Laravel Form Validation: Ensuring Data Integrity and Security
In today’s digital world, data integrity and security are of paramount importance. When dealing with user input, it is crucial to validate the data to ensure its integrity and protect against malicious attacks. Laravel, a popular PHP framework, provides a powerful and intuitive form validation system that allows developers to validate user input effortlessly. In this blog post, we will explore Laravel’s form validation capabilities and learn how to implement them effectively to safeguard our applications.
What is Form Validation?
Form validation is the process of verifying the correctness and validity of user input in web forms. It involves checking whether the data provided by the user adheres to specific rules and constraints defined by the application. The primary goals of form validation are to ensure data integrity, enhance user experience, and prevent security vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks.
Laravel Form Validation Basics
Laravel provides a comprehensive form validation system out of the box. The framework offers a fluent interface for defining validation rules and automatically handles error messages. Let’s dive into some code examples to understand how Laravel’s form validation works.
Example 1: Basic Validation Rule
php public function store(Request $request) { $validatedData = $request->validate([ 'name' => 'required', 'email' => 'required|email', 'password' => 'required|min:8', ]); // Process validated data }
In the above example, we define validation rules for three form fields: ‘name’, ’email’, and ‘password’. The ‘required’ rule ensures that the fields are not empty, while ’email’ and ‘min’ rules enforce email format and minimum length constraints, respectively. If any of the validation rules fail, Laravel will automatically redirect the user back to the form with the appropriate error messages.
Example 2: Custom Error Messages
php public function store(Request $request) { $messages = [ 'name.required' => 'The name field is required.', 'email.required' => 'The email field is required.', 'email.email' => 'The email must be a valid email address.', 'password.required' => 'The password field is required.', 'password.min' => 'The password must be at least 8 characters.', ]; $validatedData = $request->validate([ 'name' => 'required', 'email' => 'required|email', 'password' => 'required|min:8', ], $messages); // Process validated data }
In this example, we provide custom error messages for each validation rule by specifying an associative array $messages. Laravel will use these messages when displaying validation errors to the user. Custom error messages enable us to provide more informative and user-friendly feedback.
Common Validation Rules
Laravel offers a wide range of built-in validation rules that cater to various scenarios. Let’s explore some of the most commonly used rules:
- ‘required’: Ensures the field is not empty.
- ’email’: Validates the field value as an email address.
- ‘numeric’: Verifies that the field contains only numeric characters.
- ‘min: value’: Specifies the minimum length or value constraint.
- ‘max: value’: Specifies the maximum length or value constraint.
- ‘unique: table, column’: Validates that the field value is unique in a specified database table column.
- ‘confirmed’: Matches two fields to confirm their values are identical, often used for password confirmation.
These are just a few examples of Laravel’s extensive validation rules. The framework provides many more rules, allowing developers to handle various scenarios effectively.
Custom Validation Rules
In addition to the built-in validation rules, Laravel enables us to define our custom validation rules easily. This feature is particularly useful when dealing with unique business logic or specific data formats. Let’s look at an example:
php public function boot() { Validator::extend('telephone', function ($attribute, $value, $parameters, $validator) { return preg_match('/^(\+\d{1,3}[- ]?)?\d{10}$/', $value); }); }
In this example, we define a custom validation rule named ‘telephone’ using the Validator::extend method. The rule checks whether the given value matches a regular expression pattern for a valid telephone number. Once defined, we can use the ‘telephone’ rule like any other built-in rule in our validation logic.
Form Request Validation
While inline validation within controller methods works well for simple forms, Laravel provides an even more elegant solution called “Form Request Validation.” It allows us to encapsulate the validation logic within dedicated form request classes, resulting in cleaner and more maintainable code. Let’s see how it works:
Step 1: Generate a Form Request Class
To generate a new form request class, we can use the make:request artisan command:
shell php artisan make:request StoreUserRequest
Step 2: Define Validation Rules
Open the generated StoreUserRequest class and define the validation rules within the rules method:
php public function rules() { return [ 'name' => 'required', 'email' => 'required|email|unique:users', 'password' => 'required|min:8', ]; }
Step 3: Use the Form Request Class
Now, we can use the StoreUserRequest class in our controller method:
php public function store(StoreUserRequest $request) { // Validation passed, process the request }
By type-hinting the method parameter with the form request class (StoreUserRequest), Laravel will automatically validate the incoming request before executing the store method. If the validation fails, Laravel will handle the redirect and error messages automatically.
Conclusion
Data integrity and security are crucial aspects of web development, and Laravel provides an excellent form validation system to ensure both. By leveraging Laravel’s intuitive validation features, developers can easily validate user input, enforce business rules, and protect their applications against security vulnerabilities. In this blog post, we explored the basics of Laravel form validation, including built-in and custom validation rules, error messages, and the powerful “Form Request Validation” technique. By implementing these best practices, you can enhance the integrity and security of your Laravel applications, providing a robust user experience for your users.
Table of Contents
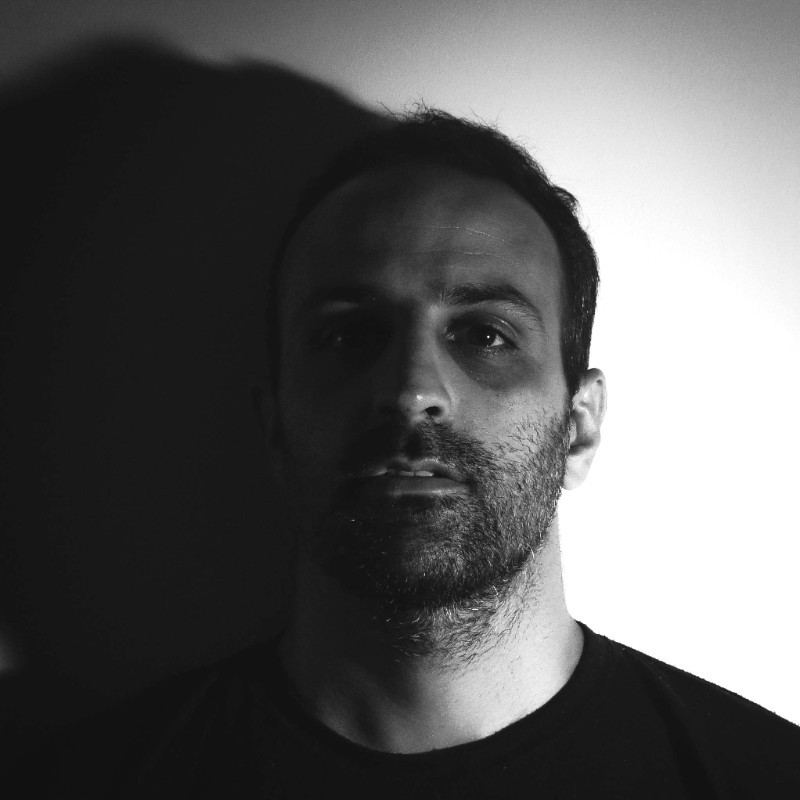
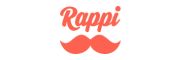