How to implement social authentication with Laravel Socialite?
Implementing social authentication with Laravel Socialite is like opening your doors to a broader audience—it allows users to log in to your application using their existing social media accounts, making the login process seamless and convenient. Here’s how you can implement social authentication with Laravel Socialite in your Laravel application:
Install Laravel Socialite: The first step is to install Laravel Socialite via Composer. You can do this by running the following command in your terminal:
bash composer require laravel/socialite
Configure Socialite Providers: Laravel Socialite supports various OAuth providers out of the box, including Facebook, Twitter, Google, GitHub, and more. To use a specific provider, you need to configure its credentials in your Laravel application’s config/services.php file. Each provider requires specific client ID, client secret, and callback URL settings, which you obtain by creating an application with the respective OAuth provider.
Redirect Users to Social Authentication Provider: In your Laravel application’s login page, add buttons or links for users to log in using their social media accounts. When a user clicks on one of these buttons, you’ll need to redirect them to the corresponding OAuth provider’s authorization URL. Laravel Socialite provides a convenient method for initiating the OAuth authentication flow:
php return Socialite::driver('facebook')->redirect();
Handle OAuth Callback: After the user authorizes your application, the OAuth provider redirects them back to your application’s callback URL along with an authorization code. You’ll need to handle this callback and exchange the authorization code for an access token using Laravel Socialite’s stateless method:
php $user = Socialite::driver('facebook')->stateless()->user();
Retrieve User Information: Once you have the user’s access token, you can use it to retrieve their profile information from the OAuth provider. Laravel Socialite provides a user() method that returns a user object containing the user’s profile data:
php $user = Socialite::driver('facebook')->user();
Authenticate User: Finally, you can authenticate the user in your Laravel application using their social media profile information. You may need to check if the user already exists in your application’s database and create a new user record if they don’t.
Handle Error Cases: It’s essential to handle error cases gracefully, such as when the user cancels the authentication process or when there’s an error retrieving user information from the OAuth provider. Laravel Socialite provides methods for handling these scenarios and returning appropriate error messages to the user.
By following these steps, you can seamlessly integrate social authentication into your Laravel application using Laravel Socialite. Social authentication provides a convenient login option for users and enhances the user experience of your application.
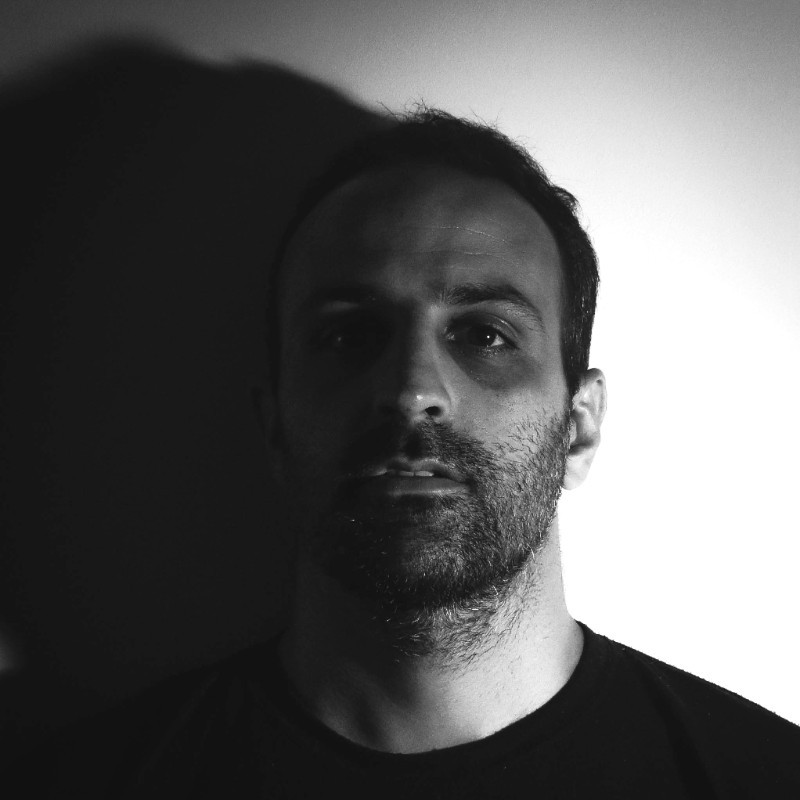
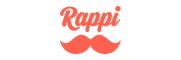