Meteor and React: Combining the Best of Both Worlds
In the fast-paced world of web development, staying ahead of the curve is crucial. Developers are constantly seeking ways to build applications that are not only efficient and reliable but also offer a seamless user experience. Meteor and React, two popular technologies in the JavaScript ecosystem, have emerged as powerful contenders in this realm. Combining the best of both worlds, developers can leverage the strengths of Meteor’s real-time capabilities and React’s component-based architecture to build cutting-edge applications that deliver on all fronts.
1. Understanding Meteor
1.1 What is Meteor?
Meteor, also known as Meteor.js, is an open-source JavaScript platform used for building web and mobile applications. Released in 2012, Meteor quickly gained popularity due to its ease of use and full-stack capabilities. One of the key features that set Meteor apart is its reactivity model, which enables real-time data synchronization between the client and the server. This means that any changes made to the data on the server are automatically reflected on the client, without the need for manual updates.
1.2 Advantages of Meteor
- Full-Stack Integration: Meteor provides a complete development stack, including the front-end, back-end, and database. This integration simplifies the development process, allowing developers to focus on building features rather than managing different components.
- Real-Time Communication: Meteor’s reactivity model facilitates real-time communication, making it ideal for applications that require instant updates and collaboration features.
- Hot Code Reload: With Meteor, developers can witness changes instantly as they code, thanks to its built-in hot code reload feature. This accelerates the development process and streamlines debugging.
- Large Community and Packages: Meteor has a vibrant community and a vast ecosystem of packages, which means developers can easily find solutions to common problems and extend the functionality of their applications.
2. Exploring React
2.1 What is React?
React, developed and maintained by Facebook, is a popular JavaScript library for building user interfaces. Since its release in 2013, React has revolutionized the way developers approach front-end development. Its component-based architecture allows developers to create reusable and modular UI components, resulting in more maintainable codebases and improved developer productivity.
2.2 Advantages of React
- Component-Based Architecture: React’s component-based approach promotes code reusability, making it easier to manage complex UI elements by breaking them down into smaller, manageable components.
- Virtual DOM: React’s Virtual DOM efficiently updates and renders only the components that have changed, reducing unnecessary re-rendering and boosting performance.
- JSX – JavaScript XML: React’s JSX syntax enables developers to write UI components using a familiar HTML-like syntax, making it more intuitive for front-end developers to transition to React.
- Thriving Ecosystem: With a large community and extensive third-party libraries, React offers a wealth of resources, tools, and solutions that enhance development capabilities.
3. The Power of Combining Meteor and React
3.1 Building Real-Time Applications
Meteor’s real-time capabilities and React’s component-based architecture complement each other perfectly when building real-time applications. Meteor’s reactivity model allows changes to propagate instantly to connected clients, while React’s Virtual DOM efficiently updates the UI components affected by these changes. This combination results in a smooth and responsive user experience, particularly crucial for collaborative applications like chat platforms, collaborative document editors, and real-time analytics dashboards.
Let’s take a look at a simple example of a real-time voting application using Meteor and React:
jsx // VoteApp.jsx import React, { useState } from 'react'; import { useTracker } from 'meteor/react-meteor-data'; import { Polls } from '/imports/api/polls'; const VoteApp = () => { const [selectedOption, setSelectedOption] = useState(null); const polls = useTracker(() => Polls.find().fetch()); const handleVote = (pollId) => { Meteor.call('polls.vote', pollId, selectedOption); }; return ( <div> <h1>Real-Time Voting App</h1> {polls.map((poll) => ( <div key={poll._id}> <h3>{poll.question}</h3> <ul> {poll.options.map((option, index) => ( <li key={index}> <input type="radio" name={`poll_${poll._id}`} value={index} onChange={() => setSelectedOption(index)} /> {option} </li> ))} </ul> <button onClick={() => handleVote(poll._id)}>Vote</button> </div> ))} </div> ); }; export default VoteApp;
In this example, we’re using Meteor’s real-time capabilities to synchronize voting data across connected clients. React’s component-based structure allows us to create reusable poll components and efficiently update the user interface when users cast their votes.
3.2 Seamless Isomorphic Rendering
Isomorphic rendering, also known as universal rendering, is a technique that allows the same codebase to render on both the server and the client. Meteor and React are well-suited for isomorphic rendering, enabling developers to improve application performance and search engine optimization (SEO) by rendering initial views on the server and later seamlessly transitioning to client-side rendering.
To achieve isomorphic rendering, we can use the ReactDOMServer and ReactDOM APIs in React. Here’s a simplified example of a server-rendered React component using Meteor:
jsx // Server-side rendering with Meteor and React import React from 'react'; import { renderToString } from 'react-dom/server'; const App = () => { return <h1>Hello, Server-Side Rendering!</h1>; }; // Render the component to a string const appString = renderToString(<App />); // Send the HTML string to the client WebApp.rawConnectHandlers.use((req, res) => { res.writeHead(200); res.end(`<!DOCTYPE html><html><head></head><body>${appString}</body></html>`); });
By combining Meteor’s server-side capabilities and React’s component rendering, we achieve a seamless user experience with faster initial page loads and improved SEO.
4. Best Practices and Tips
4.1 Manage State with React Hooks
With React hooks, managing state in functional components becomes more straightforward and less error-prone. Leverage hooks like useState and useEffect to manage local component state and handle side effects respectively.
jsx import React, { useState, useEffect } from 'react'; const Counter = () => { const [count, setCount] = useState(0); useEffect(() => { // Do something after every render }, [count]); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount((prevCount) => prevCount + 1)}>Increment</button> </div> ); };
4.2 Optimize React Component Rendering
React’s Virtual DOM makes rendering efficient, but it’s essential to avoid unnecessary re-renders. Use React.memo or shouldComponentUpdate to prevent re-rendering when the component’s props haven’t changed.
jsx import React from 'react'; const MemoizedComponent = React.memo(({ data }) => { // Component rendering logic }); class PureComponent extends React.PureComponent { render() { // Component rendering logic } }
4.3 Modularize Meteor Methods
To maintain a clean and organized codebase, modularize Meteor methods by keeping them in separate files based on their functionality. Use the import and export statements to structure your methods and reduce complexity.
javascript // imports/api/polls.js import { Meteor } from 'meteor/meteor'; import { Mongo } from 'meteor/mongo'; export const Polls = new Mongo.Collection('polls'); Meteor.methods({ 'polls.vote'(pollId, selectedOption) { // Voting logic here }, });
Conclusion
In conclusion, the combination of Meteor and React brings together the best of both worlds in web development. Meteor’s real-time capabilities enable developers to build dynamic and collaborative applications, while React’s component-based architecture ensures maintainable and efficient user interfaces. Moreover, their synergy allows for seamless isomorphic rendering and enhances the overall development experience.
By harnessing the power of Meteor and React, developers can create modern and responsive web applications that delight users and stand out in the competitive landscape. Whether you’re building a real-time chat application or a sophisticated data visualization dashboard, the Meteor-React duo is a potent toolkit that empowers developers to innovate and create cutting-edge experiences.
So, embrace the amalgamation of Meteor and React and take your web development prowess to new heights! Happy coding!
Table of Contents
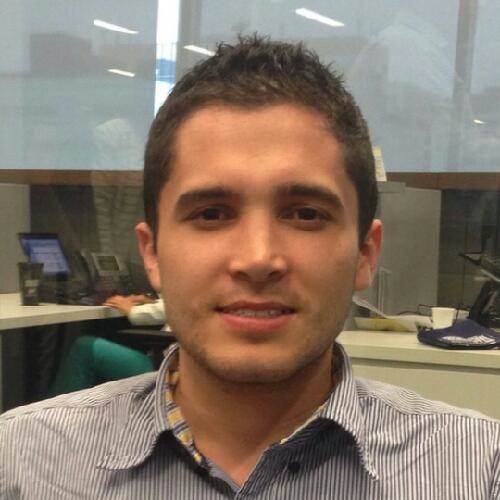
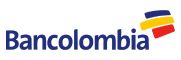