Meteor for Mobile Development: Creating Cross-platform Apps
In today’s fast-paced digital world, mobile applications have become an integral part of our lives. Whether it’s for communication, entertainment, or productivity, people rely on mobile apps for various purposes. As a developer, it’s crucial to be able to create mobile apps efficiently and effectively. This is where Meteor, a full-stack JavaScript framework, comes into play. In this blog post, we will explore how Meteor enables developers to create cross-platform apps effortlessly.
1. What is Meteor?
Meteor is an open-source JavaScript platform that allows developers to build web, mobile, and desktop applications using a single codebase. It follows the principles of reactive programming, enabling real-time data updates and synchronization between the server and client. With Meteor, you can write your entire application using JavaScript, from the front-end to the back-end, which streamlines the development process.
2. Benefits of Using Meteor for Mobile Development
2.1. Cross-platform Development
One of the significant advantages of Meteor is its ability to create cross-platform applications. You can develop apps for iOS, Android, and the web using the same codebase. This eliminates the need for separate development teams and reduces time and effort spent on maintaining multiple codebases. With Meteor, you can maximize code reuse and ensure consistent user experiences across different platforms.
2.2. Real-time Data Synchronization
Meteor’s real-time data synchronization is a game-changer for mobile app development. With its built-in reactivity, you can easily update and display data in real-time without manually refreshing the page. This feature is particularly useful for chat applications, collaborative tools, and any app that requires instant updates. Meteor takes care of the data synchronization, allowing you to focus on building great user experiences.
2.3. Full-stack JavaScript Development
With Meteor, you can write both the front-end and back-end of your application using JavaScript. This full-stack JavaScript development approach offers several benefits. First, it reduces the learning curve for developers who are already familiar with JavaScript. Second, it enables seamless communication between the client and server, as both sides use the same language. Finally, it simplifies the development process by eliminating the need to switch between different programming languages or frameworks.
3. Getting Started with Meteor for Mobile Development
To get started with Meteor for mobile development, follow these steps:
Step 1: Install Meteor
To install Meteor, visit the official Meteor website (https://www.meteor.com) and follow the installation instructions for your operating system. Meteor provides easy-to-use installation packages, making the setup process quick and straightforward.
Step 2: Create a New Meteor Project
Once Meteor is installed, open your terminal or command prompt and run the following command to create a new Meteor project:
bash meteor create my-app
This command creates a new directory named “my-app” and sets up the basic structure for your Meteor application.
Step 3: Run the Meteor App
Navigate to the project directory using the command prompt and run the following command to start the Meteor app:
bash cd my-app meteor
This command launches the Meteor development server and automatically opens your app in a web browser. You can now begin building your cross-platform mobile app using Meteor.
4. Building a Cross-platform App with Meteor
4.1. Creating the User Interface
In Meteor, user interfaces are built using a combination of HTML, CSS, and Blaze, a templating engine. Here’s an example of a simple login form in Meteor:
html <template name="loginForm"> <form> <input type="text" name="username" placeholder="Username"> <input type="password" name="password" placeholder="Password"> <button type="submit">Login</button> </form> </template>
In this example, we define a template named “loginForm” with input fields for the username and password, as well as a login button.
4.2. Implementing the Server-side Logic
Next, we need to implement the server-side logic to handle the login form submission and authentication. Here’s an example of how you can handle form submissions using Meteor’s server-side methods:
javascript import { Meteor } from 'meteor/meteor'; Meteor.methods({ 'loginUser'(username, password) { // Perform authentication logic here // ... }, });
In this code snippet, we define a server-side method named “loginUser” that takes the username and password as parameters. Inside this method, you can implement your authentication logic, such as verifying the user’s credentials against a database.
4.3. Adding Reactivity with Meteor’s Database
Meteor comes with a built-in MongoDB integration, which allows you to add real-time reactivity to your app. Here’s an example of how you can define a collection and use it in your app:
javascript import { Meteor } from 'meteor/meteor'; import { Mongo } from 'meteor/mongo'; export const Tasks = new Mongo.Collection('tasks'); if (Meteor.isServer) { Meteor.publish('tasks', function tasksPublication() { return Tasks.find(); }); }
In this example, we define a collection named “Tasks” and publish it on the server. On the client side, you can subscribe to this collection and use it in your templates to display and manipulate data.
Conclusion
Meteor provides a powerful and efficient framework for creating cross-platform mobile apps. With its cross-platform development capabilities, real-time data synchronization, and full-stack JavaScript approach, Meteor simplifies the mobile development process and enhances developer productivity. By following the steps outlined in this blog post and leveraging the code samples provided, you can start building your own cross-platform apps using Meteor today. Happy coding!
Table of Contents
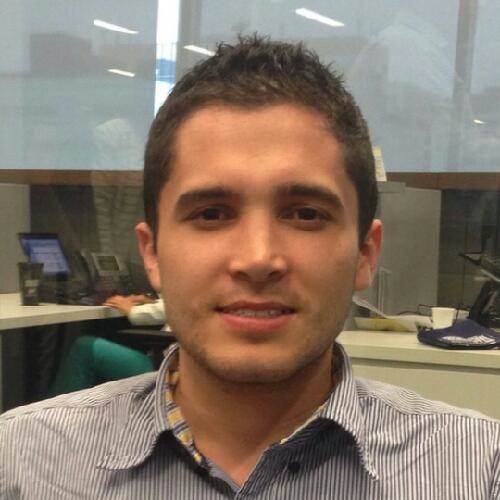
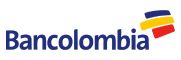