Authorization Made Simple: Laravel’s Must-Know Features of Policies and Gates
Laravel, the elegant PHP framework for web artisans, offers a comprehensive set of tools for building robust and secure applications. One of the areas where Laravel truly shines is in its approach to handling authorization. With built-in features like Policies and Gates, Laravel provides a simple and clean way to manage access to different parts of your application.
In this blog post, we’ll delve into Laravel Policies and Gates, explaining how they work and providing practical examples. Let’s start the journey!
1. What are Laravel Policies?
Policies in Laravel provide a straightforward way to organize authorization logic around a particular model or resource. If you’ve ever needed to determine if a user can view, edit, or delete an article, for example, a Policy can be your best friend.
2. Creating a Policy
Let’s say we’re building a blog and want to determine who can edit a blog post. First, we’d generate a Policy using the `artisan` command:
```bash php artisan make:policy PostPolicy --model=Post ```
This command will create a `PostPolicy` class in the `app/Policies` directory. If you open this file, you’ll find several pre-defined methods like `view`, `create`, `update`, and `delete`.
3. Implementing the Policy
In the `PostPolicy`, you can define the authorization logic for updating a post:
```php public function update(User $user, Post $post) { return $user->id === $post->user_id; } ```
Here, we’re saying that a post can only be updated by its creator (or owner).
4. Using the Policy
In a controller or anywhere in your application, you can now easily check if a user is authorized to update a post:
```php if ($user->can('update', $post)) { // The user is allowed to update the post... } ```
5. What are Laravel Gates?
While Policies are tailored for specific models or resources, Gates provide a more general way to filter access. Gates are Closures that determine if a user is authorized to perform a given action.
6. Defining a Gate
Gates are typically defined in the `AuthServiceProvider` in the `boot` method. Here’s an example where we determine if a user is an admin:
```php Gate::define('isAdmin', function ($user) { return $user->role == 'admin'; }); ```
7. Using the Gate
You can now use this gate in your application to determine if a user has administrative privileges:
```php if (Gate::allows('isAdmin')) { // The current user is an admin... } // Alternatively: if (Gate::denies('isAdmin')) { // The current user is not an admin... } ```
8. Combining Policies and Gates
For a more robust authorization system, you can combine Policies and Gates. For instance, while a Gate checks if a user is an admin, a Policy can determine if the same user is the owner of a particular resource.
9. Before Middleware
You can also use Policies and Gates in routes or controllers using the `middleware` method:
```php Route::put('/post/{post}', 'PostController@update')->middleware('can:update,post'); ```
Here, the `update` action on the `PostController` is only accessible if the user is authorized to update the given post.
Conclusion
Laravel’s Policies and Gates offer a clean, elegant, and efficient way to handle authorization. By understanding and utilizing these features, developers can ensure their applications are not only secure but also maintainable and scalable. Whether it’s a simple blog or a complex enterprise application, Laravel’s authorization tools make it easier to manage user access and privileges. So, the next time you’re wondering how to handle that tricky authorization logic, just remember: Laravel’s got your back!
Table of Contents
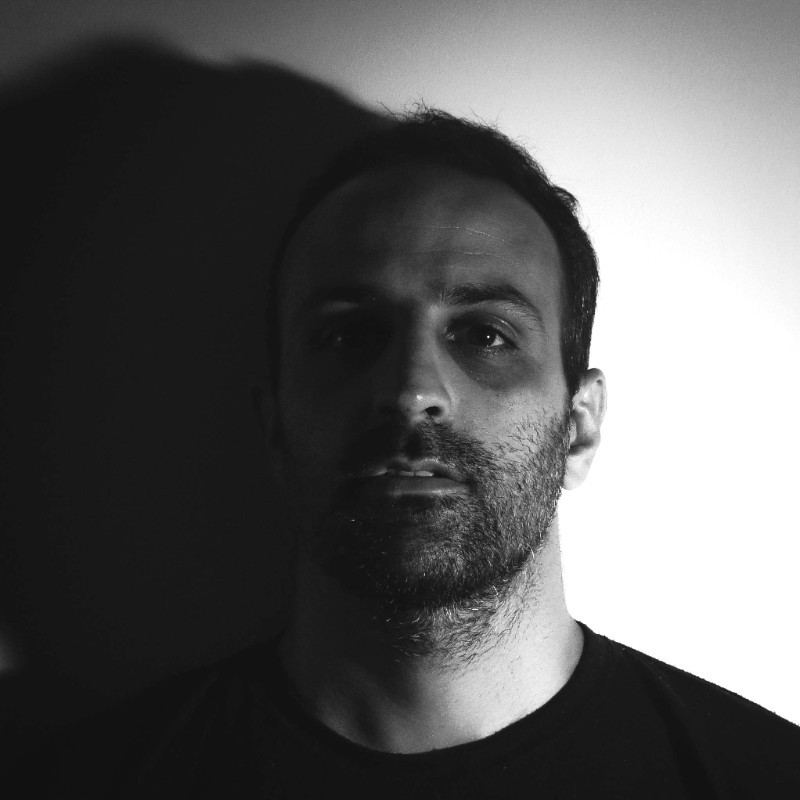
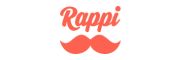