Embrace Global Audience: Build Multi-Language Applications with Laravel Localization
Web applications are inherently global by design, making it critical to cater to a diverse user base with varying language preferences. This crucial aspect of modern web development is well-understood by Laravel, one of the most popular PHP frameworks.
Table of Contents
The decision to hire Laravel developers is often driven by the framework’s robust features for building multi-language applications, including its standout feature – Localization. In this post, we delve into how you can leverage Laravel’s localization features, a task made simpler when you hire Laravel developers, to create an inclusive, multi-language application.
1. Introduction to Laravel Localization
Laravel’s localization features provide an easy way to retrieve strings in various languages, thereby allowing you to support multiple languages within your application. It uses “language files” to store all the strings of a text, organized by languages.
These language files are stored in the `resources/lang` directory. Within this directory, there should be a subdirectory for each language supported by the application (English, French, German, etc.), named using the ISO 639-1 language codes like ‘en’, ‘fr’, ‘de’, etc.
Each of these subdirectories contains a series of PHP files that return an array of keyed strings. For example, if your application has English and French support, the language files should be organized as follows:
``` /resources /lang /en messages.php /fr messages.php ```
In `messages.php` for English and French, you might have:
```php // resources/lang/en/messages.php return [ 'welcome' => 'Welcome to our application', ]; ```
```php // resources/lang/fr/messages.php return [ 'welcome' => 'Bienvenue dans notre application', ]; ```
In your views, you may then retrieve these strings using the `__` helper function provided by Laravel, which will automatically use the correct language:
```php echo __('messages.welcome'); ```
2. Setting the Locale
The `app.locale` configuration value in the `config/app.php` file determines the default locale that will be used by the `__` translation function. For instance, if the default locale is set to ‘en’, Laravel will use the English language files:
```php // config/app.php 'locale' => 'en', ```
However, you can change the active locale at runtime using the `setLocale` method on the `App` facade:
```php use Illuminate\Support\Facades\App; App::setLocale('fr'); ```
3. User Preferred Locale
You may want to allow users to select their preferred locale and store it in the database. You could then retrieve this value and set it as the application locale each time a user interacts with your application.
In the User model:
```php // app/Models/User.php public function preferredLocale() { return $this->locale; } ```
In a middleware:
```php // app/Http/Middleware/SetUserLocale.php use Illuminate\Support\Facades\App; public function handle($request, Closure $next) { if (auth()->user() && auth()->user()->preferredLocale()) { App::setLocale(auth()->user()->preferredLocale()); } return $next($request); } ```
Don’t forget to register the middleware in your `Kernel.php` file:
```php // app/Http/Kernel.php protected $middlewareGroups = [ 'web' => [ // ... \App\Http\Middleware\SetUserLocale::class, ], ]; ```
4. Fallback Locale
In addition to the current locale, you may also configure a “fallback locale” in the `config/app.php` file. This language will be used when the active language does not contain a given translation string:
```php // config/app.php 'fallback_locale' => 'en', ```
5. Localization in the View
In your Blade views, you can use the `@lang` directive:
```html <div> @lang('messages.welcome') </div> ```
6. Pluralization
Laravel’s translator also supports pluralization. In your language files, use the “pipe” character to distinguish singular and plural forms:
```php // resources/lang/en/messages.php return [ 'apples' => 'There is one apple|There are many apples', ]; ```
You can then use the `trans_choice` function in your views:
```html <div> {{ trans_choice('messages.apples', 10) }} </div> ```
7. Localization in Validation
You can also use localization in form validation. Let’s say you have a `validation.php` language file:
```php // resources/lang/en/validation.php return [ 'required' => 'The :attribute field is required.', ]; ```
In your validation code, Laravel will automatically use the correct language:
```php $request->validate([ 'title' => 'required|unique:posts|max:255', 'body' => 'required', ]); ```
Conclusion
Laravel’s localization features offer an efficient way to handle multiple languages in your application, enhancing the user experience by allowing them to interact in their preferred language. This capability is essential in today’s global digital landscape, and it’s one of the reasons why many businesses choose to hire Laravel developers.
These experts know how to use Laravel’s comprehensive and efficient features, like localization, to their full potential. By hiring Laravel developers, you’re investing in a robust, user-friendly multilingual platform.
With Laravel Localization, you can confidently greet your users in a way that feels most familiar to them, whether that’s “Hello, World!”, “Bonjour, le monde!”, “Hallo, Welt!”, or any other greeting in your user’s preferred language.
Table of Contents
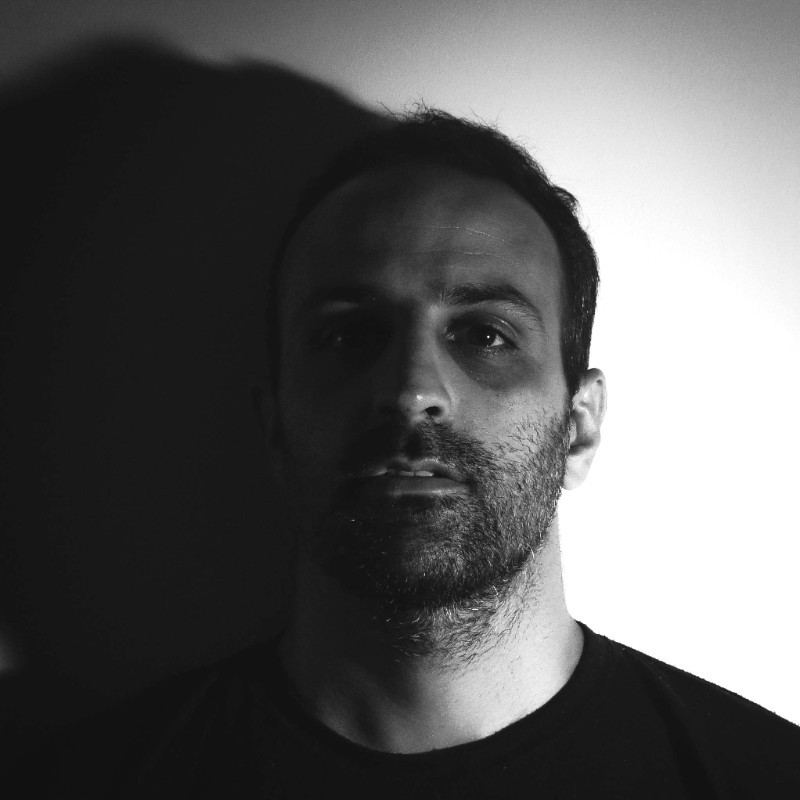
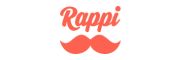