Laravel Testing: Ensuring Quality with PHPUnit
In the world of web development, delivering high-quality software is not just a preference, but a necessity. Users expect seamless experiences, and any glitches or bugs can lead to frustration and lost business opportunities. Laravel, a popular PHP framework, recognizes the significance of robust testing to ensure a reliable application. PHPUnit, a widely-used testing framework for PHP, plays a pivotal role in achieving this goal by allowing developers to write and execute tests efficiently. In this article, we’ll delve into the importance of testing in Laravel, explore PHPUnit’s features, and provide you with essential tips and code samples to master testing in your Laravel projects.
Table of Contents
1. The Significance of Testing in Laravel
1.1. Ensuring Reliability
Testing is not just a phase that comes after the development process; it’s an integral part of the entire software development lifecycle. In Laravel, testing enables you to catch bugs and issues early in the development process, reducing the likelihood of major problems surfacing in production. By identifying and addressing issues during testing, you can enhance the reliability and stability of your application.
1.2. Supporting Continuous Integration
Modern development practices, such as continuous integration and continuous delivery (CI/CD), demand robust testing. Automated tests, including unit tests, integration tests, and acceptance tests, are essential components of a successful CI/CD pipeline. Laravel’s integration with PHPUnit allows you to automate these tests, ensuring that code changes do not inadvertently introduce regressions or errors.
1.3. Introducing PHPUnit for Laravel Testing
PHPUnit is a unit testing framework specifically designed for PHP applications. It enables developers to write test cases and assertions to validate the functionality of their code. Laravel comes with PHPUnit out of the box, making it seamless to integrate testing into your development workflow.
1.4. Writing Your First Test
Let’s get started with a simple example of a test in a Laravel application. Assume you have a basic “Task” model, and you want to test whether creating a task works as expected. Here’s how you can write a test case for it:
php namespace Tests\Unit; use Tests\TestCase; use App\Models\Task; class TaskTest extends TestCase { public function testTaskCreation() { $taskData = [ 'title' => 'Sample Task', 'description' => 'This is a test task', ]; $task = Task::create($taskData); $this->assertEquals('Sample Task', $task->title); $this->assertEquals('This is a test task', $task->description); } }
In this example, we create a test case class TaskTest that extends Laravel’s TestCase. The testTaskCreation method defines the test itself. We create a sample task using the Task::create() method and then use PHPUnit’s assertion methods to validate whether the task’s properties match the expected values.
1.5. Running Tests
To run your tests, you can use the php artisan test command. Laravel will discover and execute all the test cases defined in your application. Running this command will provide you with detailed information about which tests passed and which ones failed.
2. Best Practices for Laravel Testing with PHPUnit
2.1. Test Naming Convention
Clear and descriptive test names enhance the readability of your test suite. Following a naming convention, such as starting test methods with “test” and using camel case, can make your tests more organized and understandable.
2.2. Isolation and Dependency Injection
When writing tests, it’s essential to isolate the code you’re testing from external dependencies. Laravel’s dependency injection makes it easier to replace real implementations with mock objects for testing purposes. Mocking objects helps create controlled testing environments, allowing you to focus solely on the functionality you’re testing.
2.3. Data Seeding for Consistency
Consistency is crucial in testing. Laravel provides a convenient way to seed your database with test data using seeders. This ensures that your tests are conducted on a consistent dataset, preventing unexpected behavior due to varying data.
2.4. Testing HTTP Endpoints
Laravel offers an expressive and elegant way to test HTTP endpoints. You can simulate requests and responses using the actingAs method to authenticate users and the get, post, put, and delete methods to interact with endpoints. This enables you to validate the behavior of your routes and controllers.
2.5. Assertions for Various Scenarios
PHPUnit provides a rich set of assertion methods to cover various scenarios. Some commonly used assertions include:
- assertEquals: Checks if two values are equal.
- assertNotNull: Verifies that a value is not null.
- assertContains: Checks if a value is present in an array.
- expectException: Validates that a specific exception is thrown.
Conclusion
Effective testing is a cornerstone of building reliable and maintainable Laravel applications. With PHPUnit’s integration into the Laravel ecosystem, developers have a powerful tool to ensure the quality and stability of their code. By following best practices, writing meaningful tests, and using Laravel’s testing features, you can create a robust test suite that empowers you to make changes with confidence, knowing that your application remains solid. So, embrace testing as an integral part of your development process and unlock the potential to deliver exceptional user experiences through top-notch Laravel applications.
Table of Contents
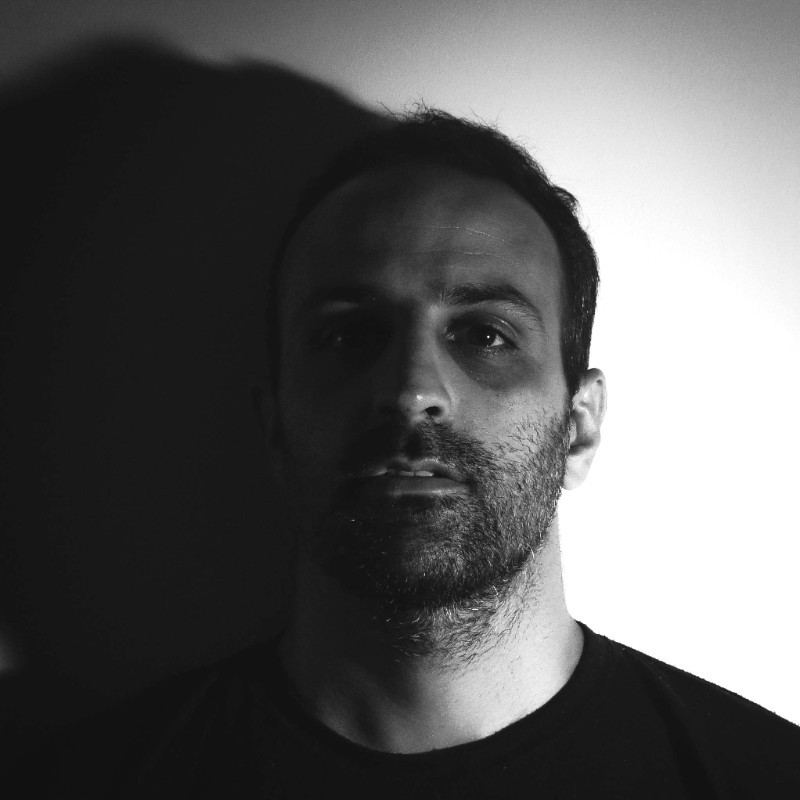
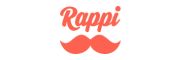