From Installation to Insights: A Comprehensive Guide to Laravel Horizon
Laravel is renowned for providing developers with tools that simplify complex processes. Among these tools is Laravel Horizon, a beautiful dashboard and code-driven configuration system for Laravel Redis queues. Horizon provides a seamless interface to manage and monitor queues, ensuring jobs are executed and tracked efficiently.
In this post, we’ll explore Laravel Horizon, its features, and how to use it to manage and monitor queues effectively.
1. Introduction to Laravel Queues
Before diving into Horizon, it’s important to have a basic understanding of Laravel queues. Queues allow you to defer the processing of a time-consuming task, such as sending an email, until a later time. This speeds up web requests to your application.
When a task is deferred, it’s placed on a queue, which is processed in the background by a “worker”. Laravel supports multiple queue connections, including Redis, database, Amazon SQS, and Beanstalkd.
2. Why Laravel Horizon?
While Laravel provides basic functionality to manage and monitor queues, Horizon takes this a notch higher by providing:
– A beautiful UI dashboard to monitor job throughput, runtime, and other metrics.
– Code-driven configuration.
– Balance workers for multiple queue configurations.
– Job retries.
– Detailed metrics, including job failures and successes.
3. Installing Laravel Horizon
To install Horizon, you need to have Redis installed and configured with Laravel. Then, pull in the Horizon package via composer:
```bash composer require laravel/horizon ```
After installation, publish its assets:
```bash php artisan horizon:install ```
This will also publish the `horizon.php` configuration file to `config/horizon.php`.
4. Configuring Horizon
The primary configuration for Horizon resides in the `config/horizon.php` file. Here you can define multiple environments with different configurations.
Example:
```php 'environments' => [ 'production' => [ 'supervisor-1' => [ 'connection' => 'redis', 'queue' => ['default'], 'balance' => 'simple', 'processes' => 10, 'tries' => 3, ], ], 'local' => [ 'supervisor-1' => [ 'connection' => 'redis', 'queue' => ['default'], 'balance' => 'simple', 'processes' => 3, 'tries' => 1, ], ], ], ```
Here, different configurations for `production` and `local` environments are set. You can adjust processes, connection types, balancing strategy, etc.
5. Using the Horizon Dashboard
To view the Horizon dashboard, you just need to navigate to `/horizon` on your application. The dashboard provides a real-time insight into queue workloads, recent jobs, failed jobs, and job metrics.
Example:
On the dashboard, you might see:
– Pending Jobs: 50
– Active Jobs: 10
– Completed Jobs: 200
– Failed Jobs: 5
You can click on each metric to get detailed information. For instance, clicking on “Failed Jobs” will show each failed job, the error it threw, and an option to retry the job.
6. Job Prioritization and Balancing
Horizon provides a feature to prioritize jobs and balance workers. In the configuration, you can use the `balance` option to specify the strategy (`simple`, `auto`, or `false`).
Example:
If you want to prioritize an `emails` queue over `listeners`, you can use:
```php 'queue' => ['emails', 'listeners'] ```
Horizon will then always ensure that `emails` queue jobs are processed before the `listeners`.
7. Monitoring Specific Jobs
Horizon allows you to monitor specific jobs for their performance and behavior.
Example:
In the `config/horizon.php`, you can define:
```php 'monitored' => [ App\Jobs\SendReminderEmail::class, App\Jobs\ProcessPodcast::class, ], ```
This will ensure Horizon keeps a closer look at these jobs, and you can easily see their metrics separately on the dashboard.
8. Notifications
Horizon has a built-in notification system to alert you of failed jobs. By default, it uses the `mail` notification channel, but you can configure it to use Slack or SMS.
Example:
To send notifications to a Slack channel:
```php 'notifications' => [ 'mail' => false, 'slack' => [ 'webhook_url' => 'your-slack-webhook-url', ], ], ```
Conclusion
Laravel Horizon is an essential tool for applications using Redis queues. It provides a robust solution to manage and monitor queue workloads, ensuring that your background jobs run smoothly and efficiently. Whether you are looking for a real-time monitoring dashboard or tools to optimize worker balance, Horizon has got you covered. Give it a spin and experience the difference it can make in your Laravel applications.
Table of Contents
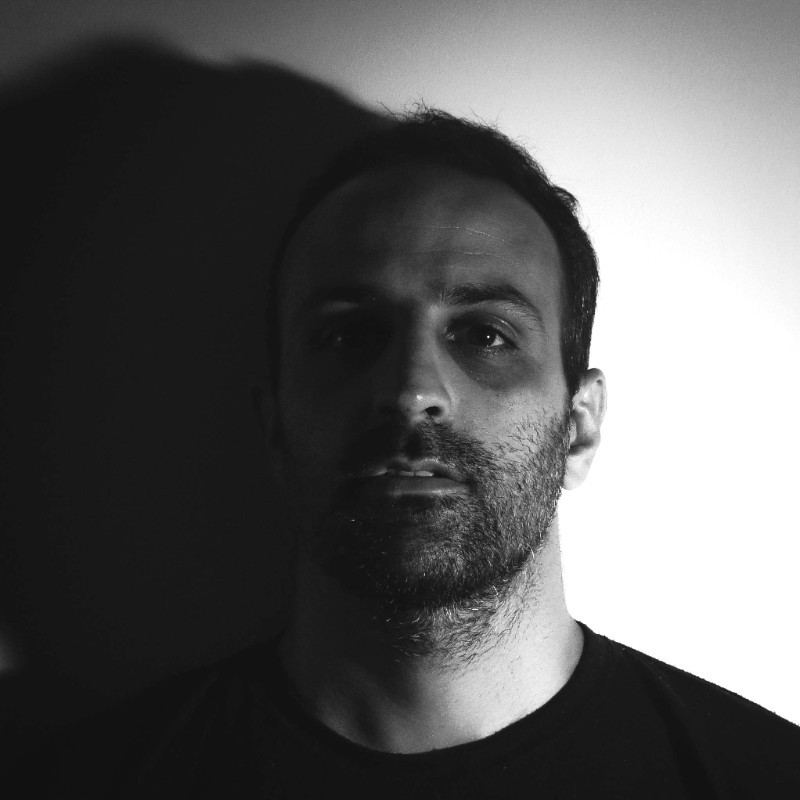
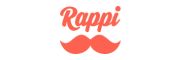