How to install and configure Laravel Passport?
Installing and configuring Laravel Passport is like setting up a secure gateway for your API, allowing users to authenticate and access protected resources with ease—it’s a straightforward process that requires a few simple steps. Let’s walk through how to install and configure Laravel Passport in a human-friendly way:
First, ensure that you have a Laravel application up and running. If you haven’t already created a Laravel project, you can do so using Composer by running the following command in your terminal or command prompt:
lua composer create-project --prefer-dist laravel/laravel your-project-name
Once your Laravel project is set up, navigate to your project directory in the terminal and install Laravel Passport using Composer:
bash composer require laravel/passport
This command will download and install the Laravel Passport package and its dependencies into your Laravel project.
Next, run the Passport installation command to publish the Passport configuration files and migrate the necessary database tables:
php artisan passport:install
This command will generate encryption keys and create the necessary database tables for storing API tokens and client credentials.
After installing Laravel Passport, you’ll need to add the Laravel\Passport\HasApiTokens trait to your User model. This trait provides convenient methods for interacting with API tokens:
php use Laravel\Passport\HasApiTokens; class User extends Authenticatable { use HasApiTokens, Notifiable; // Your model code... }
Next, you’ll need to configure Laravel Passport to use the appropriate driver for storing API tokens. Open your config/auth.php file and set the api driver to passport:
php 'guards' => [ 'api' => [ 'driver' => 'passport', 'provider' => 'users', ], ],
Once you’ve completed these steps, Laravel Passport is installed and configured in your Laravel application. You can now use Passport to issue API tokens, authenticate users, and protect your API routes.
To learn more about how to use Laravel Passport, refer to the official Laravel Passport documentation, which provides detailed instructions and examples for implementing OAuth2 authentication in your Laravel applications. With Laravel Passport, you can build secure and scalable APIs with confidence, knowing that your users’ data is protected and accessible only to authorized parties.
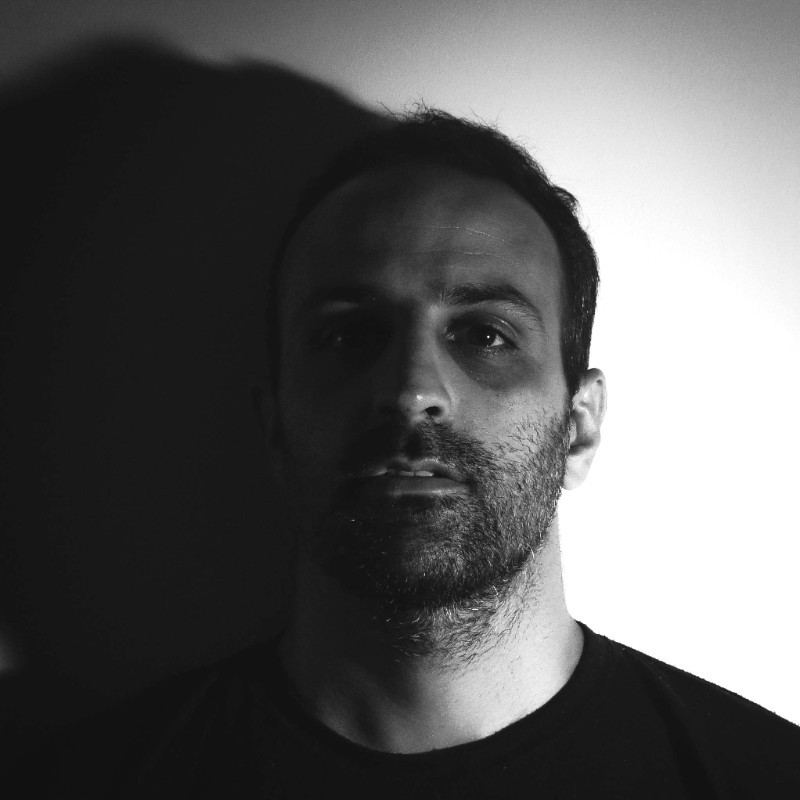
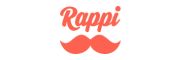