Building a Meteor Blogging Platform: From Idea to Deployment
The world of blogging has seen tremendous growth in recent years, with millions of bloggers sharing their thoughts, experiences, and expertise on various topics. If you’ve ever considered starting your own blogging platform, Meteor is an excellent choice for its rapid development capabilities and real-time features. In this tutorial, we’ll guide you through the process of building a Meteor-based blogging platform, step by step, from the initial idea to deployment. By the end, you’ll have a fully functional blogging platform where users can publish articles and engage with each other in real-time.
Prerequisites
Before we dive into the development process, let’s ensure you have the necessary prerequisites in place:
Basic Knowledge of JavaScript: Familiarity with JavaScript is essential, as Meteor is built on Node.js and heavily relies on JavaScript.
Meteor: Make sure you have Meteor installed on your system. If not, head to the Meteor website (https://www.meteor.com/) and follow the installation instructions for your OS.
Text Editor: Choose a code editor of your choice; Visual Studio Code, Atom, or Sublime Text are popular options.
Setting Up the Project
Let’s start by creating a new Meteor project for our blogging platform. Open your terminal and enter the following command:
bash meteor create meteor-blog-platform
This command will create a new Meteor project named meteor-blog-platform.
Next, navigate to the project directory:
bash cd meteor-blog-platform
Now, let’s remove the default files that we won’t need for our blogging platform:
bash rm meteor-blog-platform.css meteor-blog-platform.html meteor-blog-platform.js
Designing the Database
Our blogging platform will require a database to store articles, user information, and comments. For this tutorial, we’ll use MongoDB, the default database supported by Meteor.
Step 1: Install MongoDB
If you don’t have MongoDB installed, you can download and install it from the official website (https://www.mongodb.com/try/download/community). Alternatively, you can use a cloud-based MongoDB service.
Step 2: Define Data Models
Let’s define the data models for our blogging platform. We need three collections: Articles, Users, and Comments.
In your project directory, create a new folder called collections:
bash mkdir collections
Inside the collections folder, create three files: articles.js, users.js, and comments.js. In each of these files, define the data models using Meteor’s Mongo.Collection:
collections/articles.js
javascript import { Mongo } from 'meteor/mongo'; export const Articles = new Mongo.Collection('articles'); Articles.allow({ insert: () => false, update: () => false, remove: () => false, }); Articles.deny({ insert: () => true, update: () => true, remove: () => true, }); // Define the article schema here
collections/users.js
javascript import { Mongo } from 'meteor/mongo'; export const Users = new Mongo.Collection('users'); Users.allow({ insert: () => false, update: () => false, remove: () => false, }); Users.deny({ insert: () => true, update: () => true, remove: () => true, }); // Define the user schema here
collections/comments.js
javascript import { Mongo } from 'meteor/mongo'; export const Comments = new Mongo.Collection('comments'); Comments.allow({ insert: () => false, update: () => false, remove: () => false, }); Comments.deny({ insert: () => true, update: () => true, remove: () => true, }); // Define the comment schema here
Replace the comments with your desired schema for each collection. For example, the article schema might include fields like title, content, author, and createdAt.
Creating the User Interface
Next, we’ll work on creating the user interface for our blogging platform. We’ll use Meteor’s built-in Blaze template engine to achieve this.
Step 1: Install Blaze
Meteor comes with Blaze by default, so you don’t need to install anything separately.
Step 2: Layout Template
In the root of your project directory, create a new file called main.html. This file will serve as the layout template for your blogging platform. Add the following content to it:
main.html
html <!DOCTYPE html> <html> <head> <title>Meteor Blog Platform</title> </head> <body> <header> <h1>Meteor Blogging Platform</h1> </header> <main> {{> yield}} </main> <footer> <p>© {{currentYear}} Meteor Blog Platform</p> </footer> </body> </html>
In this layout, we have a header, a main content area that will render the current template, and a footer displaying the current year.
Step 3: Home Template
Create a new file called home.html in your project directory:
home.html
html <template name="home"> <div> <h2>Welcome to Meteor Blog Platform</h2> <!-- Display the list of articles here --> </div> </template>
This template will be our home page, displaying a welcome message and the list of articles.
Step 4: Register Templates
Now that we’ve created the layout and home templates, let’s register them in our main.js file:
main.js
javascript import { Template } from 'meteor/templating'; import './main.html'; import './home.html'; Template.home.helpers({ currentYear() { return new Date().getFullYear(); }, });
This code imports the templates and registers the home template with a helper function to get the current year.
Implementing Functionality
So far, we have the basic structure in place. Now, let’s implement functionality to add, display, and edit articles.
Step 1: Adding Articles
We’ll start by allowing registered users to add articles to our platform.
collections/articles.js
javascript // ... Articles.allow({ insert: (userId, doc) => { // Allow inserting articles only if the user is logged in return !!userId; }, update: () => false, remove: () => false, }); // …
Now, let’s create a form to add articles in the home.html template:
home.html
html <template name="home"> <div> <h2>Welcome to Meteor Blog Platform</h2> {{#if currentUser}} <form> <input type="text" name="title" placeholder="Title"> <textarea name="content" placeholder="Content"></textarea> <button type="submit">Add Article</button> </form> {{/if}} <!-- Display the list of articles here --> </div> </template>
Step 2: Displaying Articles
To display the list of articles, we need to fetch the data from the Articles collection and iterate over it in the home template.
home.js
javascript import { Template } from 'meteor/templating'; import { Articles } from '../collections/articles'; import './home.html'; Template.home.helpers({ currentYear() { return new Date().getFullYear(); }, articles() { return Articles.find({}, { sort: { createdAt: -1 } }); }, }); Template.home.events({ 'submit form'(event) { event.preventDefault(); const target = event.target; const title = target.title.value; const content = target.content.value; // Insert the new article into the database Articles.insert({ title, content, createdAt: new Date(), author: Meteor.userId(), }); // Clear the form fields after submitting target.title.value = ''; target.content.value = ''; }, });
Step 3: User Authentication
We added the ability to add articles, but we only want authenticated users to have access to this functionality. Let’s add user authentication using Meteor’s accounts-ui package.
Step 3.1: Install accounts-ui
In your terminal, run the following command to install the accounts-ui package:
bash meteor add accounts-ui
Step 3.2: Import accounts-ui
In your main.js file, import the accounts-ui package to activate the user interface for login and registration:
main.js
javascript import 'meteor/accounts-ui';
Step 3.3: Display Login Buttons
Update the main.html file to display the login buttons in the header:
main.html
html <!DOCTYPE html> <html> <head> <title>Meteor Blog Platform</title> </head> <body> <header> <h1>Meteor Blogging Platform</h1> {{> loginButtons}} </header> <main> {{> yield}} </main> <footer> <p>© {{currentYear}} Meteor Blog Platform</p> </footer> </body> </html>
Now, the login buttons will be displayed in the header.
Step 4: Editing Articles
To allow authors to edit their articles, we’ll add an edit button next to each article. When clicked, it will open a form to edit the article’s title and content.
home.html
html <template name="home"> <div> <h2>Welcome to Meteor Blog Platform</h2> {{#if currentUser}} <form> <input type="text" name="title" placeholder="Title"> <textarea name="content" placeholder="Content"></textarea> <button type="submit">Add Article</button> </form> {{/if}} <ul> {{#each articles}} <li> <h3>{{title}}</h3> <p>{{content}}</p> {{#if isAuthor author}} <button class="edit">Edit</button> {{/if}} </li> {{/each}} </ul> </div> </template>
home.js
javascript // ... Template.home.helpers({ // ... isAuthor(author) { return author === Meteor.userId(); }, }); Template.home.events({ // ... 'click .edit'(event, instance) { const target = event.target; const articleId = $(target).data('id'); // Find the article in the database const article = Articles.findOne(articleId); // Update the form fields with the article data instance.$('input[name=title]').val(article.title); instance.$('textarea[name=content]').val(article.content); }, });
Step 5: Deploying the Blogging Platform
Now that you have built your Meteor blogging platform, it’s time to deploy it and make it accessible to the world. Meteor supports easy deployment through its built-in hosting service, Galaxy, or other platforms like Heroku and DigitalOcean.
Deploying with Meteor Galaxy
Meteor Galaxy is the official hosting service for Meteor applications. Follow these steps to deploy your blogging platform with Galaxy:
1. Create an account on Meteor Galaxy (https://www.meteor.com/galaxy).
2. Install the Meteor CLI if you haven’t already:
bash curl https://install.meteor.com/ | sh
3. Login to your Galaxy account using the Meteor CLI:
bash meteor login
4. Prepare your app for deployment:
bash meteor deploy your-blog-app-name.meteorapp.com
Replace your-blog-app-name with your desired application name.
5. Your application is now deployed! Visit your-app-name.meteorapp.com to access your blogging platform.
Congratulations! You’ve successfully built and deployed your own Meteor-based blogging platform from idea to deployment. Now, you can continue enhancing it with features like user profiles, categories, and more. Happy blogging!
Conclusion
In this tutorial, we took you on a journey to build a Meteor blogging platform from scratch. We covered setting up the project, designing the database, creating the user interface, implementing functionality, and finally deploying the platform for the world to see. You now have a solid foundation to continue exploring Meteor’s capabilities and customizing your blogging platform to meet your unique needs. Whether you want to run a personal blog or create a community of writers, Meteor provides a robust and real-time environment to make your blogging dreams come true. Happy coding!
Table of Contents
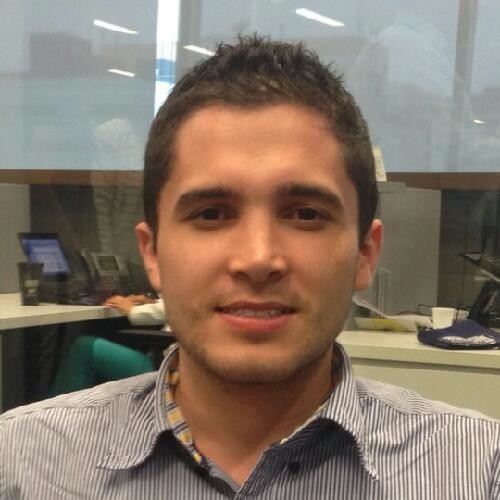
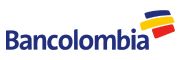