Test-Driven Development with Laravel: Building Robust Applications
In the world of web development, ensuring the quality and stability of applications is crucial. This is where Test-Driven Development (TDD) comes into play. Laravel, a popular PHP framework, provides excellent support for implementing TDD practices. By following TDD principles, developers can build robust applications with confidence.
In this blog post, we will dive deep into Test-Driven Development with Laravel, exploring its benefits, best practices, and real-life examples. We will cover the fundamental concepts of TDD and walk through the process of building a Laravel application using TDD. So, let’s get started!
Understanding Test-Driven Development (TDD)
Test-Driven Development is an iterative development approach that focuses on writing tests before writing the production code. TDD follows a simple three-step cycle:
- Write a test: Start by writing a failing test that describes the desired behavior or functionality.
- Make the test pass: Write the minimum amount of code necessary to make the test pass.
- Refactor the code: Once the test passes, refactor the code to improve its design without changing its behavior.
By following this cycle, TDD ensures that the code is always accompanied by tests, which act as a safety net against regressions and provide documentation for the expected behavior.
Setting Up Laravel for Test-Driven Development
To begin with, let’s set up Laravel for TDD. Assuming you have Laravel installed, you can create a new Laravel project using the following command:
bash $ laravel new myproject
Once the project is created, navigate to the project directory:
bash $ cd myproject
Laravel provides a built-in testing framework called PHPUnit. Make sure you have PHPUnit installed globally by running the following command:
bash $ composer global require phpunit/phpunit
Once PHPUnit is installed, Laravel is ready for TDD.
Writing Your First Test in Laravel
In Laravel, tests are stored in the tests directory. By default, Laravel provides a tests/Feature directory for feature tests and a tests/Unit directory for unit tests.
Let’s create a simple test for our application. In the tests/Feature directory, create a new file called ExampleTest.php:
php <?php namespace Tests\Feature; use Illuminate\Foundation\Testing\RefreshDatabase; use Illuminate\Foundation\Testing\WithFaker; use Tests\TestCase; class ExampleTest extends TestCase { /** * A basic test example. * * @return void */ public function testBasicTest() { $response = $this->get('/'); $response->assertStatus(200); } }
In the above example, we have created a basic test that asserts that a GET request to the root URL (/) returns a status code of 200.
Running Tests in Laravel
To run the tests in Laravel, we can use the php artisan test command. Run the following command in the terminal:
bash $ php artisan test
Laravel will automatically discover and execute the tests within the tests directory.
Implementing Test-Driven Development Workflow
Now that we have seen how to write and run tests in Laravel, let’s explore the TDD workflow. We will follow the TDD cycle mentioned earlier to build a simple feature.
Step 1: Write a failing test:
Create a new test in tests/Feature directory named UserTest.php:
php <?php namespace Tests\Feature; use App\Models\User; use Illuminate\Foundation\Testing\RefreshDatabase; use Illuminate\Foundation\Testing\WithFaker; use Tests\TestCase; class UserTest extends TestCase { use RefreshDatabase; public function testUserCreation() { $this->withoutExceptionHandling(); $userCount = User::count(); $userData = [ 'name' => 'John Doe', 'email' => 'john@example.com', 'password' => bcrypt('password'), ]; $response = $this->post('/users', $userData); $response->assertStatus(201); $this->assertCount($userCount + 1, User::all()); } }
In the above test, we simulate creating a new user and assert that the response status is 201 (indicating a successful creation) and that the total user count increases by 1.
Step 2: Make the test pass:
To make the test pass, we need to create the necessary routes and controllers in Laravel. Let’s update the routes/web.php file:
php use App\Http\Controllers\UserController; Route::post('/users', [UserController::class, 'store']);
Next, create a new controller UserController by running the following command:
bash $ php artisan make:controller UserController
Open the UserController.php file and add the store method:
php <?php namespace App\Http\Controllers; use App\Models\User; use Illuminate\Http\Request; class UserController extends Controller { public function store(Request $request) { $user = User::create([ 'name' => $request->input('name'), 'email' => $request->input('email'), 'password' => bcrypt($request->input('password')), ]); return response()->json($user, 201); } }
In the above code, we create a new user using the input data from the request and return a JSON response with the user data and a status of 201.
Step 3: Refactor the code:
At this point, our test is passing, but it’s essential to refactor the code to improve its design and maintainability. Refactoring involves making the code cleaner, more modular, and easier to understand without altering its behavior.
Conclusion
Test-Driven Development is a powerful methodology for building robust and stable applications in Laravel. By writing tests first, developers gain confidence in their code, improve code quality, and reduce the likelihood of introducing bugs and regressions.
In this blog post, we explored the fundamentals of Test-Driven Development with Laravel, from setting up Laravel for TDD to implementing a TDD workflow. We covered writing tests, running tests, and following the TDD cycle, ensuring the quality and reliability of our Laravel applications.
By adopting Test-Driven Development practices, you can elevate your Laravel development skills and create applications that are well-tested, maintainable, and future-proof.
So, embrace Test-Driven Development and start building robust applications with Laravel today!
Table of Contents
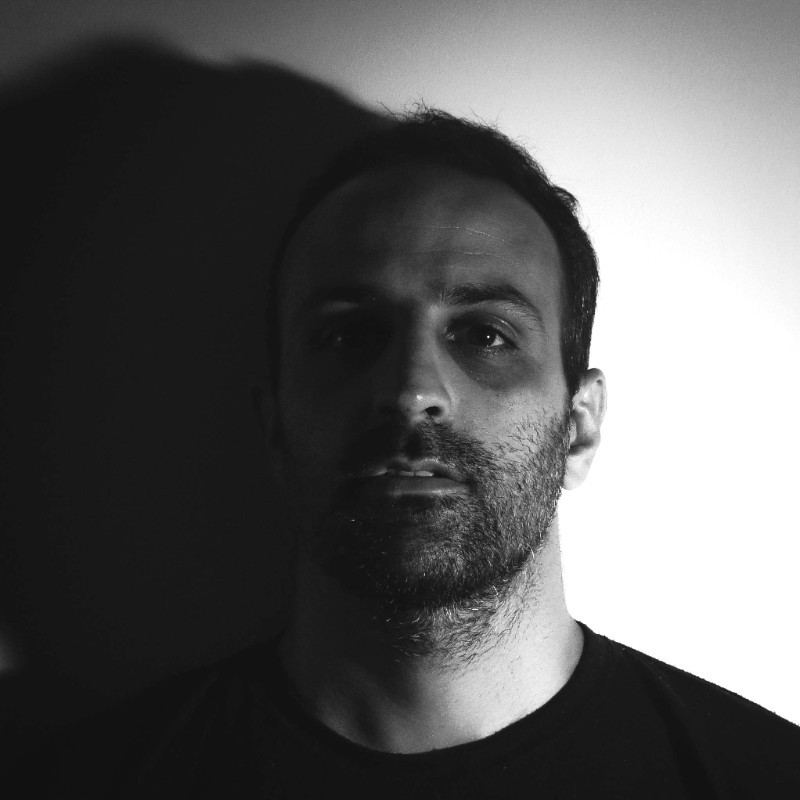
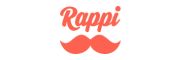