Revolutionize Your Laravel Workflow with Macro Extensions
Laravel, the popular PHP framework, has always been at the forefront when it comes to providing developers with the tools to write clean, maintainable, and expressive code. Among its treasure trove of features, Laravel’s Macros stand out as an elegant way to extend the core functionality of the framework. In essence, Macros allow developers to add custom methods to certain Laravel objects, making the system more malleable and tailored to specific application needs.
In this blog post, we will delve deep into how to use Macros in Laravel and showcase some examples to illustrate its power and flexibility.
1. Understanding Macros
The Macroable trait is Laravel’s secret sauce behind this feature. By default, many of Laravel’s core classes use this trait, allowing them to be “macroed”. These classes include:
– `Illuminate\Support\Collection`
– `Illuminate\Support\Str`
– `Illuminate\Http\Response`
– And many others…
When you define a macro on any of these classes, you’re effectively adding a new method to it.
2. Basic Example
Imagine you’re frequently checking if a collection is not only non-empty but also has more than one item. Instead of writing this logic repeatedly, you can define a macro.
```php use Illuminate\Support\Collection; Collection::macro('hasMultiple', function () { return $this->count() > 1; }); $items = collect([1, 2]); if ($items->hasMultiple()) { echo "The collection has multiple items!"; } ```
This is a simplistic illustration, but it emphasizes how seamlessly the custom method integrates with the collection instance.
2.1 Str::macro Example
Suppose you often find yourself needing to prefix a string with a certain set of characters. Using Laravel’s string helper, `Str`, this becomes an effortless task.
```php use Illuminate\Support\Str; Str::macro('prefixMr', function ($name) { return 'Mr. ' . $name; }); echo Str::prefixMr('Smith'); // Outputs: Mr. Smith ```
2.2 Extending Responses
At times, you might want to send custom responses from your controller. For instance, a JSON response that always sends additional meta data. Macros can help!
```php use Illuminate\Http\Response; Response::macro('jsonWithMeta', function ($data, $meta = [], $status = 200, array $headers = [], $options = 0) { $formattedResponse = [ 'data' => $data, 'meta' => $meta ]; return new Response(json_encode($formattedResponse, $options), $status, $headers); }); // Using the macro in a controller: return response()->jsonWithMeta( ['name' => 'John'], ['timestamp' => now()] ); ```
The above example will generate a JSON response like:
```json { "data": { "name": "John" }, "meta": { "timestamp": "2023-09-22 10:00:00" } } ```
2.3 Binding Singletons with Macros
Sometimes, you may want to bind a singleton service into the service container and then add a macro to conveniently resolve it.
```php app()->singleton('customLogger', function () { return new \Monolog\Logger('custom'); }); Response::macro('withLog', function ($message, $data) { app('customLogger')->info($message, $data); return $this; }); // Now, in a controller or middleware: return response('Hello World')->withLog('User accessed the endpoint.', ['user_id' => 1]); ```
3. Tips on Using Macros
- Be Cautious: While macros are powerful, they can also be a source of confusion if overused. It’s important to ensure that the functionality you’re adding is generic enough to be a good fit as a macro.
- Documentation: Since macros add methods to classes at runtime, they won’t be evident in the class source code. Ensure you document any macros you create so other developers on the team are aware.
- Avoid Overwriting: Ensure that your macro’s name doesn’t conflict with existing methods. Check with methods like `$collection->hasMethod(‘methodName’)` before defining a macro.
Conclusion
Laravel’s Macros are a testament to the framework’s commitment to developer happiness. They offer a level of flexibility that’s unparalleled, allowing developers to bend the framework to their application-specific needs without forking or making core modifications. Whether it’s for custom collection operations, tailored string manipulations, or specialized responses, macros provide a seamless way to supercharge your Laravel experience. Happy coding!
Table of Contents
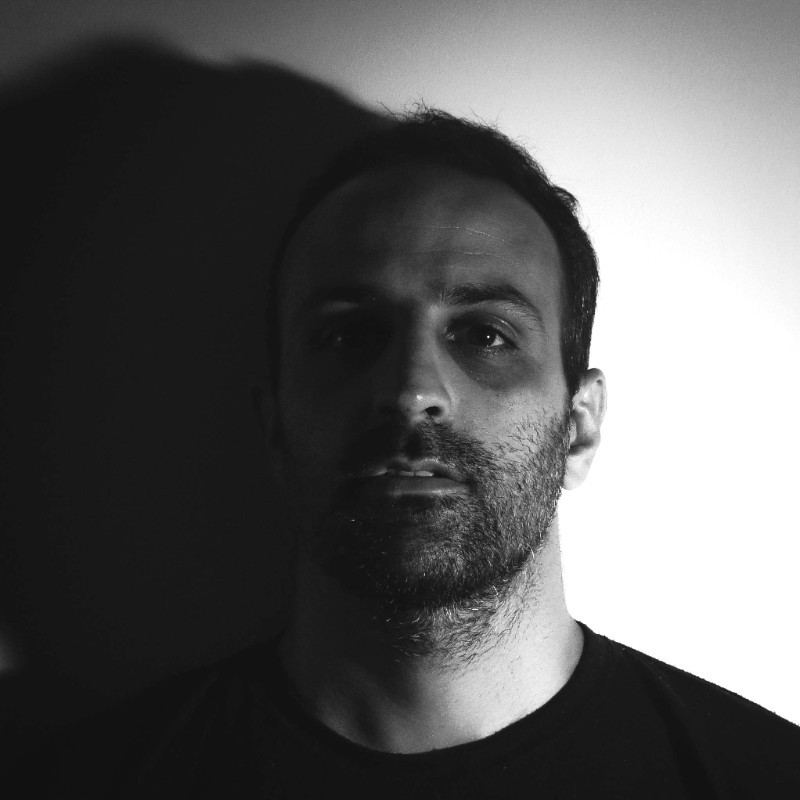
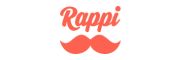