File Management in Laravel: A Deep Dive into Local & Cloud Storage Techniques
Laravel, a popular PHP framework, offers powerful tools to simplify many web application tasks. One of its valuable utilities is the Laravel Storage API, which provides a streamlined interface to work with local file systems and cloud storage solutions. In this blog post, we will explore how to use this feature to manage files and delve into some practical examples.
1. Understanding Laravel’s File System Abstraction
Laravel’s Storage facade provides a consistent API over various storage backends, leveraging the power of the Flysystem PHP package. This means you can swap out a local file system for a cloud-based one like Amazon S3 without changing your code significantly.
Out of the box, Laravel supports:
– Local storage
– Amazon S3
– FTP
– SFTP
– Rackspace
2. Configuration
Before diving into examples, let’s briefly discuss configuration.
File system configurations are stored in the `config/filesystems.php` configuration file. Here, you’ll see a `default` disk, which is used when no disk is specified during storage operations.
For example, to configure Amazon S3, you might have:
```php 's3' => [ 'driver' => 's3', 'key' => env('AWS_ACCESS_KEY_ID'), 'secret' => env('AWS_SECRET_ACCESS_KEY'), 'region' => env('AWS_DEFAULT_REGION'), 'bucket' => env('AWS_BUCKET'), ], ```
Make sure you set the appropriate environment variables in your `.env` file.
3. Working with Files on the Local File System
3.1. Storing a New File
You can use the `put` method on the Storage facade.
```php use Illuminate\Support\Facades\Storage; Storage::disk('local')->put('example.txt', 'Hello, Laravel!'); ```
3.2. Retrieving a File
To get the contents of a file:
```php $contents = Storage::get('example.txt'); ```
3.3. Checking if a File Exists
```php if (Storage::exists('example.txt')) { // File exists } ```
4. Using Cloud Storage (e.g., Amazon S3)
Switching from local to S3 is a breeze. Just replace `’local’` with `’s3’`.
4.1. Uploading a File to S3
```php Storage::disk('s3')->put('example.txt', 'Hello from S3!'); ```
4.2. Retrieving and Streaming a File
Instead of using `get`, which pulls the file into memory, you can stream it directly to the user:
```php return Storage::disk('s3')->response('example.txt'); ```
4.3. Generating Temporary URLs
This is useful if you want to provide temporary access to private files:
```php $temporaryUrl = Storage::disk('s3')->temporaryUrl( 'example.txt', now()->addMinutes(5) ); ```
5. Advanced Features
5.1. File Visibility
You can set the visibility of files as either public or private:
```php Storage::disk('s3')->put('example.txt', 'Hello', 'public'); ```
5.2. Prepending & Appending
Instead of retrieving, modifying, and storing again, you can prepend or append content directly:
```php Storage::prepend('example.txt', 'Before Hello'); Storage::append('example.txt', 'After Hello'); ```
5.3. Custom Filesystems
Laravel’s extensible nature allows you to extend the Storage facade to work with other cloud platforms or custom file systems. You can write a custom driver and register it, providing even more versatility to the framework.
Conclusion
Laravel’s Storage facade and the underlying Flysystem integration make working with files and cloud storage not only painless but also enjoyable. The ease of swapping out storage backends without major code overhauls showcases Laravel’s commitment to developer happiness and maintainability.
By familiarizing yourself with the Storage API, you’ll be better equipped to manage files in a scalable way, whether you’re serving them from your local machine or a distributed cloud-based system.
Table of Contents
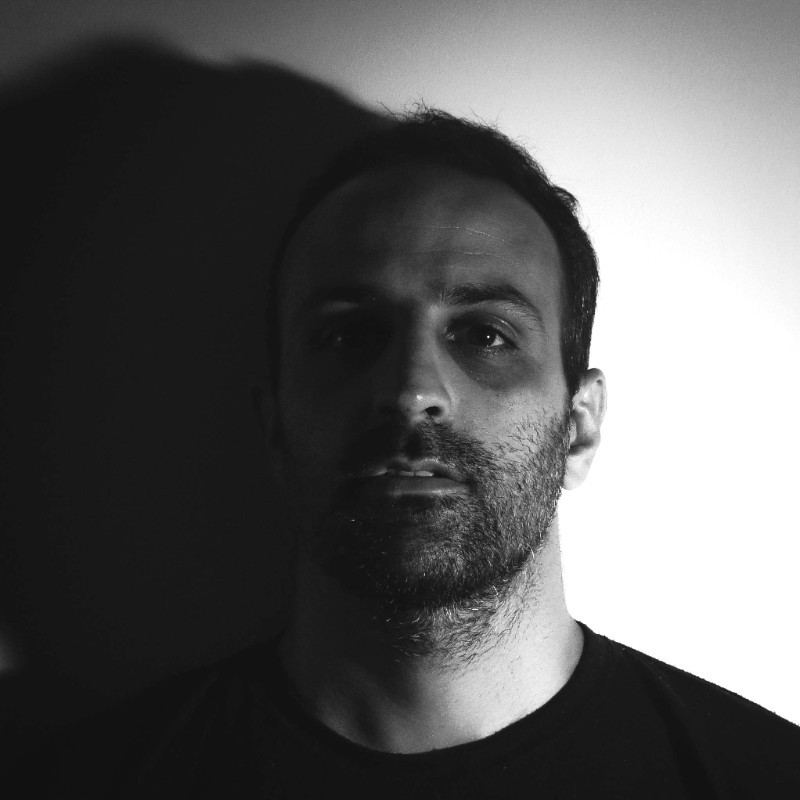
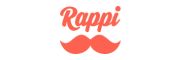