Meteor Authentication and Authorization: Implementing User Management
In today’s digital landscape, ensuring the security of user data and controlling access to different parts of an application is of utmost importance. Meteor, a full-stack JavaScript framework, offers powerful tools to implement user authentication and authorization seamlessly. This blog will guide you through the process of setting up user management with authentication and authorization in your Meteor application.
1. Understanding Authentication and Authorization:
Before diving into the implementation details, it’s essential to understand the difference between authentication and authorization. Authentication is the process of verifying a user’s identity to ensure they are who they claim to be. On the other hand, authorization involves granting specific privileges and permissions to authenticated users based on their roles or other criteria.
2. Setting Up a New Meteor Project:
First, make sure you have Node.js and Meteor installed on your system. Create a new Meteor project using the following command:
bash meteor create my-app cd my-app
3. Implementing User Registration and Login:
To begin user management, we need to add packages that provide user accounts functionality. Meteor simplifies this process with the accounts-base and accounts-password packages. Run the following commands to add these packages:
bash meteor add accounts-base meteor add accounts-password
Now, let’s create the user registration and login forms. In your client/main.html file, add the following code:
html <template name="signup"> <form> <input type="email" name="email" placeholder="Email"> <input type="password" name="password" placeholder="Password"> <button type="submit">Sign Up</button> </form> </template> <template name="login"> <form> <input type="email" name="email" placeholder="Email"> <input type="password" name="password" placeholder="Password"> <button type="submit">Login</button> </form> </template>
Now, let’s implement the corresponding event handlers in client/main.js:
javascript Template.signup.events({ 'submit form': function (event) { event.preventDefault(); const email = event.target.email.value; const password = event.target.password.value; Accounts.createUser({ email, password }, function (error) { if (error) { // Handle registration error console.error(error); } else { // Redirect or show success message } }); }, }); Template.login.events({ 'submit form': function (event) { event.preventDefault(); const email = event.target.email.value; const password = event.target.password.value; Meteor.loginWithPassword(email, password, function (error) { if (error) { // Handle login error console.error(error); } else { // Redirect or show success message } }); }, });
With these implementations, you now have user registration and login forms integrated into your Meteor application.
4. Securing Routes and Implementing Authorization:
In most applications, certain routes or pages should only be accessible to authenticated users. To enforce this, we can use route-based authorization. First, let’s install the necessary package:
bash meteor add kadira:flow-router
Next, we need to create a route that requires authentication to access. In client/main.html, add the following template:
html <template name="dashboard"> <h1>Welcome to the Dashboard!</h1> </template>
In client/main.js, add the corresponding route and the necessary authorization check:
javascript import { FlowRouter } from 'meteor/kadira:flow-router'; FlowRouter.route('/dashboard', { name: 'dashboard', action: function () { if (Meteor.userId()) { BlazeLayout.render('mainLayout', { main: 'dashboard' }); } else { FlowRouter.go('/'); } }, });
In the code above, we check if a user is authenticated using Meteor.userId(). If the user is authenticated, we render the dashboard template; otherwise, we redirect the user to the homepage.
5. Handling User Roles and Permissions:
In many applications, different users may have different roles and permissions, which affect their access to certain features or data. We can achieve this in Meteor using the alanning:roles package. Let’s install it:
bash meteor add alanning:roles
Now, let’s define some roles and assign them to users. In server/main.js, add the following code:
javascript import { Roles } from 'meteor/alanning:roles'; const adminEmail = 'admin@example.com'; const moderatorEmail = 'moderator@example.com'; Meteor.startup(function () { // Create admin user if not exists if (Meteor.users.find({ 'emails.address': adminEmail }).count() === 0) { const adminId = Accounts.createUser({ email: adminEmail, password: 'adminpassword', }); Roles.addUsersToRoles(adminId, ['admin']); } // Create moderator user if not exists if (Meteor.users.find({ 'emails.address': moderatorEmail }).count() === 0) { const moderatorId = Accounts.createUser({ email: moderatorEmail, password: 'moderatorpassword', }); Roles.addUsersToRoles(moderatorId, ['moderator']); } });
In the code above, we create admin and moderator users during startup if they don’t exist and assign them their respective roles using Roles.addUsersToRoles.
Now that we have roles assigned, we can implement authorization checks based on user roles. Modify the FlowRouter.route in client/main.js as follows:
javascript FlowRouter.route('/dashboard', { name: 'dashboard', action: function () { if (Meteor.userId()) { if (Roles.userIsInRole(Meteor.userId(), 'admin')) { BlazeLayout.render('mainLayout', { main: 'dashboard' }); } else { FlowRouter.go('/'); } } else { FlowRouter.go('/'); } }, });
In this code, we use Roles.userIsInRole to check if the current user has the ‘admin’ role before rendering the dashboard template.
6. Additional Security Considerations:
In addition to the steps mentioned above, here are some other security considerations to keep in mind:
- Password Policies: Enforce strong password policies to prevent weak passwords.
- Account Lockouts: Implement account lockout mechanisms to prevent brute-force attacks.
- Two-Factor Authentication (2FA): Offer 2FA to enhance user account security.
- Secure Data Transmission: Ensure all data is transmitted over HTTPS to protect it from eavesdropping.
- Input Validation: Validate and sanitize all user inputs to prevent injection attacks.
Conclusion
In this blog, we explored how to implement robust user management with authentication and authorization in your Meteor application. By following these steps and best security practices, you can create a secure environment for your users while providing a seamless and personalized experience based on their roles and permissions. Remember, security is an ongoing process, so stay updated with the latest security trends and continually improve your application’s defenses against potential threats. Happy coding!
Table of Contents
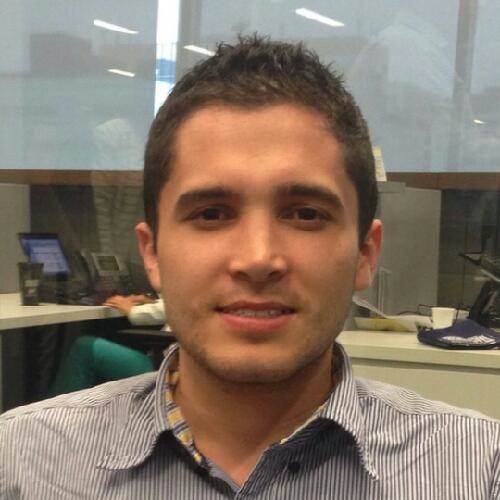
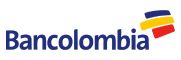