Laravel and Vue.js Integration: Building Modern Web Apps
In today’s fast-paced digital world, building modern web applications requires a combination of backend frameworks and frontend libraries. Laravel, a powerful PHP framework, and Vue.js, a progressive JavaScript framework, form a formidable duo for developing dynamic and interactive web apps. This blog post will guide you through the process of integrating Laravel and Vue.js, showcasing their synergy and enabling you to build modern web applications efficiently.
Why Laravel and Vue.js?
Laravel is a feature-rich PHP framework renowned for its elegant syntax, robust routing system, and comprehensive documentation. On the other hand, Vue.js is a progressive JavaScript framework that excels in creating interactive user interfaces. Integrating Laravel and Vue.js combines the best of both worlds, enabling developers to build modern web applications with ease.
Setting up Laravel and Vue.js
To start our integration journey, we need to set up Laravel and Vue.js in our development environment. First, ensure PHP and Composer are installed. Then, create a new Laravel project using the Laravel installer or Composer. Next, set up a Vue.js project using the Vue CLI. We’ll configure Laravel to serve the Vue.js application as a single-page application (SPA) for a seamless user experience.
Code Sample:
javascript // Laravel Installation composer create-project laravel/laravel my-project // Vue.js Installation npm install -g @vue/cli vue create my-project // Laravel Configuration // Edit routes/web.php Route::get('/{any}', function () { return view('app'); })->where('any', '.*'); // Edit resources/views/app.blade.php <!DOCTYPE html> <html> <head> <title>Laravel Vue Integration</title> <link href="{{ asset('css/app.css') }}" rel="stylesheet"> </head> <body> <div id="app"></div> <script src="{{ asset('js/app.js') }}"></script> </body> </html>
Creating a Laravel API
With the initial setup complete, we can now focus on building a Laravel API to handle data requests from our Vue.js frontend. Laravel provides a straightforward way to create RESTful APIs using its built-in routing and controller system. We’ll create routes and controllers to handle CRUD (Create, Read, Update, Delete) operations on our application’s resources.
Code Sample:
php // Laravel API Routes // Edit routes/api.php Route::apiResource('posts', 'PostController'); // Laravel API Controller php artisan make:controller PostController // Edit app/Http/Controllers/PostController.php public function index() { return Post::all(); } // Implement other CRUD methods (show, store, update, destroy) in the controller
Building the Vue.js Frontend
Now that our API is in place, let’s move on to building the frontend using Vue.js. Vue.js provides a component-based architecture that promotes reusability and modularity. We’ll create Vue components to display, create, update, and delete resources using the Laravel API we built. We’ll also incorporate Vue Router for navigation and Vuex for state management.
Code Sample:
php // Vue.js Component <template> <div> <h1>Posts</h1> <ul> <li v-for="post in posts" :key="post.id">{{ post.title }}</li> </ul> </div> </template> <script> export default { data() { return { posts: [], }; }, mounted() { axios.get('/api/posts').then((response) => { this.posts = response.data; }); }, }; </script>
Connecting Laravel and Vue.js
To establish communication between Laravel and Vue.js, we’ll use Axios, a promise-based HTTP client, on the frontend to send requests to the Laravel API. Axios provides an intuitive way to make HTTP requests and handle responses asynchronously. We’ll configure Axios to send requests to our Laravel API endpoints and handle the returned data in our Vue.js components.
Code Sample:
javascript // Install Axios in Vue.js project npm install axios // Vue.js Component <script> import axios from 'axios'; export default { // ... methods: { fetchPosts() { axios.get('/api/posts').then((response) => { this.posts = response.data; }); }, }, mounted() { this.fetchPosts(); }, }; </script>
Implementing CRUD Operations
With the foundation set, we can now dive into implementing CRUD operations using Laravel and Vue.js. We’ll enhance our Vue.js components to allow users to create, read, update, and delete resources through the Laravel API. By leveraging Laravel’s Eloquent ORM, we can easily perform database operations and update the Vue.js frontend accordingly.
Code Sample:
php // Vue.js Component <template> <div> <h1>Posts</h1> <ul> <li v-for="post in posts" :key="post.id"> {{ post.title }} <button @click="deletePost(post.id)">Delete</button> </li> </ul> <form @submit.prevent="createPost"> <input type="text" v-model="newPost.title" placeholder="Enter title" /> <button type="submit">Create</button> </form> </div> </template> <script> import axios from 'axios'; export default { // ... methods: { // ... createPost() { axios.post('/api/posts', this.newPost).then((response) => { this.posts.push(response.data); this.newPost = { title: '' }; }); }, deletePost(postId) { axios.delete(`/api/posts/${postId}`).then(() => { this.posts = this.posts.filter((post) => post.id !== postId); }); }, }, }; </script>
Authentication and Authorization
Building secure web applications often requires implementing authentication and authorization. Laravel offers a comprehensive authentication system that seamlessly integrates with Vue.js. We can use Laravel’s built-in features such as middleware and policies to control access to certain routes and actions based on user roles and permissions. Additionally, Vue.js can leverage Laravel’s authentication APIs to authenticate users and manage user sessions.
Code Sample:
javascript // Laravel Authentication Routes // Edit routes/api.php Route::post('login', 'AuthController@login'); Route::post('logout', 'AuthController@logout'); Route::post('register', 'AuthController@register'); // Laravel Authentication Controller php artisan make:controller AuthController // Edit app/Http/Controllers/AuthController.php public function login(Request $request) { $credentials = $request->only('email', 'password'); if (Auth::attempt($credentials)) { $user = $request->user(); $token = $user->createToken('authToken')->accessToken; return response()->json(['token' => $token], 200); } return response()->json(['error' => 'Unauthorized'], 401); } // Vue.js Authentication Component <template> <div> <form @submit.prevent="login"> <input type="email" v-model="credentials.email" placeholder="Email" /> <input type="password" v-model="credentials.password" placeholder="Password" /> <button type="submit">Login</button> </form> </div> </template> <script> import axios from 'axios'; export default { // ... data() { return { credentials: { email: '', password: '', }, }; }, methods: { login() { axios.post('/api/login', this.credentials).then((response) => { const token = response.data.token; // Store the token in local storage or Vuex store for future requests // Redirect the user to the protected routes }); }, }, }; </script>
Optimizing Performance with Laravel and Vue.js
To ensure our web application delivers optimal performance, we can apply various techniques and strategies. Laravel offers caching mechanisms, eager loading, and query optimization techniques. On the Vue.js side, we can implement lazy loading, code splitting, and utilize Vue’s reactive system efficiently. These optimizations contribute to faster page load times and a smoother user experience.
Deployment Considerations
When it comes to deploying Laravel and Vue.js applications, we have multiple options. We can host our backend on platforms like Heroku, AWS, or DigitalOcean, and use a content delivery network (CDN) to serve our frontend assets. Additionally, we need to configure the appropriate environment variables, set up secure HTTPS connections, and consider strategies like caching, asset optimization, and monitoring to ensure a reliable and scalable deployment.
Conclusion
Laravel and Vue.js integration provides developers with a powerful toolkit to build modern web applications efficiently. By combining Laravel’s robust backend capabilities and Vue.js’s dynamic frontend components, we can create seamless and engaging user experiences. From setting up the development environment to implementing CRUD operations, authentication, and optimization techniques, this blog post has provided you with a solid foundation to leverage the synergy between Laravel and Vue.js for your next web application project.
Table of Contents
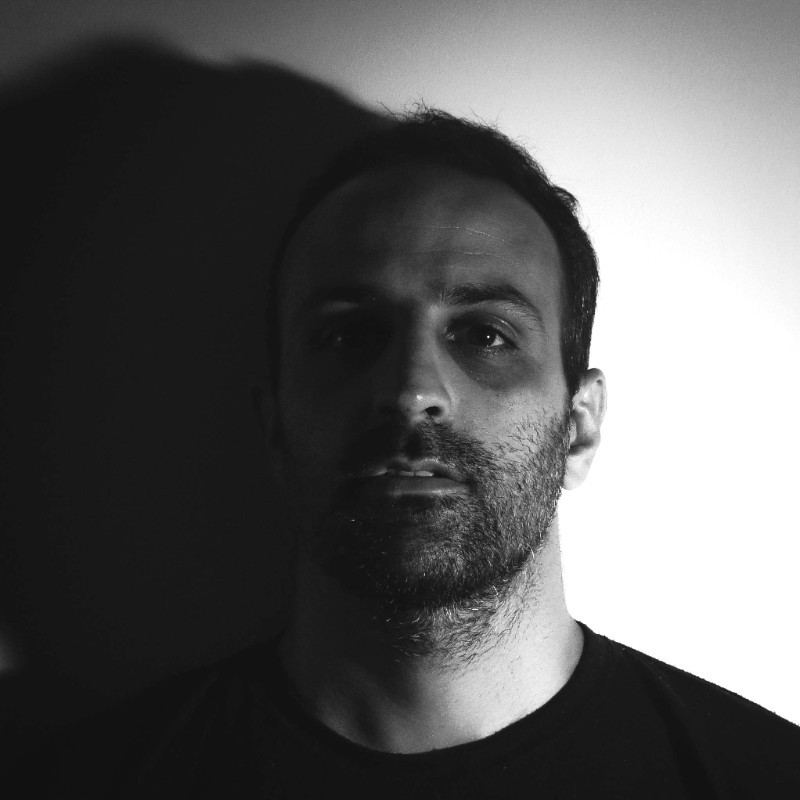
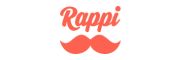