How to implement search functionality in Laravel?
Implementing search functionality in Laravel is akin to organizing a library—it enables users to find relevant information quickly and efficiently within your application. Here’s a user-friendly guide on how to implement search functionality in Laravel:
Define Searchable Models: Start by identifying the models in your Laravel application that you want to make searchable. These could be models representing articles, products, users, or any other entities that users might want to search for. Ensure that these models use the Searchable trait provided by Laravel Scout.
Install and Configure Laravel Scout: Laravel Scout is a powerful package that provides full-text search capabilities to your Laravel application. Install Laravel Scout and its driver package (such as Algolia or Elasticsearch) using Composer:
bash composer require laravel/scout
Configure your Scout driver in the config/scout.php configuration file, specifying the appropriate credentials and settings for your chosen search engine.
Make Models Searchable: Use the Searchable trait provided by Laravel Scout to make your models searchable. Simply add the Searchable trait to your model classes and define the searchable attributes that you want to include in the search index.
Index Data: Once your models are searchable, use the scout:import Artisan command to index data into your chosen search engine. This command will sync your database records with the search index, making them searchable via Scout.
Implement Search Logic: Implement search logic in your application to allow users to search for relevant content. Create a search form or input field in your application’s frontend, allowing users to enter search queries.
Perform Search Queries: When a user submits a search query, use Laravel Scout’s search functionality to perform full-text search queries against the search index. Use the search method provided by the Searchable trait to execute search queries on your models:
php $results = YourModel::search($query)->get();
Replace YourModel with the name of your searchable model class and $query with the user’s search query.
Display Search Results: Finally, display the search results to the user in your application’s frontend. Iterate over the search results and present them in a user-friendly format, such as a list or grid of search results.
Optional: Implement Pagination and Filtering: For large datasets, consider implementing pagination to break search results into multiple pages. Additionally, you can provide filtering options to allow users to refine their search results based on specific criteria.
By following these steps, you can implement search functionality in your Laravel application, allowing users to search for relevant content quickly and efficiently. Laravel Scout simplifies the process of adding full-text search capabilities to your application, making it easy to build powerful search functionality that enhances the user experience.
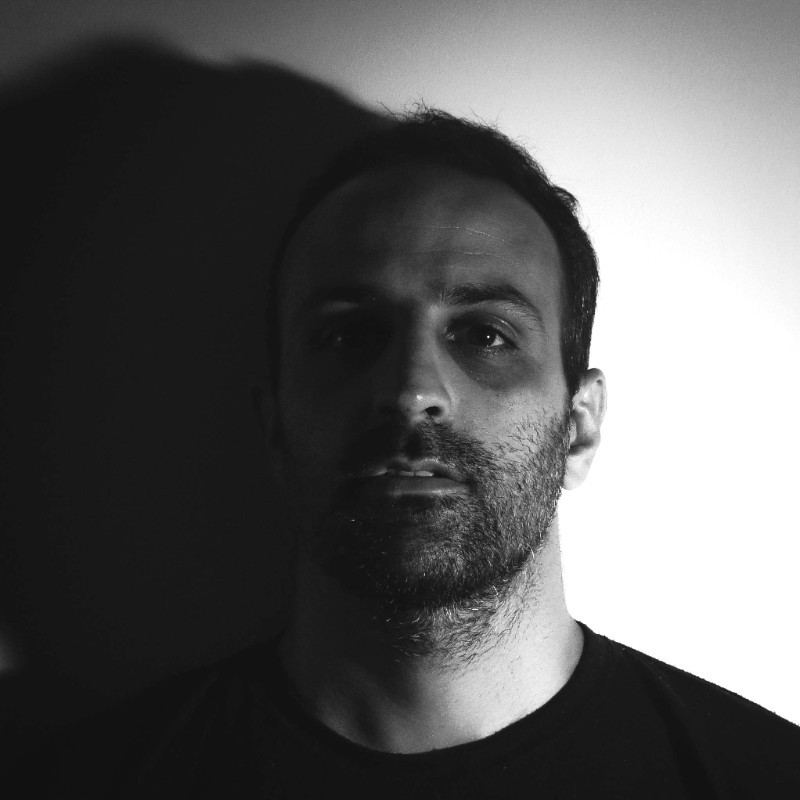
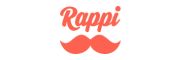