Experience Lightning-Fast Searches in Laravel with Scout & Algolia
Search functionality is paramount for any web application. Users expect smooth, fast, and relevant search results. While Laravel, a PHP framework, offers basic search capabilities, combining it with Algolia elevates the search experience. Laravel Scout is a package that bridges the gap between Laravel and Algolia, providing powerful search capabilities to Laravel applications.
In this blog post, we’ll dive deep into integrating Laravel Scout with Algolia, followed by examples that will help you understand its full potential.
1. What is Algolia?
Algolia is a hosted search engine platform that provides lightning-fast search results with typo-tolerant features. It’s optimized for high-speed, relevant search experiences. Its infrastructure is distributed and redundant, which ensures maximum uptime and speed.
2. What is Laravel Scout?
Laravel Scout is an official Laravel package that provides a simple, driver-based solution for adding full-text search functionality to your Eloquent models. While it supports multiple drivers, Algolia is one of the most popular ones.
3. Integration of Laravel Scout with Algolia
3.1. Installation
Begin by pulling in the Scout package using Composer:
```bash composer require laravel/scout ```
Then, publish the Scout configuration using:
```bash php artisan vendor:publish --provider="Laravel\Scout\ScoutServiceProvider" ```
Once Scout is installed, update your `.env` file with your Algolia credentials:
``` ALGOLIA_APP_ID=YourAlgoliaAppId ALGOLIA_SECRET=YourAlgoliaAdminApiKey ```
3.2. Making your model searchable
To make a model searchable, use the `Searchable` trait on the model:
```php use Laravel\Scout\Searchable; class Post extends Model { use Searchable; } ```
Now, any time you save a model, it’ll be automatically indexed in Algolia!
Examples:
- Basic search
```php // Searching for posts containing "Laravel" $posts = App\Post::search('Laravel')->get(); ```
- Pagination
Algolia’s speed makes it ideal for paginating results:
```php $posts = App\Post::search('Laravel')->paginate(10); ```
- Where Clauses
Scout provides a method for adding “where” clauses to your search queries:
```php // Getting all published posts about "Laravel" $posts = App\Post::search('Laravel') ->where('published', 1) ->get(); ```
- Custom Indexing
By default, all of the model’s attributes are searchable. But you can customize the data to be indexed by overriding the `toSearchableArray` method:
```php public function toSearchableArray() { return [ 'title' => $this->title, 'body' => $this->body, 'author_name' => $this->author->name, ]; } ```
4. Benefits of Using Laravel Scout with Algolia
- Speed: Algolia returns results in milliseconds, enhancing the user experience.
- Typo-tolerance: Even if a user makes a typo, Algolia intelligently guesses what they intended to type.
- Relevance: Algolia’s ranking formula ensures the most relevant results show up first.
- Scalability: Suitable for applications of any size, from a few records to millions.
- Laravel Integration: Scout integrates seamlessly, making it incredibly easy to index and search Eloquent models.
Conclusion
The combination of Laravel Scout and Algolia provides a powerful, seamless, and efficient way to implement search functionality in a Laravel application. The integration is smooth, and the resulting search experience is nothing short of exceptional. If you’re building a Laravel application that needs search functionality, look no further than Scout and Algolia. With the examples provided above, you can start implementing today and offer your users an unparalleled search experience.
Table of Contents
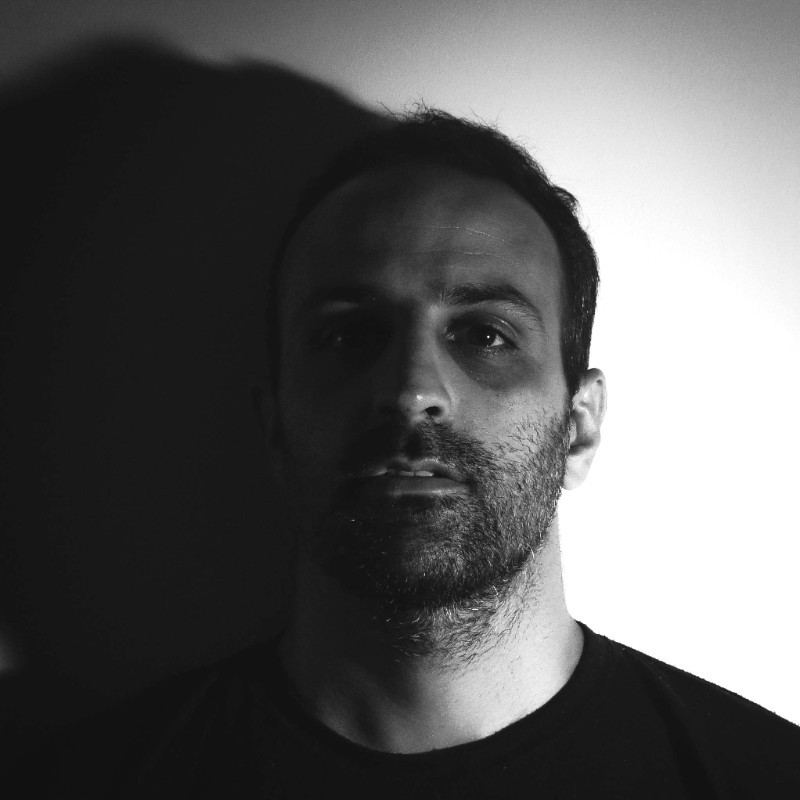
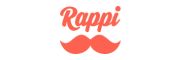