Scaling Laravel Applications: Strategies for Handling High Traffic
When it comes to building web applications, Laravel has established itself as a popular and powerful PHP framework. Its easy-to-use syntax and rich set of features make it a top choice for businesses looking to hire Laravel developers. From small prototypes to large enterprise applications, Laravel equips developers with the tools to build robust solutions. However, when your Laravel application begins to receive high traffic, performance issues may arise. This is when the expertise of Laravel developers can truly shine.
In this post, we’ll explore several strategies that skilled Laravel developers employ to effectively scale applications to handle high traffic efficiently and effectively.
1. Code Optimization
The first step in scaling your Laravel application should always be code optimization. Improper or inefficient code can create bottlenecks in your application, which are only magnified as traffic increases. When you hire Laravel developers with a strong understanding of the framework’s best practices, they can expertly navigate through your codebase and identify areas of inefficiency. Their expertise can ensure that your Laravel application is primed for scalability right from the start.
1.1. Leverage Eager Loading
One of the main culprits of Laravel performance issues is the N+1 problem, which can be solved by implementing Eager Loading. By predicting the data that will be needed in advance, you can reduce the number of queries to your database.
```php $users = User::with('orders')->get();
1.2. Use Laravel’s Cache
Laravel comes with built-in caching that is easy to use. Caching can significantly reduce the load on your server by temporarily storing frequently requested data. Remember to cache both your views and data queries where appropriate.
```php $users = Cache::remember('users', 3600, function () { return User::all(); });
2. Database Optimization
A significant part of a Laravel application’s speed relates to how swiftly it can retrieve data from the database. Therefore, optimizing your database is a critical aspect of handling high traffic. When you hire Laravel developers, they bring along their deep understanding of database structures and performance tuning. Their expertise can play a pivotal role in streamlining database interactions, making your Laravel application much more efficient and capable of managing high traffic conditions.
2.1. Database Indexing
Database indexing can significantly improve the performance of your database queries. For example, if you often search for users by email, make sure to add an index to the ‘email’ column in your ‘users‘ table.
2.2. Database Pagination
If you display a large amount of data on a single page, consider using Laravel’s built-in pagination functionality. This limits the amount of data retrieved from the database and loaded into memory at any given time.
```php $users = User::paginate(10);
3. Use a Load Balancer
As traffic to your application increases, you may find that a single server is insufficient to handle all the requests. In this case, a load balancer can distribute incoming traffic across multiple servers, reducing the load on any single server.
4. Horizontal and Vertical Scaling
There are two main strategies to scale your server resources – vertical scaling (increasing the capacity of your current server) and horizontal scaling (adding more servers).
4.1. Vertical Scaling
Vertical scaling is the simpler option as it involves increasing your server’s power (RAM, CPU, etc.). However, it has a limit and can be costly in the long run.
4.2. Horizontal Scaling
Horizontal scaling involves adding more servers to your application, which, combined with a load balancer, can significantly improve your application’s ability to handle high traffic. This strategy is more complicated to implement but offers better long-term scalability.
5. Implement a Queue System
Laravel’s queue system allows you to defer the processing of a lengthy task, such as sending an email, until a later time, which can drastically speed up web requests to your application.
```php Mail::queue('email.welcome', $data, function ($message) { // });
6. Use CDN for Static Assets
A Content Delivery Network (CDN) is a system of distributed servers that deliver pages and other web content to a user based on their geographic locations. By using a CDN to serve your application’s static assets
(images, CSS, JavaScript), you can reduce the load on your server and improve site speed.
7. Implement HTTP/2
HTTP/2 is a major revision of the HTTP protocol. One of its many improvements over HTTP/1.1 is the ability to multiplex multiple requests over a single TCP connection, which reduces the amount of data being transferred, thus increasing speed.
8. Use Microservices
Splitting your application into microservices can help manage high traffic. Each microservice can be independently deployed, scaled, and even written in different programming languages.
While managing microservices can be complex, the benefits they bring in terms of scalability and the division of responsibility often outweigh the drawbacks.
9. Use Docker for Environment Isolation
Docker provides a way to run applications securely isolated in a container, packaged with all its dependencies and libraries. With Docker, you can maintain consistency across multiple development and production environments. This makes it easier to identify potential issues and fix them before they impact your application’s ability to handle high traffic.
Scaling with Docker involves creating a Docker image of your application and deploying it on multiple containers. These containers can be run on the same server or distributed across multiple servers, improving resource utilization, and providing a smoother experience for your users.
```bash docker build -t your-application . docker run -d -p 80:80 your-application
10. Implement Rate Limiting
Rate limiting is a technique for limiting network traffic. It sets a limit on how many requests a client can make to the server in a specific time period. Laravel provides a middleware that makes it easy to limit the number of requests that a client, identified by their IP address, can make to a route over a specific time interval.
This can be very useful to protect your application from DDoS attacks, web scrapers or just to keep your traffic at a manageable level.
```php Route::middleware('throttle:60,1')->group(function () { Route::get('/user', function () { // }); });
Conclusion
Scaling a Laravel application to handle high traffic involves several strategies, including code optimization, database optimization, load balancing, server scaling, queuing, CDN, implementing HTTP/2, and even splitting your application into microservices.
While this might seem daunting, remember that not all applications need to implement all strategies. This is where the expertise of Laravel developers can make a difference. If you’re considering hiring Laravel developers, they can assist in identifying the bottlenecks in your application and apply the most effective strategies gradually.
With careful planning, testing, and optimization, Laravel developers can successfully scale your application to handle high traffic, ensuring a smooth user experience, no matter how popular your application becomes. So, hiring Laravel developers can indeed prove to be a strategic move for your business.
Table of Contents
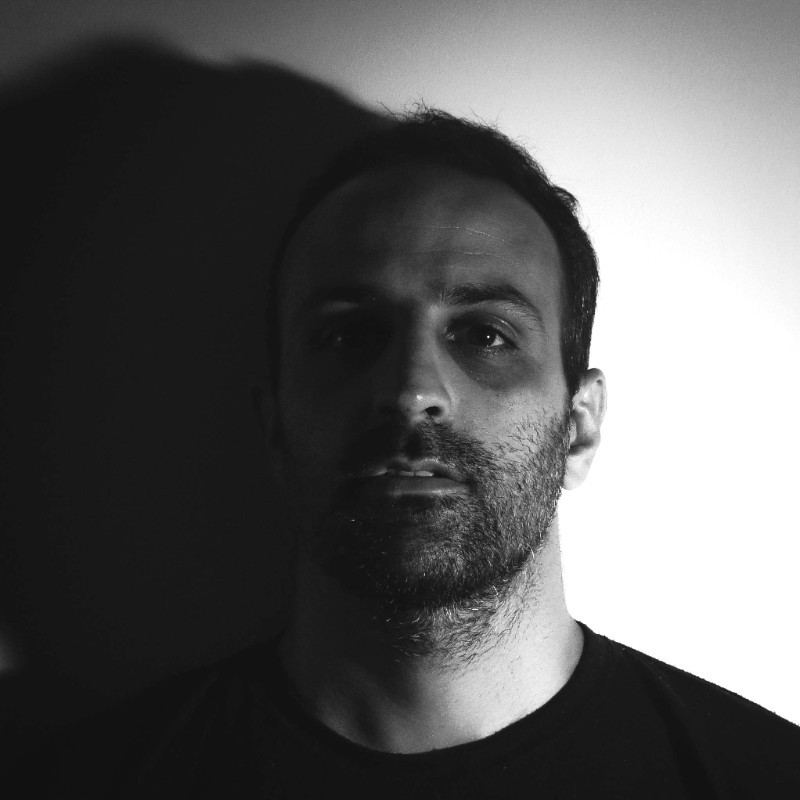
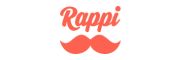