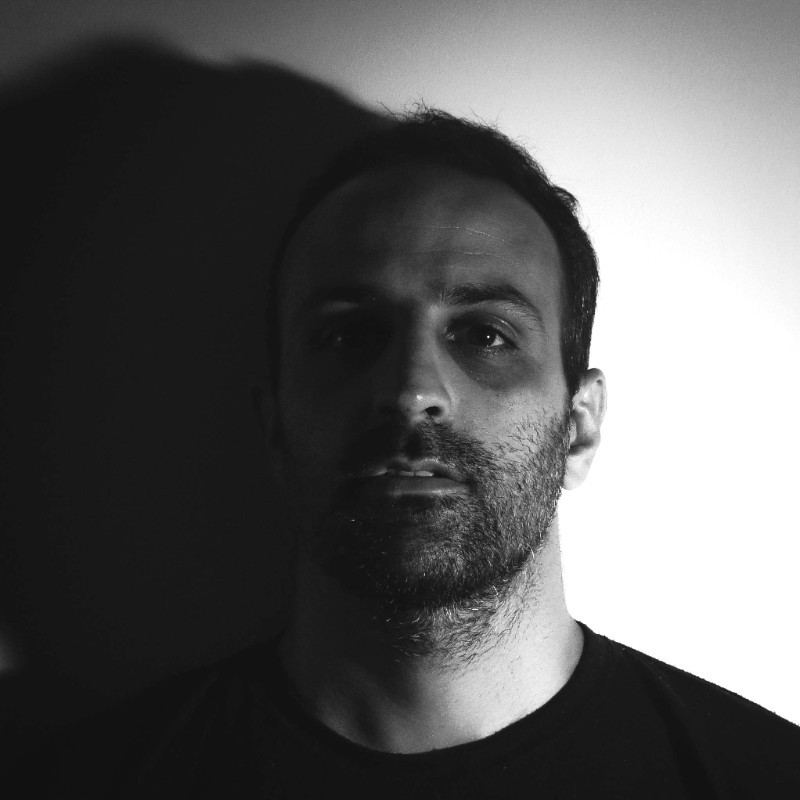
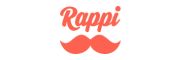