How to manage environment variables in Laravel?
Managing environment variables in Laravel is like organizing your application’s secrets and configurations in a secure and manageable way—it ensures that sensitive information remains protected while allowing for flexibility and customization. Here’s how you can manage environment variables in Laravel in a user-friendly manner:
Understanding Environment Variables: Environment variables are dynamic values that can affect the behavior of your application. They are used to store configuration settings, API keys, database credentials, and other sensitive information that your application needs to function properly.
Using the .env File: Laravel uses a .env file located in the root directory of your project to manage environment variables. This file is not included in version control and is specific to each environment (e.g., development, staging, production). It follows a simple key-value pair format, where each line represents a variable assignment:
makefile APP_NAME=Laravel APP_ENV=local APP_KEY=base64:your_app_key_here ...
Accessing Environment Variables: In your Laravel application, you can access environment variables using the env() helper function. This function retrieves the value of the specified environment variable from the .env file:
php $appName = env('APP_NAME');
Setting Default Values: You can provide default values for environment variables in case they are not defined in the .env file. This ensures that your application continues to function even if certain variables are missing:
php $appName = env('APP_NAME', 'Laravel');
Sensitive Information: Avoid storing sensitive information directly in the .env file, especially if you’re sharing your codebase with others or using version control systems like Git. Instead, use placeholders or reference external secrets management services for sensitive data like passwords or API keys.
Environment-specific Configurations: You can create multiple .env files for different environments (e.g., .env.development, .env.staging, .env.production) and specify environment-specific configurations and variables in each file.
Caching Environment Variables: In production environments, it’s a good practice to cache environment variables to improve performance and reduce file system operations. You can use the config:cache Artisan command to cache configuration files, including environment variables:
arduino php artisan config:cache
By following these best practices, you can effectively manage environment variables in your Laravel application, ensuring security, flexibility, and ease of configuration across different environments.
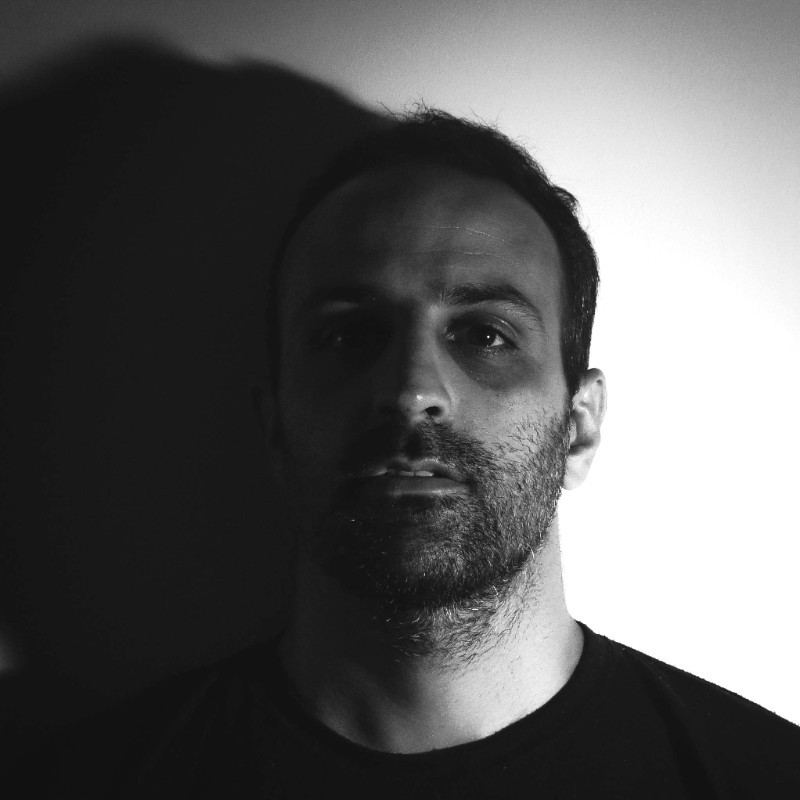
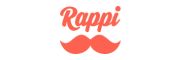