How to validate form input in Laravel?
Validating form input in Laravel is like having a safety net to catch any mistakes or missing information users might submit—it’s all about ensuring that the data your application receives is accurate, complete, and safe to use. Let’s explore a human-friendly guide on how to validate form input in Laravel:
When a user submits a form on your Laravel-powered website, it’s crucial to validate the data they’ve entered before processing it further. Laravel provides a convenient and powerful validation feature that makes it easy to define rules for each form field and automatically handle validation errors.
To validate form input in Laravel, you’ll first need to define the validation rules for your form fields. Laravel’s validation rules cover a wide range of scenarios, such as required fields, email addresses, numeric values, and more.
For example, suppose you have a registration form with fields for the user’s name, email, and password. You might define the validation rules for this form like this:
php $validatedData = $request->validate([ 'name' => 'required', 'email' => 'required|email|unique:users', 'password' => 'required|min:8', ]);
In this example, we’re using Laravel’s validation rules to specify that the name and email fields are required, the email field must be a valid email ad
dress, and the email field must be unique in the users table. Additionally, the password field is required and must be at least 8 characters long.
Once you’ve defined the validation rules, Laravel will automatically validate the incoming request data against these rules. If any validation rules fail, Laravel will redirect the user back to the form with the validation errors flashed to the session, allowing you to display them to the user and prompt them to correct their input.
To display validation errors in your form, you can use Laravel’s Blade templating engine to loop through the errors and display them next to the corresponding form fields. For example:
php @if ($errors->any()) <div class="alert alert-danger"> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif
By validating form input in Laravel, you can ensure that your application receives accurate and safe data from users, reducing the risk of errors, security vulnerabilities, and data inconsistencies. With Laravel’s intuitive validation syntax and powerful features, you can create robust and user-friendly forms that enhance the usability and reliability of your web applications.
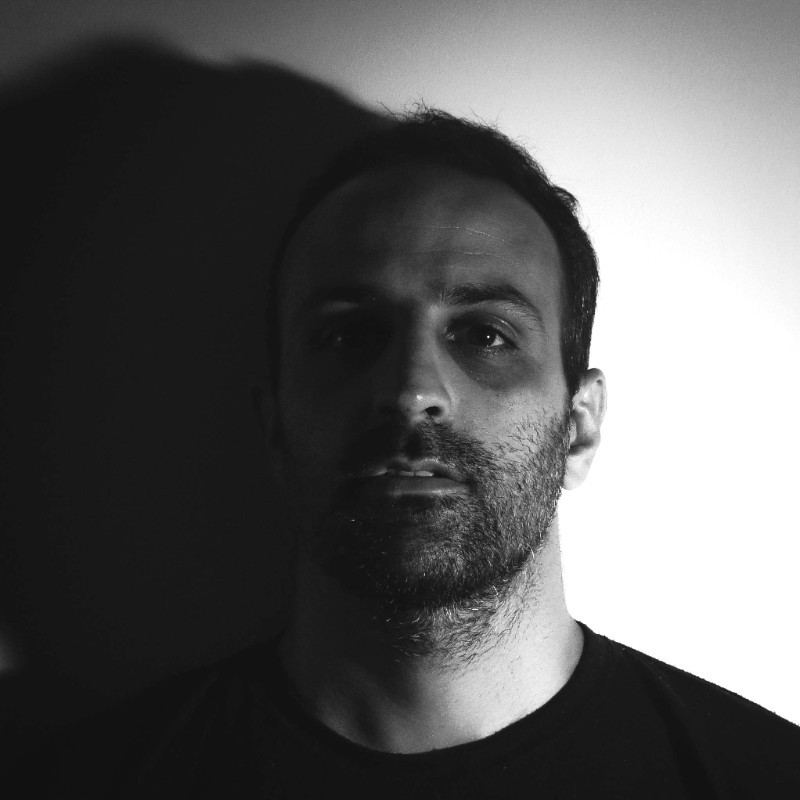
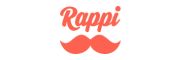