Empower Your Web App: An Introduction to Laravel’s Multi-Language Support
The world is a global village, and businesses are seeking to reach wider audiences across different cultures and languages. Thus, it’s imperative for web developers to create applications that cater to diverse users. Laravel, one of the most popular PHP frameworks, offers built-in support for multi-language applications. This article will explore Laravel’s localization features, showing you how to build multi-language apps.
1. Introduction to Laravel’s Localization
Laravel’s localization features allow you to retrieve language strings based on the user’s locale, enabling you to deliver content tailored to the user’s preferred language. The strings are stored in files within the `resources/lang` directory.
For example, if you are supporting English and Spanish, you would have:
``` /resources /lang /en messages.php /es messages.php ```
Each file returns an array of keyed strings.
2. Setting Up Language Files
Let’s start with a simple example. Inside the `messages.php` for English (`resources/lang/en/messages.php`):
```php <?php return [ 'welcome' => 'Welcome to our application!', ]; ```
For Spanish (`resources/lang/es/messages.php`):
```php <?php return [ 'welcome' => '¡Bienvenido a nuestra aplicación!', ]; ```
3. Retrieving Translation Strings
You can retrieve these translation strings using the `trans` helper function or its shorter version, the `__` function.
```php echo trans('messages.welcome'); // or echo __('messages.welcome'); ```
Based on the user’s set locale, Laravel will automatically fetch the appropriate string.
4. Setting the Locale
The default locale is defined in the `config/app.php` configuration file:
```php 'locale' => 'en', ```
To change the locale at runtime:
```php App::setLocale('es'); ```
You can also get the current locale:
```php $locale = App::getLocale(); ```
5. Handling Pluralization
Laravel’s localization also supports pluralization forms. This is especially handy for languages with complex pluralization rules.
For example, in `messages.php`:
```php <?php return [ 'apples' => 'There is one apple|There are many apples', ]; ```
You can retrieve it with:
```php echo trans_choice('messages.apples', 1); // outputs: There is one apple echo trans_choice('messages.apples', 10); // outputs: There are many apples ```
6. Using JSON Language Files
For applications with heavy frontend JavaScript components, using JSON language files might be preferable. In this case, you don’t need to specify a namespace.
Assuming you have a `resources/lang/en.json`:
```json { "Welcome to our application!": "Welcome to our application!" } ```
You can retrieve it as:
```php echo __('Welcome to our application!'); ```
7. Real-life Example: Building a Multi-Language Website
Let’s assume you’re building a website that supports English and French.
7.1. Set Up Language Files
Create `en` and `fr` directories in `resources/lang` and place a `messages.php` file in each.
7.2. Set Up Routes
```php Route::get('welcome/{locale}', function ($locale) { App::setLocale($locale); return view('welcome'); }); ```
7.3. The Welcome View
In `resources/views/welcome.blade.php`:
```php <!DOCTYPE html> <html> <head> <title>{{ __('messages.title') }}</title> </head> <body> <h1>{{ __('messages.welcome') }}</h1> </body> </html> ```
7.4. Translate
In `resources/lang/en/messages.php`:
```php <?php return [ 'title' => 'Welcome Page', 'welcome' => 'Hello, user!', ]; ```
And in `resources/lang/fr/messages.php`:
```php <?php return [ 'title' => 'Page d'accueil', 'welcome' => 'Bonjour, utilisateur!', ]; ```
Now, visiting `/welcome/en` will display the English version, while `/welcome/fr` will show the French version.
Conclusion
Building multi-language applications in Laravel is a breeze thanks to its powerful localization features. By leveraging the built-in tools, developers can ensure that their applications are globally accessible and user-friendly.
Whether it’s a simple website or a complex application with numerous translations, Laravel’s localization tools make it easy to cater to a global audience. Happy coding!
Table of Contents
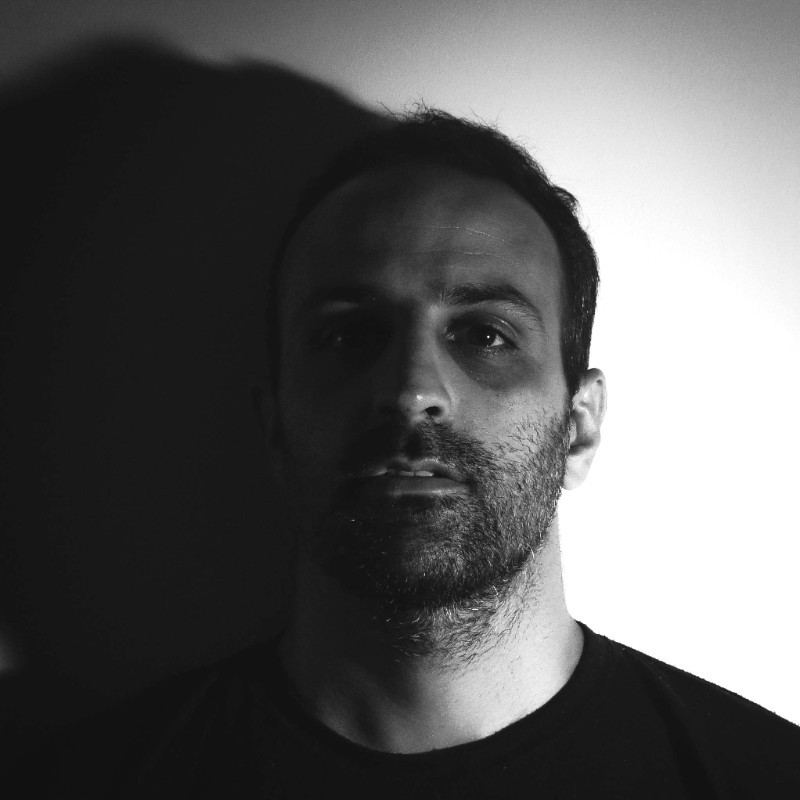
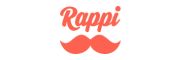