Laravel Socialite: Integrating Social Authentication into Your App
In today’s digital age, user authentication is a crucial aspect of web applications. However, creating a seamless and secure authentication system from scratch can be time-consuming and complex. That’s where Laravel Socialite comes to the rescue. Laravel Socialite is a powerful package that simplifies the process of integrating social authentication into your Laravel application, allowing users to sign in using their social media accounts like Facebook, Google, Twitter, and more. In this blog post, we’ll explore the ins and outs of Laravel Socialite and guide you through the steps of incorporating social authentication into your app.
Table of Contents
1. Introduction to Laravel Socialite
1.1. What is Social Authentication?
Social authentication, also known as social login, allows users to log into your application using their existing social media accounts. Instead of creating a new account and password, users can simply click a button associated with their preferred social platform to access your app. This not only streamlines the registration process but also eliminates the need for users to remember yet another set of login credentials.
1.2. Why Choose Laravel Socialite?
Laravel Socialite simplifies the integration of social authentication into your Laravel application. It provides an elegant, fluent interface for authenticating with various social platforms. Here are a few reasons why Laravel Socialite stands out:
- Ease of Use: Laravel Socialite abstracts the complexities of OAuth authentication, making it easy to implement social login without delving into intricate details.
- Flexibility: It supports multiple social platforms out of the box, and you can extend it to integrate with custom providers if needed.
- Security: Laravel Socialite handles secure OAuth authentication, ensuring that user data remains protected during the login process.
- Consistency: The package maintains a consistent API across different social providers, simplifying the development process.
2. Setting Up Your Laravel Project
2.1. Laravel Installation
If you haven’t already set up a Laravel project, you can create one using the Laravel installer or Composer:
bash composer create-project --prefer-dist laravel/laravel YourAppName
2.2. Composer Package Installation
Next, you’ll need to install the Laravel Socialite package. Open your terminal and navigate to your project’s directory, then run the following command:
bash composer require laravel/socialite
This will fetch the necessary files and add the package to your project.
3. Configuring Social Media Applications
3.1. Creating Social Media Apps
Before you can integrate social authentication, you need to create applications on the respective social media platforms. Here, we’ll take a look at configuring authentication for Facebook and Google.
3.2. Obtaining API Credentials
For Facebook authentication, visit the Facebook for Developers website and create a new app. Once created, you’ll be provided with an App ID and App Secret, which you’ll use in your Laravel application to connect to Facebook’s API.
For Google authentication, navigate to the Google Cloud Platform Console and create a new project. Then, enable the “Google+ API” and create credentials. You’ll receive a Client ID and Client Secret, which are required for integrating Google authentication.
4. Installing and Setting Up Laravel Socialite
4.1. Installing Socialite
With your Laravel project set up and social media app credentials in hand, it’s time to install and configure Laravel Socialite.
In your config/app.php file, add the SocialiteServiceProvider to the providers array:
php 'providers' => [ // ... Laravel\Socialite\SocialiteServiceProvider::class, ],
Then, add the Socialite facade to the aliases array in the same file:
php 'aliases' => [ // ... 'Socialite' => Laravel\Socialite\Facades\Socialite::class, ],
5. Implementing Social Authentication
5.1. Creating Authentication Routes
In your routes/web.php file, define routes for social authentication. For instance, to create routes for Facebook and Google authentication, you can add the following:
php Route::get('auth/facebook', 'AuthController@redirectToFacebook'); Route::get('auth/facebook/callback', 'AuthController@handleFacebookCallback'); Route::get('auth/google', 'AuthController@redirectToGoogle'); Route::get('auth/google/callback', 'AuthController@handleGoogleCallback');
5.2. Handling Callbacks
In the routes defined above, the AuthController handles the redirect and callback actions. Let’s create the controller and add the necessary methods:
bash php artisan make:controller AuthController
In the AuthController.php file, you’ll have methods like redirectToFacebook, handleFacebookCallback, redirectToGoogle, and handleGoogleCallback to manage the authentication flow for each platform.
6. Integrating Facebook Authentication
6.1. Configuring Facebook Provider
Open the config/services.php file and add the following configuration for Facebook:
php 'facebook' => [ 'client_id' => env('FACEBOOK_CLIENT_ID'), 'client_secret' => env('FACEBOOK_CLIENT_SECRET'), 'redirect' => env('FACEBOOK_REDIRECT'), ],
6.2. Authentication Process
In the AuthController methods, use the Socialite facade to initiate the authentication process and handle the callback:
php use Socialite; public function redirectToFacebook() { return Socialite::driver('facebook')->redirect(); } public function handleFacebookCallback() { $user = Socialite::driver('facebook')->user(); // Handle user data and authentication }
7. Enabling Google Authentication
7.1. Setting Up Google Provider
Similarly, in the config/services.php file, add the Google configuration:
php 'google' => [ 'client_id' => env('GOOGLE_CLIENT_ID'), 'client_secret' => env('GOOGLE_CLIENT_SECRET'), 'redirect' => env('GOOGLE_REDIRECT'), ],
7.2. Authentication Flow
In the AuthController methods for Google authentication:
php public function redirectToGoogle() { return Socialite::driver('google')->redirect(); } public function handleGoogleCallback() { $user = Socialite::driver('google')->user(); // Handle user data and authentication }
8. Customizing and Extending
8.1. Redirecting After Authentication
After successful authentication, you can redirect users to specific routes or pages based on your application’s logic:
php public function handleFacebookCallback() { $user = Socialite::driver('facebook')->user(); // Handle user data and authentication return redirect()->route('dashboard'); }
8.2. Accessing User Details
You can access the user’s information obtained from the social platform like this:
php $user->getId(); $user->getName(); $user->getEmail(); // …
9. Handling Errors and Exceptions
9.1. Common Issues and Solutions
While integrating social authentication, you might encounter various errors or exceptions. Some common issues include:
- Invalid Redirect URIs: Make sure the redirect URIs in your social media app configurations match the routes in your Laravel app.
- Token Mismatch Exception: If you encounter a token mismatch exception during callback handling, ensure your VerifyCsrfToken middleware excludes the social authentication routes.
10. Enhancing Security and User Experience
10.1. Storing User Information
Upon successful authentication, you can store the user’s information in your application’s database. Create a User model and migration, and implement the necessary logic to save user details.
10.2. Managing Sessions
Utilize Laravel’s built-in session management to keep users authenticated across different parts of your application. Laravel’s session handling makes it easy to manage user sessions securely.
Conclusion
Integrating social authentication into your Laravel application enhances user experience and simplifies the sign-up process. Laravel Socialite provides an elegant and secure way to achieve this without the hassle of dealing with OAuth intricacies. By following the steps outlined in this blog post, you can seamlessly integrate Facebook, Google, and other social authentication providers into your app, creating a more user-friendly and efficient experience for your users. Happy coding!
In this blog post, we’ve explored the world of Laravel Socialite and its capabilities in integrating social authentication into your Laravel application. From setting up your project and configuring social media applications to implementing authentication flows and handling user data, you now have a comprehensive guide to enhance your app’s user experience and security. So go ahead and start integrating social authentication with Laravel Socialite – your users will thank you for it!
Table of Contents
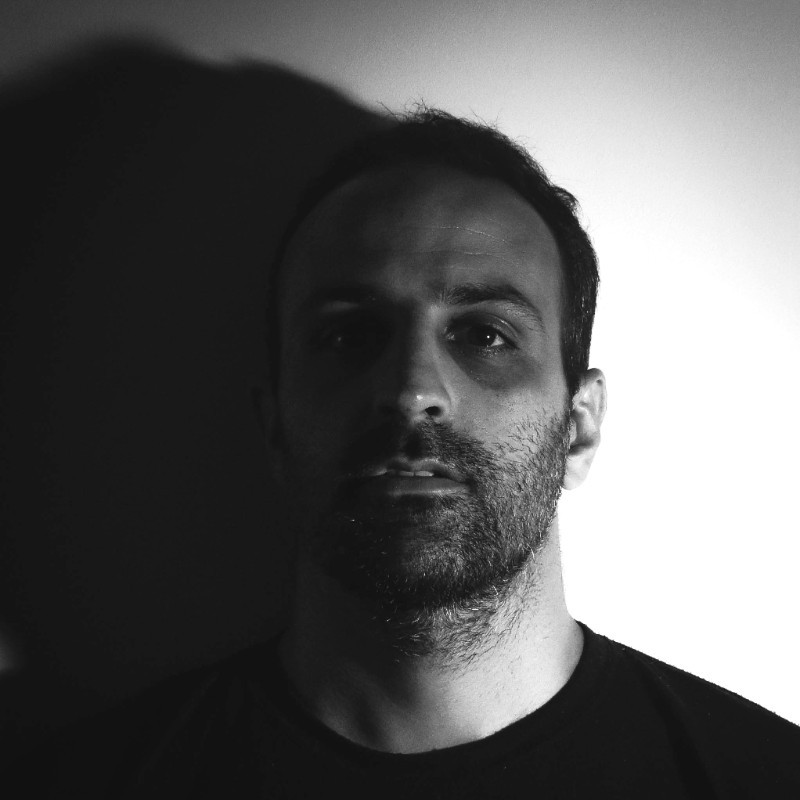
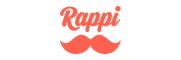