Customizing Laravel: Adding Your Own Logic to HTTP Requests with Middleware
Middleware in Laravel is a powerful feature that allows you to wrap HTTP requests with additional custom logic. They act like protective layers around your application, adding a series of filters or checks that a request must pass before it reaches the core application logic. This is why many choose to hire Laravel developers, for their adeptness in implementing such robust systems. Middleware can serve a variety of purposes, from authenticating users to sanitizing input data or managing cross-origin resource sharing (CORS) settings. If you’re looking to hire Laravel developers, you’ll want them to be knowledgeable in these aspects. In this blog post, we’ll delve into how to create and utilize custom middleware in Laravel, demonstrating the skills you would expect when you hire Laravel developers.
What is Laravel Middleware?
Before diving into examples, let’s first understand what Middleware is. Middleware is a bridge that connects the request and response. It checks the incoming HTTP request and decides whether the request should be passed further into the application or returned early. Middleware in Laravel serves various purposes, like performing authentication checks, authorizing actions, sanitizing input, caching responses, and more. It gives developers an elegant way to add custom logic to the HTTP request-response cycle without cluttering the core application code.
An Example of Laravel’s Built-in Middleware
Laravel comes with several built-in middleware, and you’ve likely already used some of them. Let’s take a look at one of Laravel’s built-in middleware for an example: the authentication middleware, which is named `auth`.
Consider the following piece of code in your route file (`routes/web.php`):
```php Route::get('/dashboard', function () { return view('dashboard'); })->middleware('auth'); ```
In the code snippet above, the `auth` middleware is applied to the `/dashboard` route. This means that if a user tries to access the `/dashboard` URL without being authenticated, they’ll be redirected to the login page. The middleware ensures that only authenticated users can access the dashboard page, adding a layer of security to our application.
Creating Custom Middleware
Creating your own middleware in Laravel is a straightforward process. For our first custom middleware, let’s create a middleware that restricts access based on the user’s age.
You can generate a new middleware using the `make:middleware` Artisan command. Open your terminal, navigate to your Laravel project’s root, and run:
```bash php artisan make:middleware CheckAge ```
This command will generate a new middleware class in the `app/Http/Middleware` directory. Open the `CheckAge.php` file:
```php namespace App\Http\Middleware; use Closure; use Illuminate\Http\Request; class CheckAge { public function handle(Request $request, Closure $next) { // Custom logic goes here... return $next($request); } } ```
The `handle` method is where our custom logic will live. Let’s modify this function to check for the user’s age:
```php public function handle(Request $request, Closure $next) { if ($request->age < 18) { return redirect('home'); } return $next($request); } ```
In this case, if the age provided in the request is less than 18, the middleware will redirect the user to the home page. Otherwise, it will continue processing the request.
Registering Middleware
Once the middleware is created, it must be registered before use. Middleware can be registered globally, or it can be assigned to specific routes or groups of routes.
Globally Middleware
Global middleware runs on every HTTP request to your application. To register a middleware globally, add it to the `$middleware` array in your `app/Http/Kernel.php` file:
```php protected $middleware = [ // Other middleware... \App\Http\Middleware\CheckAge::class, ]; ```
Route Middleware
If you don’t want the middleware to run on every request, you can register it as route middleware. This way, you can assign it only to the routes that need it. Add the middleware to the `$routeMiddleware` array in the `Kernel.php` file:
```php protected $routeMiddleware = [ // Other middleware... 'age' => \App\Http\Middleware\CheckAge::class, ]; ```
Now you can use this middleware in your routes:
```php Route::get('/event', function () { // Event details })->middleware('age'); ```
Middleware Parameters
Middleware can also accept parameters, which can add more flexibility to the logic you implement.
Let’s adjust our `CheckAge` middleware to accept an age parameter. This way, we can use the same middleware to restrict access for different age groups:
```php public function handle(Request $request, Closure $next, $age) { if ($request->age < $age) { return redirect('home'); } return $next($request); } ```
Now you can pass the minimum age as a parameter when you assign the middleware to a route:
```php Route::get('/event', function () { // Event details })->middleware('age:21'); ```
This route now requires users to be 21 or older.
Conclusion
As you can see, middleware in Laravel provides a powerful and elegant way to add custom logic to the HTTP request-response cycle. They are essential to keeping your codebase clean and organized, allowing you to separate your business logic from your request and response handling. Whether you’re a team of Laravel developers or hiring Laravel developers, you will find immense value in mastering middleware. It simplifies tasks such as authenticating users, sanitizing input data, managing CORS settings, and even age-specific checks as in our examples. Laravel’s middleware functionality undoubtedly has you covered.
Table of Contents
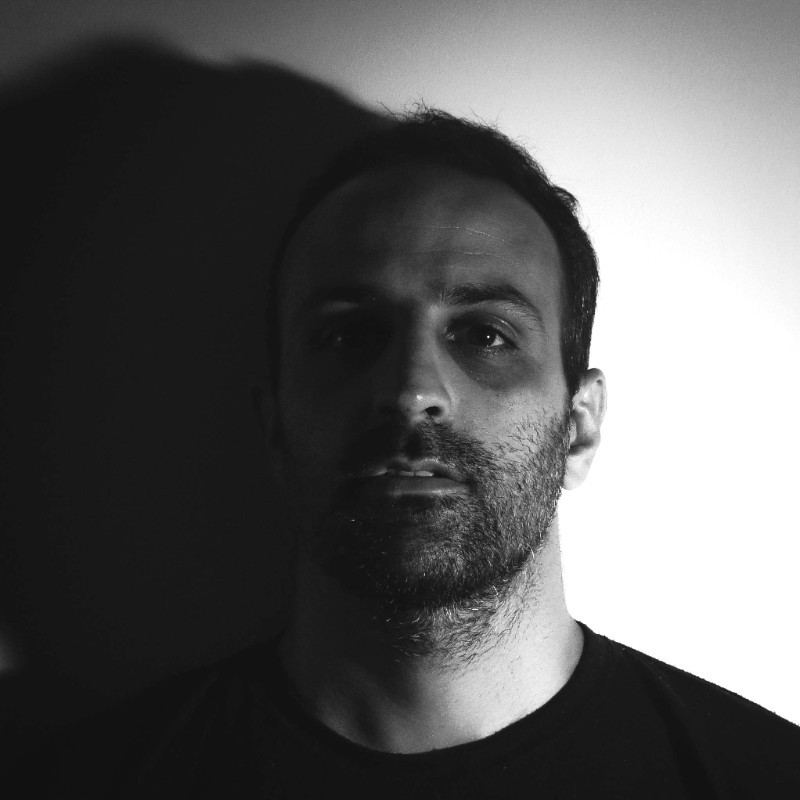
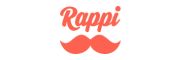