Level Up Your Laravel Toolkit: The Power of Interactive Tinker
Laravel, the popular PHP framework, is known for its robustness and a myriad of tools that facilitate smooth and rapid application development. One such tool that often doesn’t get the attention it deserves is Laravel Tinker. Tinker is an interactive shell that allows developers to interact with their Laravel application directly from the command line. In this blog post, we’ll delve into the workings of Laravel Tinker, highlighting its features and offering some illustrative examples.
1. What is Laravel Tinker?
At its core, Tinker provides an REPL (Read-Evaluate-Print Loop) interface for Laravel. Based on the powerful PsySH console, Tinker lets you interactively experiment with your application’s data, logic, and overall structure. This means that without going through the web interface or writing a temporary route or controller, you can quickly test, debug, or even run database queries.
2. Installing and Accessing Tinker
If you’ve installed Laravel via Composer, chances are Tinker is already included, as it’s a part of the standard Laravel framework. To start Tinker, open your terminal or command prompt, navigate to your Laravel project directory, and simply enter:
```bash php artisan tinker ```
You’ll then be greeted by a Tinker shell, ready for your commands.
2.1 Basic Usage
Let’s start with some elementary operations:
- Evaluating Expressions
In the Tinker shell, you can perform simple arithmetic:
```php >>> 5 + 3 => 8 ```
- Working with Laravel Eloquent Models
Suppose you have a model named `User`. You can retrieve all users with:
```php >>> App\Models\User::all(); ```
To fetch the first user:
```php >>> $user = App\Models\User::first(); => App\Models\User {#1234 id: 1, name: "John Doe", ...} ```
- Creating New Records
Using Tinker, you can also add new entries:
```php >>> $user = new App\Models\User; >>> $user->name = "Jane Smith"; >>> $user->email = "jane@example.com"; >>> $user->password = bcrypt('password'); >>> $user->save(); ```
2.2 Advanced Features
- Magic Where Queries
Tinker supports Eloquent’s dynamic where clauses. For instance, to retrieve users with the name ‘John Doe’:
```php >>> App\Models\User::whereName('John Doe')->get(); ```
- Tinker with Relationships
Assume `User` has a `hasMany` relationship with a model named `Post`. You can access the posts of a user:
```php >>> $user = App\Models\User::first(); >>> $user->posts; ```
- Calling Application Methods
If you have a method in your Laravel application, for instance, a helper function `formatDate($date)`, you can call it directly:
```php >>> formatDate(now()); => "2023-09-20" ```
- Listing Class Methods and Properties
To see the methods and properties available for an object, use the `ls` command:
```php >>> ls App\Models\User ```
2.3 Tinker Collections
Laravel’s powerful collection methods are also available in Tinker. For example, to get the list of all user emails:
```php >>> App\Models\User::all()->pluck('email'); ```
2.4 Tinker and Events
You can also work with and fire events in Tinker:
```php >>> event(new App\Events\UserRegistered($user)); ```
2.5 Exiting Tinker
To leave the Tinker environment, simply type `exit` or press `CTRL + D`.
Conclusion
Laravel Tinker is a potent tool in a developer’s arsenal, bridging the gap between rapid testing and application development. Whether it’s for debugging, data exploration, or even quick tasks like database seeding, Tinker is invaluable. As with all Laravel features, the more you use it, the more you’ll appreciate its depth and capabilities. Happy tinkering!
Table of Contents
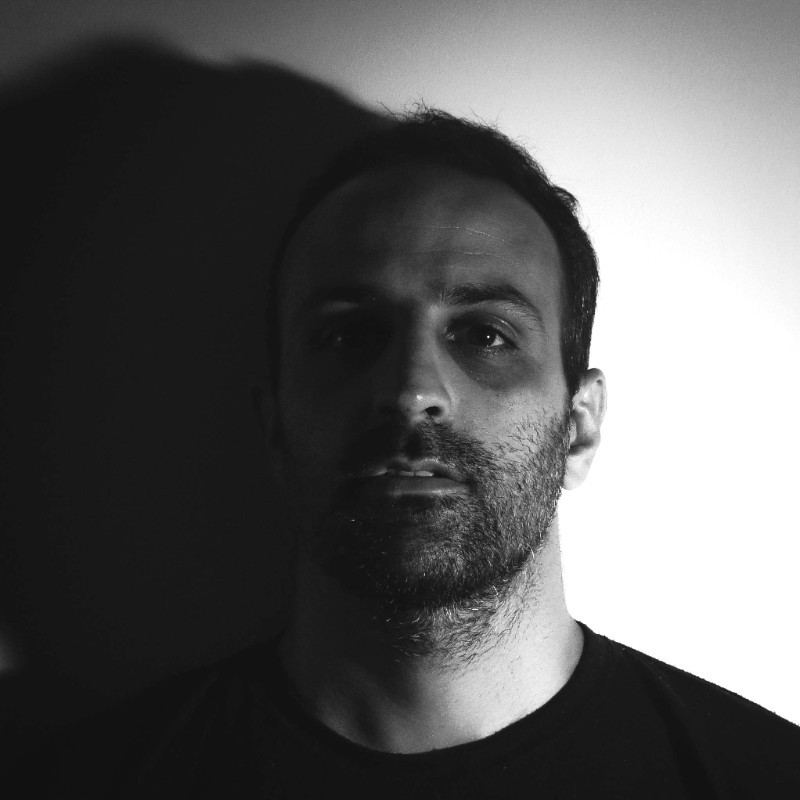
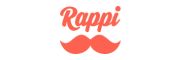