Rev Up Your Laravel Application: Performance Optimization Techniques Uncovered
In the world of web development, performance is a key factor in ensuring the success of a project. Laravel, a popular open-source PHP framework, offers developers an easy-to-use, elegant syntax for creating applications. Despite its many advantages, you may still encounter performance challenges with your Laravel application. This is where the decision to hire Laravel developers can be beneficial. This blog post will walk you through some of the most effective Laravel performance optimization techniques, which can be efficiently implemented by skilled Laravel developers, to help you build faster, more responsive applications.
1. Optimize Your Database Queries
The database is often the bottleneck when it comes to web application performance. Laravel provides a powerful Query Builder and Eloquent ORM, but it’s still crucial to optimize your database queries.
Use Eager Loading
By default, Laravel uses “lazy loading,” which means related data is not loaded until it’s explicitly requested. While this is helpful in some scenarios, it can lead to what’s known as the “N+1 problem.” Here, Laravel will execute one database query to retrieve the initial data, plus an additional query for each related record. This results in multiple, unnecessary database queries.
Eager loading, on the other hand, resolves this issue by loading all related data in a single database query. In Laravel, you can achieve eager loading using the `with()` method. Here’s an example:
```php $users = App\Models\User::with('orders')->get(); ```
In this case, Laravel will execute only two queries, irrespective of the number of users or orders.
Use Indices
Database indices can greatly enhance query performance by speeding up data retrieval. Always ensure to add indices to columns that are frequently queried. However, be cautious, as excessive indexing can slow down write operations.
2. Caching
Caching is another essential technique for improving Laravel performance. Laravel provides robust support for different caching systems, including Memcached and Redis.
Route Caching
Laravel allows you to cache all routes, significantly improving the performance of a large application. This is particularly useful in applications with numerous routes.
```php php artisan route:cache ```
Note that route caching is not advisable in development since any changes made won’t take effect until the cache is refreshed.
Config Caching
Similar to route caching, Laravel allows config caching. This is especially beneficial if your application has many configuration files.
```php php artisan config:cache ```
Again, avoid config caching during development because changes in config files won’t reflect until the cache is cleared.
Cache Database Queries
Frequently run database queries should be cached to avoid multiple identical requests. Laravel offers a simple API for caching these queries:
```php $users = Cache::remember('users', $minutes, function () { return DB::table('users')->get(); }); ```
Here, Laravel will first attempt to retrieve `users` from the cache. If it doesn’t exist, it’ll execute the closure, cache the result, and then return the data.
3. Laravel’s Built-in Artisan Commands
Laravel includes a command-line interface called Artisan that provides several helpful commands to boost your app’s performance.
Optimize Classmap
The `optimize` command is used to generate a single file containing all classes used by the application, reducing the need for file system lookups.
```php php artisan optimize ```
Compile Views
Compiling all Blade views into plain PHP code can significantly speed up your application.
```php php artisan view:cache ```
This command is particularly useful in production since views rarely change.
4. Using Laravel Mix for Asset Optimization
Laravel Mix, a fluent API for defining webpack build steps, provides several options for optimizing your CSS and JavaScript assets.
You can minify your CSS and JavaScript files, which reduces their size and improves load times. Here’s how you can do it:
```javascript const mix = require('laravel-mix'); mix.js('resources/js/app.js', 'public/js') .sass('resources/sass/app.scss', 'public/css') .minify(['public/js/app.js', 'public/css/app.css']); ```
5. Reducing Use of Plugins and Libraries
While Laravel’s ecosystem is rich with packages that can speed up your development process, they can also bog down your application. Be selective about the packages you include in your project. Only use those necessary for your app and always keep them updated.
6. Session Driver
Choose your session driver carefully. While file-based sessions are easier to implement, they may cause performance issues for larger applications. Laravel supports several session backends like Memcached and Redis which offer better performance.
7. Deploy on PHP7
PHP 7.x versions significantly outperform their PHP 5.x counterparts. If possible, ensure that your server is running a supported PHP 7.x version.
Conclusion
As we have seen, Laravel offers a wide range of features and tools for performance optimization. Implementing these techniques, often best handled by hiring experienced Laravel developers, can significantly enhance the performance and responsiveness of your Laravel applications. Always remember, optimization is not a one-time activity but a continuous process. It’s crucial to routinely monitor your application performance and apply necessary optimizations, or consider hiring Laravel developers to ensure a swift and seamless user experience.
Table of Contents
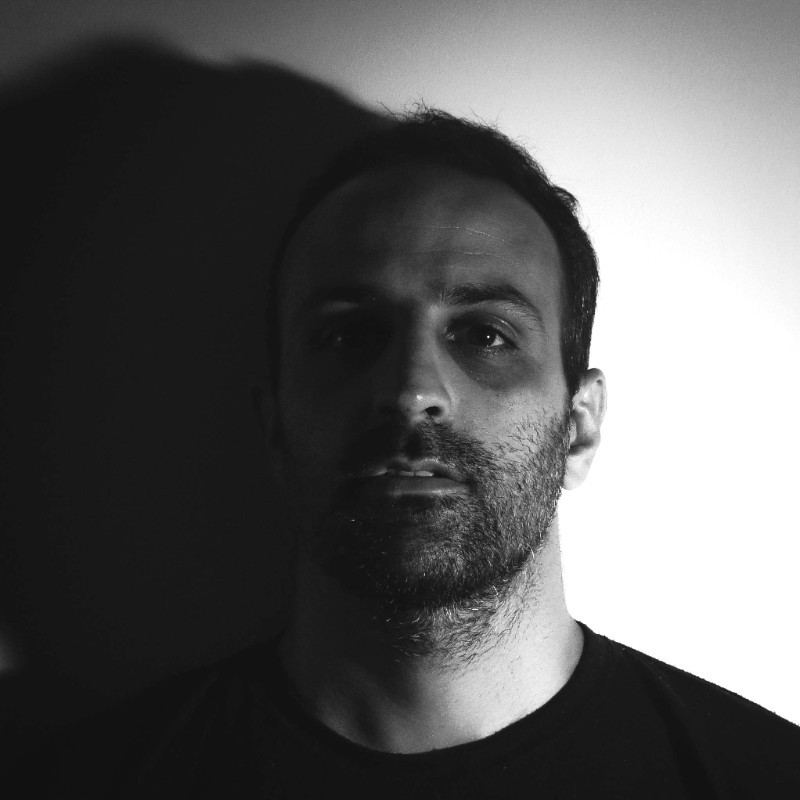
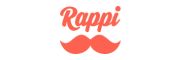