Meteor and D3.js: Creating Interactive Data Visualizations
In today’s data-driven world, the ability to understand and interpret data is crucial for making informed decisions. Data visualizations provide a powerful way to convey complex information in a clear and interactive manner. Meteor, a full-stack JavaScript framework, and D3.js, a powerful data visualization library, offer a perfect combination to build dynamic and interactive data visualizations that can be updated in real-time. In this blog post, we’ll explore how to harness the power of Meteor and D3.js to create stunning data visualizations that engage your users and bring your data to life.
1. Prerequisites
Before diving into creating interactive data visualizations, it’s essential to have a basic understanding of JavaScript, HTML, and CSS. Familiarity with Meteor and D3.js will be beneficial but not mandatory, as we’ll walk through the essential concepts step by step.
2. Setting Up the Project
Let’s start by setting up our Meteor project. If you don’t have Meteor installed, you can follow the installation instructions from the official Meteor website (https://www.meteor.com/install).
1. Create a new Meteor project:
bash meteor create data-visualization-app cd data-visualization-app
2. Remove the default files:
bash rm data-visualization-app.css rm data-visualization-app.html rm data-visualization-app.js
3. Install D3.js:
bash meteor add d3js:d3
Now that we have our project structure ready, let’s move on to the next step.
3. Loading Data with Meteor
The foundation of any data visualization is, of course, the data itself. Meteor makes it incredibly easy to handle real-time data. For the sake of this tutorial, we’ll use a simple example of visualizing the number of website visits over time.
3.1. Add some sample data:
In the server/main.js file, insert the following code:
javascript import { Meteor } from 'meteor/meteor'; Meteor.startup(() => { // Sample data const websiteVisitsData = [ { date: new Date('2023-07-01'), visits: 200 }, { date: new Date('2023-07-02'), visits: 320 }, { date: new Date('2023-07-03'), visits: 280 }, // Add more data here ]; // Publish the data to the client Meteor.publish('websiteVisits', function () { return websiteVisitsData; }); });
3.2. Subscribe to the data on the client:
In the client/main.js file, add the following code:
javascript import { Meteor } from 'meteor/meteor'; Meteor.startup(() => { // Subscribe to the data on the client Meteor.subscribe('websiteVisits'); });
With this setup, the client now has access to the website visits data published by the server. Next, let’s proceed to visualize this data using D3.js.
4. Creating a Simple Line Chart
A line chart is an excellent choice for visualizing data that changes over time. In this section, we’ll build a basic line chart using D3.js to display the website visits data we’ve loaded with Meteor.
4.1. Create the HTML template:
In the data-visualization-app.html file, add the following code:
html <head> <title>Meteor and D3.js Data Visualization</title> </head> <body> <div id="chart-container"> <svg id="line-chart"></svg> </div> </body>
4.2. Define the CSS styles:
In the data-visualization-app.css file, add the following code:
css #chart-container { width: 100%; max-width: 800px; margin: 0 auto; }
4.3. Create the JavaScript code:
In the client/main.js file, add the following code to draw the line chart:
javascript import { Template } from 'meteor/templating'; import { ReactiveVar } from 'meteor/reactive-var'; import { Meteor } from 'meteor/meteor'; import { D3 } from 'meteor/d3js:d3'; Template.body.onCreated(function () { this.websiteVisitsData = new ReactiveVar([]); this.autorun(() => { // Get the website visits data from the subscription const data = Meteor.subscribe('websiteVisits').ready() ? WebsiteVisits.find().fetch() : []; // Update the reactive variable with the latest data this.websiteVisitsData.set(data); }); }); Template.body.onRendered(function () { this.autorun(() => { // Get the website visits data from the reactive variable const data = Template.instance().websiteVisitsData.get(); // Access the SVG element const svg = D3.select('#line-chart'); // Set the dimensions for the chart const margin = { top: 20, right: 20, bottom: 30, left: 50 }; const width = +svg.attr('width') - margin.left - margin.right; const height = +svg.attr('height') - margin.top - margin.bottom; // Parse the dates and format the time scale const parseTime = D3.timeParse('%Y-%m-%d'); const x = D3.scaleTime().range([0, width]); const y = D3.scaleLinear().range([height, 0]); // Define the line function const line = D3.line() .x((d) => x(d.date)) .y((d) => y(d.visits)); // Append a group for the chart const g = svg.append('g') .attr('transform', `translate(${margin.left},${margin.top})`); // Format the data data.forEach((d) => { d.date = parseTime(d.date); d.visits = +d.visits; }); // Set the domains for the scales x.domain(D3.extent(data, (d) => d.date)); y.domain(D3.extent(data, (d) => d.visits)); // Add the X axis g.append('g') .attr('transform', `translate(0,${height})`) .call(D3.axisBottom(x)); // Add the Y axis g.append('g') .call(D3.axisLeft(y)); // Add the line path g.append('path') .datum(data) .attr('fill', 'none') .attr('stroke', 'steelblue') .attr('stroke-width', 2) .attr('d', line); }); });
With this code, the line chart will be drawn based on the website visits data retrieved from the Meteor subscription. The chart will automatically update whenever new data is published to the client, enabling real-time data visualization.
5. Adding Interactivity
Interactivity is key to engaging data visualizations. D3.js provides powerful tools to enhance user interactions. Let’s improve our line chart to include some interactivity, such as tooltips and the ability to pan and zoom.
5.1. Adding Tooltips:
Tooltips provide additional information when hovering over data points on the chart. Let’s integrate tooltips into our line chart.
javascript // ... (previous code) // Append a div for the tooltip const tooltip = D3.select('body').append('div') .attr('class', 'tooltip') .style('opacity', 0); // ... (previous code) // Add the line path g.append('path') .datum(data) .attr('fill', 'none') .attr('stroke', 'steelblue') .attr('stroke-width', 2) .attr('d', line) .on('mouseover', function (event, d) { // Show the tooltip on mouseover tooltip.transition() .duration(200) .style('opacity', 0.9); tooltip.html(`<strong>Date:</strong> ${d.date.toDateString()}<br><strong>Visits:</strong> ${d.visits}`) .style('left', `${event.pageX}px`) .style('top', `${event.pageY - 28}px`); }) .on('mouseout', function () { // Hide the tooltip on mouseout tooltip.transition() .duration(500) .style('opacity', 0); }); // ... (remaining code)
By adding these few lines of code, the chart will now display tooltips when hovering over data points, providing users with more context and details.
5.2. Enabling Pan and Zoom:
Pan and zoom functionality allows users to explore data in more detail. Let’s enable this feature in our line chart.
javascript // ... (previous code) // Append a clip path to the SVG svg.append('defs').append('clipPath') .attr('id', 'clip') .append('rect') .attr('width', width) .attr('height', height); // Append a rect to capture zoom events svg.append('rect') .attr('class', 'zoom') .attr('width', width) .attr('height', height) .attr('transform', `translate(${margin.left},${margin.top})`) .call(D3.zoom() .scaleExtent([1, 8]) .on('zoom', zoom) ); // Add the line path g.append('path') .datum(data) .attr('fill', 'none') .attr('stroke', 'steelblue') .attr('stroke-width', 2) .attr('d', line) .attr('clip-path', 'url(#clip)'); // ... (remaining code) // Zoom function function zoom(event) { const newX = event.transform.rescaleX(x); const newY = event.transform.rescaleY(y); // Update the axes with the new scale g.select('.x-axis').call(D3.axisBottom(newX)); g.select('.y-axis').call(D3.axisLeft(newY)); // Update the line path with the new scale g.select('.line') .attr('d', line.x((d) => newX(d.date)).y((d) => newY(d.visits))); } // ... (remaining code)
With the above code, users can now pan and zoom in the line chart to explore data across different time ranges effectively.
Conclusion
In this tutorial, we explored how to create interactive data visualizations using Meteor and D3.js. By combining the real-time data capabilities of Meteor with the versatile visualization options of D3.js, you can build engaging and interactive data visualizations that empower your users to gain deeper insights into your data. Whether you’re presenting website analytics, financial trends, or any other form of data, Meteor and D3.js offer a robust and dynamic solution to showcase your information effectively.
Remember, this is just the beginning of your journey into the world of data visualization. Experiment with different chart types, explore more advanced D3.js features, and integrate additional interactions to create even more compelling and informative visualizations. Happy coding!
Table of Contents
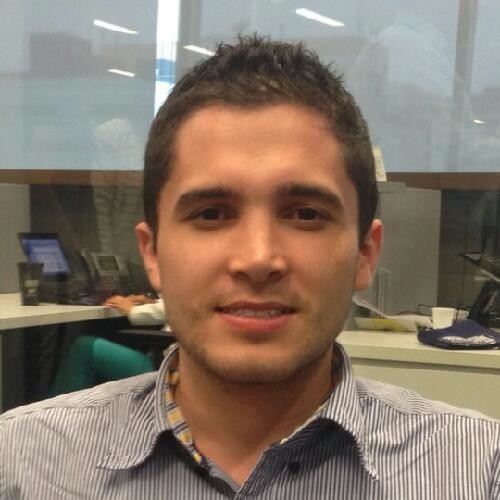
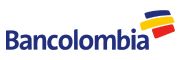