Get the Most Out of Laravel: Performance Monitoring and Optimization Explored
Laravel, an incredibly popular PHP framework, offers extensive functionality and ease of use. However, like any other framework or tool, its efficiency largely depends on the proficiency of the Laravel developers using it and how effectively its performance is monitored. This is why many businesses choose to hire Laravel developers to leverage the framework’s capabilities fully.
In this blog post, we will delve into Laravel Performance Monitoring. We will discuss methodologies, often employed by skilled Laravel developers, to track and optimize Laravel’s performance. Whether you’re looking to learn these strategies or planning to hire Laravel developers, this guide will provide valuable insights for your journey.
Table of Contents
1. Understanding Laravel Performance
Before getting into the nitty-gritty of monitoring and optimization, it’s crucial to understand what Laravel performance entails. Laravel performance usually refers to how quickly and efficiently a Laravel-based application can process requests and return responses. It includes factors such as response time, load time, database query speed, and resource utilization.
Having a high-performing Laravel application means you have an application that offers excellent user experience, higher search rankings, and better scalability.
2. Tracking Laravel Performance
2.1 Laravel Debugbar
One of the simplest ways to monitor Laravel performance is using the Laravel Debugbar. This package adds a developer toolbar to your application for easy debugging. It displays information about route, controller, views, cache, database queries and more, which can be immensely helpful in identifying performance bottlenecks.
To add Laravel Debugbar to your project, use composer:
```bash composer require barryvdh/laravel-debugbar --dev ```
After setting it up, you will see a debug bar at the bottom of your application providing insightful information about your Laravel application’s performance.
2.2 Laravel Telescope
Laravel Telescope is a powerful Laravel package for debugging and performance monitoring. It provides insights into requests, exceptions, database queries, queued jobs, mail, notifications, cache and more.
To install Laravel Telescope, use the following composer command:
```bash composer require laravel/telescope ```
After that, publish its assets using the `artisan` command:
```bash php artisan telescope:install ```
To access Laravel Telescope dashboard, append `/telescope` to your application’s base URL.
3. Optimizing Laravel Performance
3.1 Optimize Classmap
In a large Laravel application, the system has to sift through many files to find the appropriate one. By using Composer’s `dump-autoload` command, we can generate a class map. The class map is essentially a giant array that Composer uses to look up classes and find files more quickly.
```bash composer dump-autoload --optimize ```
3.2 Cache Configuration
Caching your configuration can significantly boost your Laravel application’s performance. Laravel uses a cache to remember your configuration settings instead of retrieving them from .env file on every request.
To cache your configuration settings, use the following command:
```bash php artisan config:cache ```
Remember, every time you change a configuration or environment variable, you need to refresh your configuration cache.
3.3 Optimize Routes
In Laravel, each request runs through a route matching process. If you have a large number of routes, this can become a performance issue. Laravel offers a solution by allowing you to cache routes.
```bash php artisan route:cache ```
This command will speed up your route registration, but remember, it should only be used in a production environment because you need to refresh your route cache every time you make changes to your routes.
3.4 Eager Loading
Laravel’s Eloquent ORM offers a way to work with related database objects through relationships. However, if you’re not careful, you might end up making unnecessary database queries, a common issue known as the “N+1” problem.
Eager loading is a method to load all necessary data in one database query, thereby reducing the number of queries and improving performance.
Here’s an example of lazy loading:
```php $books = App\Models\Book::all(); foreach ($books as $book) { echo $book->author->name; } ```
In the above example, for each book, Laravel will execute an additional query to get the author’s name.
Now, let’s see how eager loading can optimize this:
```php $books = App\Models\Book::with('author')->get(); foreach ($books as $book) { echo $book->author->name; } ```
With the `with(‘author’)` method, Laravel will only make two queries, regardless of the number of books: one for the books and one for the authors.
4. Advanced Optimization Tools
Beyond the built-in optimization features in Laravel, there are also third-party tools and services that provide additional ways to monitor and optimize performance.
4.1 Blackfire.io
Blackfire.io is a sophisticated tool that can help you find performance bottlenecks in your application. It not only measures the response time of your application, but it also details the performance cost of each method in your application. Blackfire.io provides visual reports, making it easier to identify problem areas.
4.2 New Relic
New Relic is another great tool to monitor Laravel applications. It provides real-time insights into how your application is performing in terms of transactions, error rate, response times, and throughput.
Conclusion
Performance monitoring and optimization are essential aspects of Laravel application development. These skills are a fundamental part of what makes experienced Laravel developers so valuable. They ensure not only an excellent user experience but also contribute to creating scalable and maintainable applications.
Implementing these strategies, which Laravel developers are often well-versed in, can help identify potential performance issues, track them, and most importantly, optimize them for better performance. Remember, the goal of performance optimization is not merely to make your Laravel application fast but to make it reliably fast.
It’s also critical to understand that performance optimization is not a one-time activity but a continuous process. Whether you’re a Laravel developer or you hire Laravel developers, constant vigilance in monitoring and optimization is vital. This way, you can ensure your Laravel application stays performant as it scales.
Table of Contents
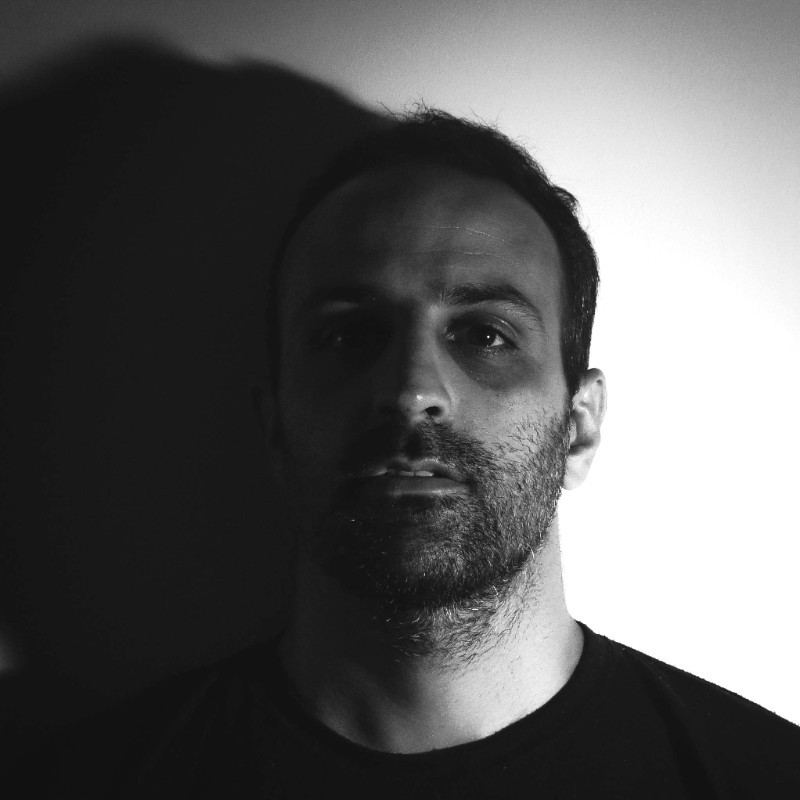
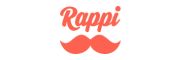