Meteor and GraphQL: Integrating the Power of Query Language
In today’s web development landscape, efficient data fetching and real-time updates have become crucial requirements for building modern applications. Meteor, a full-stack JavaScript framework, provides a robust platform for developing real-time web and mobile applications. When combined with GraphQL, a powerful query language for APIs, Meteor becomes even more powerful, enabling developers to build scalable and flexible applications. In this blog post, we will explore how Meteor and GraphQL can be integrated, highlighting the benefits and providing code samples to get you started.
1. Understanding GraphQL
1.1 What is GraphQL?
GraphQL is an open-source query language and runtime for APIs that was developed by Facebook. It provides a flexible and efficient approach to data fetching, allowing clients to request precisely the data they need from the server. Unlike traditional REST APIs where multiple requests may be required to fetch related data, GraphQL enables developers to fetch all required data in a single request. This reduces the amount of network traffic and improves performance.
1.2 Advantages of GraphQL
- Efficient data fetching: With GraphQL, clients can specify the exact data requirements, avoiding over-fetching or under-fetching of data. This granularity reduces the payload size and improves performance.
- Declarative syntax: GraphQL uses a declarative syntax, allowing clients to define the shape and structure of the response they expect. This approach provides more control to the clients and simplifies the development process.
- Strong typing system: GraphQL has a strong typing system that allows defining strict schemas. This ensures that the data returned from the server matches the expected structure, reducing runtime errors.
- Introspection: GraphQL provides introspection capabilities, allowing clients to query the schema and understand the available types and fields dynamically. This feature enables powerful tooling and documentation generation.
2. Introduction to Meteor
2.1 What is Meteor?
Meteor is a full-stack JavaScript framework that enables developers to build real-time web and mobile applications using a single codebase. It provides an opinionated structure for building applications by integrating frontend and backend seamlessly. Meteor uses a reactive data model, which means that any changes in the data are automatically propagated to the connected clients in real-time.
2.2 Key Features of Meteor
- Isomorphic code: Meteor allows developers to write isomorphic code, which can run on both the client and the server. This feature reduces code duplication and improves code sharing between the frontend and backend.
- Real-time updates: Meteor provides built-in support for real-time updates. Any changes made to the server-side data are automatically reflected in the connected clients, providing a seamless real-time experience.
- Data synchronization: Meteor automatically synchronizes data between the client and server using a mechanism called “Meteor Methods.” This simplifies data fetching and updating by abstracting away the complexities of network communication.
- Hot Code Push: Meteor supports Hot Code Push, which allows seamless updates to the running application without requiring the clients to reload the page or restart the app.
3. Integrating GraphQL with Meteor
3.1 Setting up a Meteor Project
To integrate GraphQL with Meteor, we first need to set up a Meteor project. Assuming you have Node.js and Meteor installed, follow these steps:
- Step 1: Create a new Meteor project
bash meteor create my-app
- Step 2: Navigate into the project directory
bash cd my-app
3.2 Adding GraphQL to Meteor
Next, we need to add GraphQL support to our Meteor project. We can do this by using the apollo package, which provides integration with GraphQL.
- Step 1: Add Apollo package to the project
bash meteor add apollo
- Step 2: Install required packages
bash meteor npm install @apollo/client graphql
3.3 Defining GraphQL Schema
Now that we have set up our Meteor project and added GraphQL support, let’s define the GraphQL schema. The schema defines the types, queries, mutations, and subscriptions available in our application.
javascript // imports/api/schema.graphql type Query { hello: String } type Mutation { greet(name: String!): String } schema { query: Query mutation: Mutation }
In the above example, we define a simple schema with a Query type that has a single field hello returning a String. We also define a Mutation type with a field greet that takes a name parameter and returns a String.
3.4 Writing GraphQL Resolvers
Resolvers are responsible for resolving the queries, mutations, and subscriptions defined in the schema. They contain the logic for fetching and manipulating the data.
javascript // imports/api/resolvers.js const resolvers = { Query: { hello: () => "Hello, GraphQL!", }, Mutation: { greet: (_, { name }) => `Hello, ${name}!`, }, }; export default resolvers;
In the above example, we define resolvers for the Query and Mutation types. The hello resolver returns the greeting message, and the greet resolver takes the name parameter and returns a personalized greeting.
3.5 Querying Data with GraphQL
To make use of the GraphQL API, we need to create a client-side connection and send queries to the server.
javascript // imports/startup/client/index.js import { ApolloClient, InMemoryCache, createHttpLink } from '@apollo/client'; const httpLink = createHttpLink({ uri: '/graphql', }); const client = new ApolloClient({ link: httpLink, cache: new InMemoryCache(), }); export default client;
In the above code, we create an Apollo client instance with an HTTP link that points to our GraphQL server.
To execute a query, we can use the useQuery hook provided by the @apollo/client package.
javascript // imports/ui/Hello.jsx import { useQuery, gql } from '@apollo/client'; const HELLO_QUERY = gql` query { hello } `; function Hello() { const { loading, error, data } = useQuery(HELLO_QUERY); if (loading) return <p>Loading...</p>; if (error) return <p>Error :(</p>; return <p>{data.hello}</p>; } export default Hello;
In the above code, we define a GraphQL query using the gql tag and then use the useQuery hook to fetch the data. The loading and error states are handled, and the result is rendered accordingly.
3.6 Mutations and Subscriptions with GraphQL
Similar to querying data, we can also perform mutations and subscribe to real-time updates using GraphQL in Meteor.
javascript // imports/ui/Greet.jsx import { useMutation, gql } from '@apollo/client'; const GREET_MUTATION = gql` mutation($name: String!) { greet(name: $name) } `; function Greet() { let input; const [greet, { data }] = useMutation(GREET_MUTATION); return ( <div> <form onSubmit={e => { e.preventDefault(); greet({ variables: { name: input.value } }); input.value = ''; }} > <input ref={node => { input = node; }} /> <button type="submit">Greet</button> </form> {data && <p>{data.greet}</p>} </div> ); } export default Greet;
In the above example, we define a GraphQL mutation to greet a person by name. We use the useMutation hook to execute the mutation, and the result is displayed when available.
For real-time updates, we can use GraphQL subscriptions to listen for changes and update the UI accordingly.
4. Real-time Updates with Meteor and GraphQL
4.1 Building Real-time Features with Meteor
Meteor’s reactivity model allows us to build real-time features effortlessly. By combining Meteor’s real-time capabilities with GraphQL subscriptions, we can achieve seamless real-time updates in our application.
4.2 Subscriptions in GraphQL
To use subscriptions in GraphQL, we need to define the subscription type in the schema and implement the corresponding resolver.
javascript // imports/api/schema.graphql type Subscription { newPost: Post } // imports/api/resolvers.js const resolvers = { // ... Subscription: { newPost: { subscribe: () => Posts.find(), }, }, };
In the above example, we define a subscription named newPost that listens for new posts. The resolver uses the Posts collection to retrieve the data and return it to the clients.
To consume the subscription on the client-side, we can use the useSubscription hook.
javascript // imports/ui/PostList.jsx import { useSubscription, gql } from '@apollo/client'; const NEW_POST_SUBSCRIPTION = gql` subscription { newPost { id title content } } `; function PostList() { const { data, loading } = useSubscription(NEW_POST_SUBSCRIPTION); if (loading) return <p>Loading...</p>; return ( <ul> {data.newPost.map(post => ( <li key={post.id}> <h3>{post.title}</h3> <p>{post.content}</p> </li> ))} </ul> ); } export default PostList;
In the above code, we define a GraphQL subscription using the gql tag and then use the useSubscription hook to receive real-time updates. The loading state is handled, and the new posts are rendered accordingly.
Conclusion
Integrating Meteor with GraphQL brings together the strengths of both technologies, allowing developers to build scalable and efficient applications with real-time capabilities. By leveraging the power of GraphQL’s query language and Meteor’s reactivity model, developers can deliver modern applications that meet the demands of today’s users. In this blog post, we explored the integration process, learned how to define schemas, write resolvers, and perform queries, mutations, and subscriptions using GraphQL within the Meteor framework. With this knowledge, you are now equipped to take advantage of the powerful combination of Meteor and GraphQL in your own projects. Happy coding!
Table of Contents
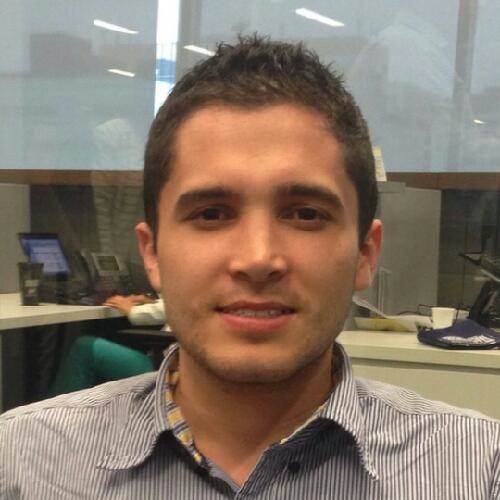
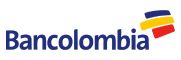