Boost Your Laravel App Performance with Job Dispatching
Laravel, a prominent PHP web application framework, offers developers a wide array of features that can help them build robust, scalable applications. One such feature is Laravel’s job dispatching capability, which not only allows you to manage background tasks with ease, but also underscores why many businesses choose to hire Laravel developers.
Table of Contents
In this blog post, we’ll explore the various ways Laravel’s job dispatching can be utilized, along with examples to illustrate its power and flexibility. If you’re looking to optimize your web applications, you might want to consider the option to hire Laravel developers who can effectively leverage these advanced features.
1. What is Job Dispatching?
Job dispatching in Laravel allows you to defer the processing of time-consuming tasks, such as sending an email, processing uploaded files, or handling complex database queries, to the background. By dispatching these tasks to background processes, the user’s request is handled quickly, ensuring a responsive user experience.
2. Setting Up Job Dispatching
Before we delve into specific examples, let’s set up Laravel’s job dispatching.
First, make sure that your Laravel project is configured to use a queue driver. You can use a variety of drivers like Redis, Database, Beanstalkd, etc. For simplicity, we will use the database driver.
- Configure the Queue Driver: Open your `.env` file and set the queue driver:
```bash QUEUE_CONNECTION=database ```
- Create Migration for Jobs Table: You can create the necessary migration file by running:
```bash php artisan queue:table php artisan migrate ```
- Run Queue Worker: Start the queue worker to process the jobs:
```bash php artisan queue:work ```
With these steps, you are ready to dispatch jobs to the background.
Example 1: Sending Emails in Background
Imagine you have a registration form where users can sign up, and you want to send a welcome email once they register.
- Create a New Job Class: Use the Artisan command to generate a job:
```bash php artisan make:job SendWelcomeEmail ```
- Edit the Job Class: Open the generated file and define the handle method:
```php <?php namespace App\Jobs; use App\Mail\WelcomeEmail; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Foundation\Bus\Dispatchable; use Illuminate\Queue\InteractsWithQueue; use Illuminate\Queue\SerializesModels; use Illuminate\Support\Facades\Mail; class SendWelcomeEmail implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; protected $user; public function __construct($user) { $this->user = $user; } public function handle() { Mail::to($this->user->email)->send(new WelcomeEmail($this->user)); } } ```
- Dispatching the Job: In your registration controller, dispatch the job:
```php use App\Jobs\SendWelcomeEmail; // ... public function register(Request $request) { // Registration logic ... dispatch(new SendWelcomeEmail($user)); return response()->json(['message' => 'User registered successfully!']); } ```
Example 2: Processing Large Files
Let’s say you want to process large CSV files uploaded by users and store the data in the database. This task can take a significant amount of time, making it an ideal candidate for background processing.
- Create a New Job Class: Generate a new job:
```bash php artisan make:job ProcessCsvFile ```
- Edit the Job Class: Edit the job to handle the processing logic:
```php // ... class ProcessCsvFile implements ShouldQueue { // ... public function handle() { // File processing logic, such as reading the CSV file, validating data, and inserting into the database. } } ```
- Dispatching the Job: In your file upload controller, dispatch the job:
```php use App\Jobs\ProcessCsvFile; // ... public function upload(Request $request) { // File upload logic ... dispatch(new ProcessCsvFile($file)); return response()->json(['message' => 'File uploaded successfully! Processing in the background.']); } ```
3. Job Chaining, Delays, and Other Advanced Features
Laravel’s job dispatching offers additional features like job chaining, where you can run multiple jobs sequentially. You can also add delays to jobs, specify the number of attempts, and much more.
Conclusion
Laravel’s job dispatching capability provides a powerful way to handle background tasks, ensuring that the user experience remains smooth and responsive. By leveraging the robust features and simplicity of Laravel’s queues, developers can efficiently manage time-consuming processes, enhancing the application’s overall performance. This is why many businesses choose to hire Laravel developers.
Whether you’re sending emails, processing large files, or managing other complex tasks, Laravel’s job dispatching system is an essential tool in modern web development. Experimenting with it in your next project might save you time and offer a more enjoyable user experience. If you’re looking to supercharge your project’s efficiency and responsiveness, consider the option to hire Laravel developers who can skillfully implement this powerful functionality.
Table of Contents
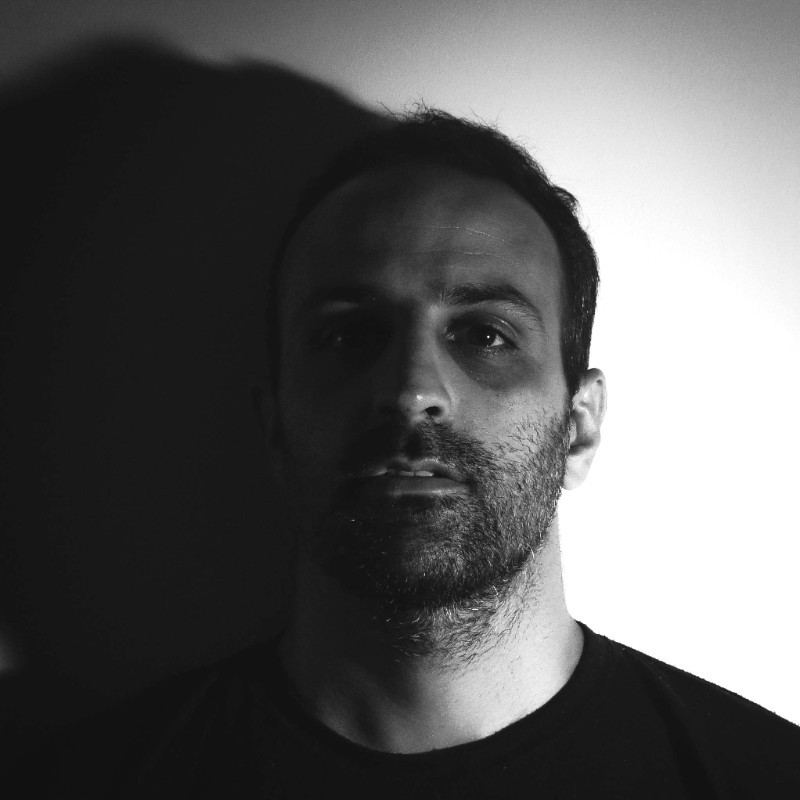
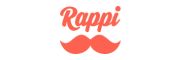