Secure Your Web Resources: Understanding & Implementing Laravel Policies
When building web applications, maintaining a consistent and reliable authorization system is crucial to protect sensitive information. Laravel, a leading PHP framework, makes implementing authorization rules straightforward using its powerful tool called “Policies”.
Table of Contents
Policies are classes that organize authorization logic based on the application’s resources. They’re beneficial when dealing with complex user roles and permissions, providing a clean, efficient, and robust method to control access to resources. However, setting up and managing these policies can be challenging for those unfamiliar with Laravel’s system. This is where the expertise of hired Laravel developers comes into play.
When you hire Laravel developers, you leverage their knowledge and experience to streamline the implementation of Laravel Policies in your applications. Now, let’s dive into how Laravel Policies work and how professional Laravel developers handle them to secure your application’s resources.”
1. Understanding Laravel Policies
In Laravel, Policies are essentially a set of methods where each one corresponds to a specific action that a user can perform on a model/resource (like viewing, creating, updating, or deleting). You can create a separate Policy class for each model you want to apply authorization to.
For example, let’s say you have a `Post` model, and you want to control which users can `view`, `update`, or `delete` a particular post. You would create a `PostPolicy` class, containing methods like `view`, `update`, `delete`, and so on.
2. Setting Up Policies
Before we can use policies, we need to generate them. Laravel provides a handy artisan command to generate a policy.
```bash php artisan make:policy PostPolicy --model=Post ```
This command will create a `PostPolicy` class in the `app/Policies` directory. The `–model` flag indicates the model that this policy corresponds to.
Now, let’s open up the newly created `PostPolicy`. You should see a number of methods corresponding to various actions (view, create, update, delete, etc.). For instance:
```php public function view(User $user, Post $post) { // } public function update(User $user, Post $post) { // } public function delete(User $user, Post $post) { // } ```
These methods are where we’ll implement our authorization rules.
3. Implementing Authorization Rules
Each method in the policy receives at least two arguments; the authenticated `User` instance and the instance of the model the action is being performed on. You should return `true` if the user is authorized to perform the given action and `false` otherwise.
Consider a scenario where we want only the owner of the post to update or delete it. In the `PostPolicy`, the `update` and `delete` methods might look like this:
```php public function update(User $user, Post $post) { return $user->id === $post->user_id; } public function delete(User $user, Post $post) { return $user->id === $post->user_id; } ```
This means only the user who created the post (i.e., their id matches the `user_id` on the `Post` model) can update or delete the post.
4. Using Policies in Controllers
Now that we’ve defined our policy, we can apply it within our controllers using Laravel’s `authorize` method.
Here’s an example of how to use it in the `PostController`:
```php public function update(Request $request, Post $post) { $this->authorize('update', $post); // The current user can update the post... } ```
In the `update` method, before we execute the update logic, we call the `authorize` method passing in the action we want to authorize and the relevant model. If the user is not authorized to perform the action, a `403 HTTP response` is automatically sent.
5. Policy Filters
Sometimes you may wish to authorize all actions for certain users. For example, you might want an administrator to have all privileges. This is where policy filters come in handy.
Within your policy, you can define a `before` method. This method will be run before any other authorization checks:
```php public function before($user, $ability) { if ($user->isAdministrator()) { return true; } } ```
In this example, if the `isAdministrator` method on the User model returns `true`, all authorization checks will be bypassed.
6. Associating Policies with Models
To associate a policy with a model, you should define a `policies` property in your `AuthServiceProvider`. Laravel maps the `Post` model to the `PostPolicy` in the `AuthServiceProvider` like this:
```php protected $policies = [ 'App\Models\Post' => 'App\Policies\PostPolicy', ]; ```
Conclusion
Laravel Policies offer a clean and robust way to handle complex authorization rules in your Laravel applications. By associating actions to your models, you can easily control who can perform what actions on specific resources. With the use of policy filters, you can also quickly bypass these checks for specific users like administrators.
Implementing these policies might seem complicated at first, which is why you might want to consider hiring Laravel developers who are experienced in managing such authorization rules.
Laravel’s strength lies in its simplicity and expressiveness, and Policies are a clear example of that. The next time you’re tasked with managing authorization in a Laravel application, make sure to leverage the power of Policies or hire skilled Laravel developers to do the job!”
Table of Contents
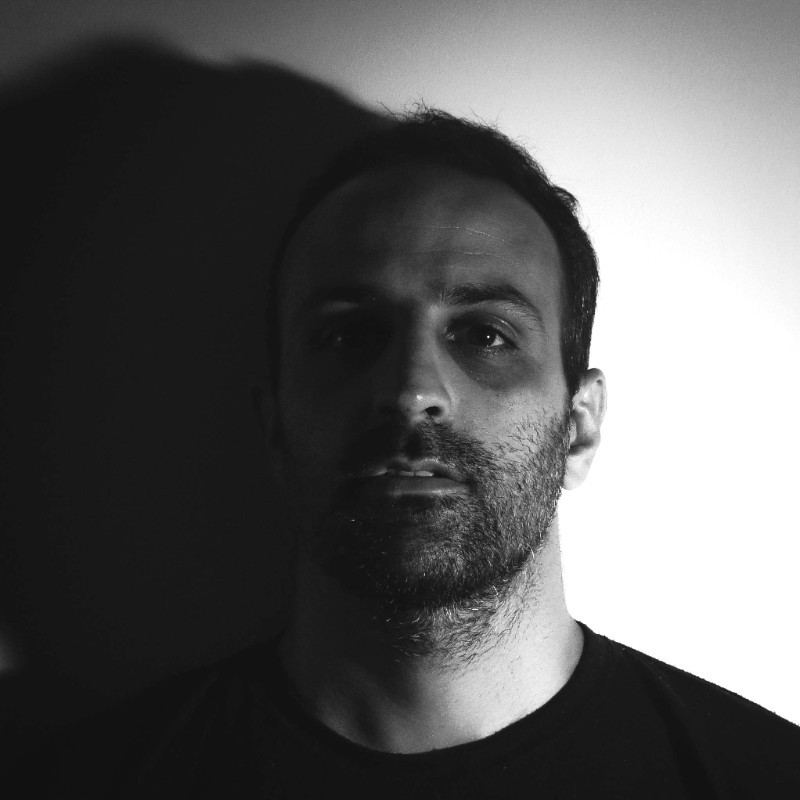
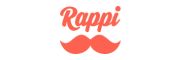