Automate with Ease: Exploring Laravel’s Task Scheduler
One of the significant advantages of using Laravel, a popular PHP framework, is its built-in task scheduling system. This feature simplifies the process of scheduling tasks that need to be run periodically, such as sending out emails or cleaning up databases. With Laravel, you can automate these repetitive tasks without requiring additional tools or services.
To fully harness the power of this system, you may choose to hire Laravel developers. These professionals, proficient in Laravel’s tools and services, can maximize the framework’s potential in automating tasks, leading to more streamlined operations in your projects.
Introduction to Task Scheduling in Laravel
Laravel’s task scheduler is wrapped around the cron syntax to provide a more user-friendly and expressive way of defining when tasks should run. It’s part of the Laravel ecosystem and thus integrated seamlessly into the Laravel project.
With Laravel’s task scheduler, instead of manually entering cron job entries on the server, you can define all your application’s tasks within Laravel itself and the only cron job entry you need on your server is a call to the Laravel command `schedule:run`.
To set up the Laravel task scheduler, you’ll need to add the following Cron entry to your server:
```bash * * * * * cd /path-to-your-project && php artisan schedule:run >> /dev/null 2>&1 ```
This Cron will call Laravel’s command scheduler every minute. When the `schedule:run` command is executed, Laravel will evaluate your scheduled tasks and run the tasks that are due.
Scheduling Tasks in Laravel
Tasks are defined in the `app/Console/Kernel.php` file’s `schedule` method. This method is called when the `artisan command schedule:run` is triggered. Here’s an example of a simple task scheduling:
```php protected function schedule(Schedule $schedule) { $schedule->command('inspire')->hourly(); } ```
In this example, the ‘inspire’ command is set to run every hour. Laravel has a wide range of scheduling options available, allowing for tasks to be run at various intervals, from every minute to once yearly.
Task Scheduling Examples
Let’s go through some practical examples to understand how Laravel Task Scheduling can help automate repetitive tasks.
Example 1: Database Cleanup
Consider a situation where your application receives a large number of sign-ups, and you want to remove unverified users after 48 hours. You can automate this task by scheduling a command that will run every day to perform this cleanup.
```php protected function schedule(Schedule $schedule) { $schedule->command('user:cleanup')->daily(); } ```
The `user:cleanup` command will be your custom command that will perform the desired task. To create this command, use the `make:command` artisan command:
```bash php artisan make:command UserCleanup --command=user:cleanup ```
This command will create a new command class in the `app/Console/Commands` directory. You can then implement the `handle` method in this class:
```php public function handle() { User::where('verified', 0) ->where('created_at', '<', Carbon::now()->subHours(48)) ->delete(); $this->info('Unverified users have been cleaned up.'); } ```
Example 2: Sending Daily Reports
Another common use case is sending daily reports by email. For this task, you can define a custom command that generates and sends the report:
```php protected function schedule(Schedule $schedule) { $schedule->command('email:dailyReport')->dailyAt('22:00'); } ```
You would create the `email:dailyReport` command as above. In the command’s handle method, you would implement the logic for generating the report and sending the email.
Example 3: Health Check
You might want to regularly ping a URL to ensure your application is running smoothly. Here, you can use the `ping` method provided by Laravel.
```php protected function schedule(Schedule $schedule) { $schedule->job(new PerformHealthCheck)->everyFiveMinutes()->ping('http://your-webhook-url'); } ```
The `PerformHealthCheck` job will perform the health check, and the URL will be pinged every five minutes.
Conclusion
Laravel’s task scheduler is an excellent tool for automating and managing repetitive tasks. It offers the flexibility of defining tasks within your Laravel code and scheduling them with a friendly, expressive syntax. By leveraging this feature, you can keep your application’s routine tasks well-managed and organized.
Hiring Laravel developers who are adept in utilizing such powerful features can greatly help your business in automating numerous tasks, thus saving time and boosting efficiency in your Laravel projects. Remember, the key to mastering Laravel’s task scheduler isn’t only about understanding its expressive syntax and flexibility, but also effectively implementing it in real-world applications.
Table of Contents
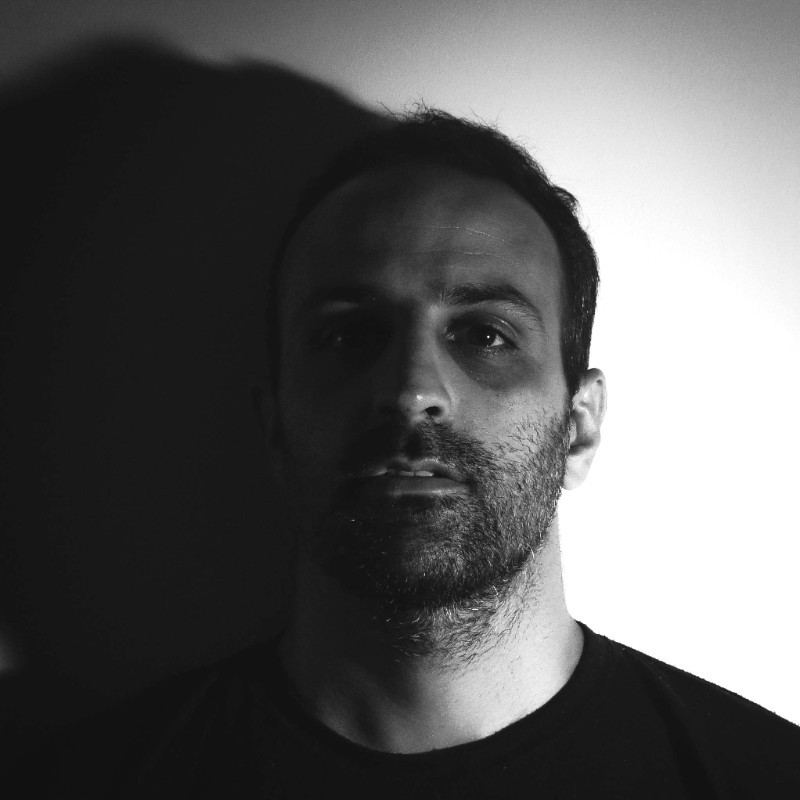
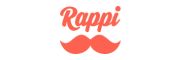