Streamlining User Authentication in Laravel with Social Logins
As the world of web development continues to evolve, it becomes more and more essential to streamline online interactions for users. One significant aspect of this evolution is user authentication. While the traditional method of logging in with an email and password still serves its purpose, the advent of social logins provides a quicker and arguably safer alternative. Businesses increasingly opt to hire developers who can efficiently implement these features.
Table of Contents
In this blog post, we’ll explore how to integrate social login functionality into a Laravel application using Laravel Socialite. This package, developed by Laravel, simplifies the process, making it an excellent task for companies to hire developers for. Laravel Socialite currently supports authentication with Facebook, Twitter, LinkedIn, Google, GitHub, and Bitbucket, offering a broad range of options for user convenience.
1. What is Laravel Socialite?
Laravel Socialite is an OAuth authentication wrapper built into the Laravel framework. It provides developers with a straightforward and fluent interface for authenticating users via various social networking services.
Let’s dive into how we can use Socialite to implement social logins with three popular platforms: Google, Facebook, and GitHub.
2. Setting up Laravel and Socialite
Before we begin, you need to have Laravel and Composer installed on your local machine. Laravel Socialite is also installed via Composer. Run the following command in your terminal:
```bash composer require laravel/socialite ```
Once installed, add the Socialite service provider in your `config/app.php` file:
```php 'providers' => [ // Other service providers... Laravel\Socialite\SocialiteServiceProvider::class, ], ```
You should also add the Socialite facade to the aliases array in your `config/app.php` file:
```php 'aliases' => [ // Other aliases... 'Socialite' => Laravel\Socialite\Facades\Socialite::class, ], ```
3. Google Login with Laravel Socialite
Firstly, you will need to create a new project in the [Google Cloud Console](https://console.cloud.google.com/), after which you can get your Client ID and Client Secret.
In your `config/services.php` file, add your Google client id, client secret, and redirect URL:
```php 'google' => [ 'client_id' => env('GOOGLE_CLIENT_ID'), 'client_secret' => env('GOOGLE_CLIENT_SECRET'), 'redirect' => env('GOOGLE_REDIRECT_URL'), ], ```
Remember to update your `.env` file with the appropriate values.
Next, define routes in your `web.php` file:
```php Route::get('login/google', [LoginController::class, 'redirectToGoogle']); Route::get('login/google/callback', [LoginController::class, 'handleGoogleCallback']); ```
Then, in your `LoginController.php`, add the following methods:
```php public function redirectToGoogle() { return Socialite::driver('google')->redirect(); } public function handleGoogleCallback() { $user = Socialite::driver('google')->user(); // $user->token; } ```
You can retrieve the user’s details from the `$user` variable and create or find the user in your database.
4. Facebook Login with Laravel Socialite
The process for Facebook is quite similar to Google’s. You will first need to create an app on the [Facebook Developers](https://developers.facebook.com/) platform. After creating the app, grab your App ID and App Secret.
Add your Facebook App ID, App Secret, and redirect URL in your `config/services.php` file:
```php 'facebook' => [ 'client_id' => env('FACEBOOK_APP_ID'), 'client_secret' => env('FACEBOOK_APP_SECRET'), 'redirect' => env('FACEBOOK_REDIRECT_URL'), ], ```
Don’t forget to update your `.env` file with the right credentials.
Now, you can define routes in your `web.php` file:
```php Route::get('login/facebook', [LoginController::class, 'redirectToFacebook']); Route::get('login/facebook/callback', [LoginController::class, 'handleFacebookCallback']); ```
And in your `LoginController.php`, add the corresponding methods:
```php public function redirectToFacebook() { return Socialite::driver('facebook')->redirect(); } public function handleFacebookCallback() { $user = Socialite::driver('facebook')->user(); // $user->token; } ```
Again, you can use the `$user` variable to find or create the user in your database.
5. GitHub Login with Laravel Socialite
For GitHub, you need to create a new OAuth application on the [GitHub Developer](https://developer.github.com/) platform. Once you have your Client ID and Client Secret, add them to your `config/services.php` file:
```php 'github' => [ 'client_id' => env('GITHUB_CLIENT_ID'), 'client_secret' => env('GITHUB_CLIENT_SECRET'), 'redirect' => env('GITHUB_REDIRECT_URL'), ], ```
Update the `.env` file with your GitHub credentials.
Define routes in your `web.php` file:
```php Route::get('login/github', [LoginController::class, 'redirectToGithub']); Route::get('login/github/callback', [LoginController::class, 'handleGithubCallback']); ```
Lastly, add these methods in your `LoginController.php`:
```php public function redirectToGithub() { return Socialite::driver('github')->redirect(); } public function handleGithubCallback() { $user = Socialite::driver('github')->user(); // $user->token; } ```
From here, you can manipulate the `$user` variable to save or authenticate the user in your application.
Conclusion
Socialite is a powerful tool in Laravel that simplifies the process of integrating social logins into your applications. This feature offers a seamless and straightforward way for users to register and login, significantly enhancing the overall user experience.
Please note, while the provided code snippets are simplified for clarity, their successful implementation requires a certain level of technical expertise. For complex tasks, you may need to hire developers to handle exceptions, ensure user persistence in your database, and manage user sessions in your application.
Remember, securing your applications and maintaining user privacy should always be at the forefront of your development process. With the right team and the right tools, you’ll be well on your way to happy coding!
Table of Contents
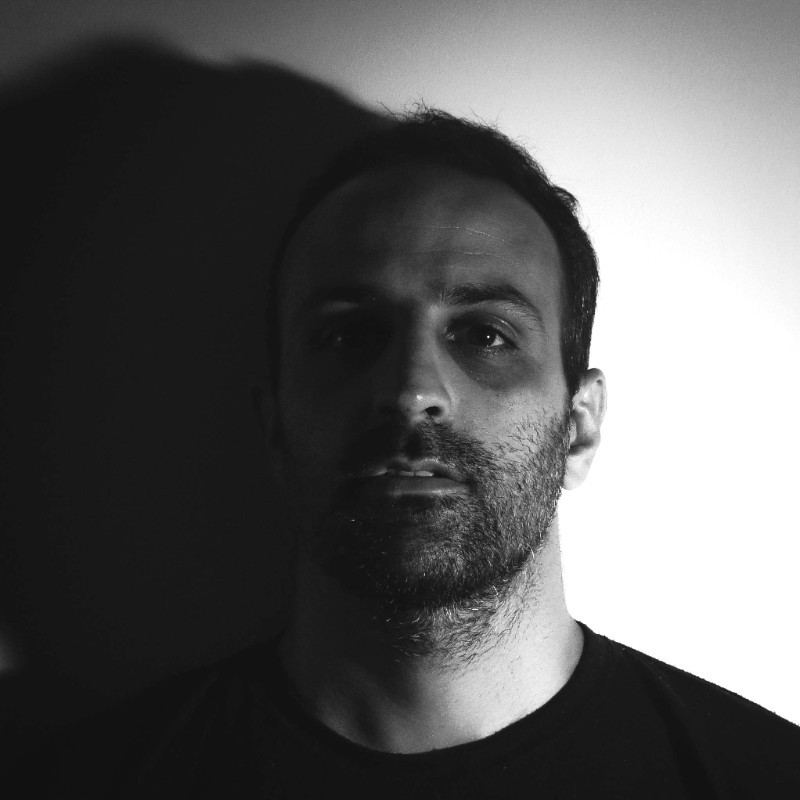
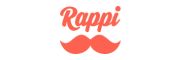