Meteor and MongoDB: Unleashing the Power of the Full Stack
In today’s fast-paced digital world, developers are constantly seeking efficient and robust tools to build powerful web applications. Meteor and MongoDB, when combined, form an unstoppable duo that enables full-stack development with ease. In this blog post, we will delve into the synergy between Meteor and MongoDB and explore how they empower developers to create scalable and real-time applications. From the basics to advanced techniques, we will cover everything you need to know to leverage the full potential of this dynamic duo.
1. What is Meteor?
Meteor is an open-source JavaScript framework that allows for rapid development of full-stack web applications. It provides a seamless, real-time, and reactive programming experience by combining the best aspects of front-end and back-end development into a single platform. With Meteor, developers can write code once and have it run on both the client and the server, enabling a smooth transition between the two.
2. What is MongoDB?
MongoDB is a document-oriented NoSQL database that provides high scalability and flexibility for modern applications. It stores data in a flexible, JSON-like format called BSON (Binary JSON), allowing for easy manipulation and retrieval. MongoDB’s dynamic schema enables developers to iterate quickly and adapt their data models as their applications evolve. Its real-time capabilities make it an excellent choice for building responsive and collaborative applications.
3. Setting up the Development Environment
Before we dive into the code, let’s set up our development environment to work with Meteor and MongoDB.
To get started, ensure that you have Node.js and npm (Node Package Manager) installed on your system. Then, follow these steps:
Install Meteor by running the following command in your terminal:
ruby $ curl https://install.meteor.com/ | sh
Install MongoDB by visiting the MongoDB website and following the installation instructions for your operating system.
Once MongoDB is installed, start the MongoDB service by running the following command:
ruby $ mongod
With the development environment set up, we can now start building our Meteor-MongoDB application.
4. Building a Real-Time Meteor App with MongoDB
Let’s create a simple real-time application using Meteor and MongoDB. We will build a chat application where users can send and receive messages in real-time.
4.1 Installing Dependencies:
First, let’s create a new Meteor application and install the necessary dependencies. Run the following commands in your terminal:
shell $ meteor create chat-app $ cd chat-app $ meteor npm install --save react react-dom $ meteor add session $ meteor add accounts-ui accounts-password
4.2 Creating a New Meteor App:
In the chat-app directory, create a new file called main.js and add the following code:
javascript import { Meteor } from 'meteor/meteor'; import { render } from 'react-dom'; import React from 'react'; import App from './App'; Meteor.startup(() => { render(<App />, document.getElementById('root')); });
4.3 Defining a MongoDB Collection:
Next, create a new file called messages.js in the imports/api directory and define a new MongoDB collection to store the messages:
javascript import { Mongo } from 'meteor/mongo'; export const Messages = new Mongo.Collection('messages');
4.4 Displaying Data in the User Interface:
Create a new file called App.js in the imports/ui directory and add the following code:
javascript import React, { Component } from 'react'; import { withTracker } from 'meteor/react-meteor-data'; import { Messages } from '../api/messages'; class App extends Component { handleSubmit(event) { event.preventDefault(); const text = this.textInput.value.trim(); Messages.insert({ text, createdAt: new Date(), }); this.textInput.value = ''; } render() { return ( <div> <header> <h1>Chat App</h1> </header> <ul> {this.props.messages.map((message) => ( <li key={message._id}>{message.text}</li> ))} </ul> <form onSubmit={this.handleSubmit.bind(this)}> <input type="text" ref={(input) => (this.textInput = input)} /> <button type="submit">Send</button> </form> </div> ); } } export default withTracker(() => { return { messages: Messages.find({}, { sort: { createdAt: -1 } }).fetch(), }; })(App);
4.5 Real-Time Updates with MongoDB Oplog:
Meteor leverages MongoDB’s oplog tailing feature to provide real-time updates to the client. It listens for changes in the database and automatically updates the user interface when new data is available.
To enable oplog tailing, run the following command in your terminal:
csharp $ meteor add mongo-livedata
With the code in place, start the Meteor application by running meteor in the terminal and visit http://localhost:3000 in your browser. You should see the chat application, where you can send and receive messages in real-time.
5. Scaling Meteor and MongoDB
As your application grows, you may need to scale both Meteor and MongoDB to handle increased traffic and data. Here are some techniques for scaling your application.
5.1 Clustering and Load Balancing:
Meteor allows you to cluster multiple instances of your application and balance the load across them using a load balancer. By distributing the workload, you can handle more concurrent users and provide better performance and availability. Tools like Nginx, HAProxy, or Meteor’s built-in load balancer can be used for load balancing.
5.2 Horizontal Scaling with MongoDB Replica Sets:
MongoDB offers horizontal scaling through replica sets. A replica set consists of multiple MongoDB instances that replicate data across them. This provides high availability and read scalability. By adding more replicas to your replica set, you can distribute the read workload and handle more read operations.
5.3 Vertical Scaling with MongoDB Sharding:
MongoDB sharding allows you to scale your write workload by distributing your data across multiple shards. Each shard is an independent MongoDB instance that stores a subset of the data. By adding more shards, you can handle more write operations and increase storage capacity.
6. Securing Your Meteor-MongoDB Application
Security is a crucial aspect of any application. Here are some best practices for securing your Meteor-MongoDB application:
6.1 Authentication and Authorization:
Implement user authentication to ensure that only authorized users can access your application. Meteor provides an authentication system out of the box, which you can customize to fit your application’s needs.
6.2 Data Validation and Sanitization:
Validate and sanitize user input on both the client and server to prevent common security vulnerabilities like SQL injection and cross-site scripting (XSS) attacks. Meteor’s built-in security features, such as method and publication restrictions, can help enforce data validation and security practices.
6.3 Implementing Role-Based Access Control:
Define roles and permissions to control what each user can access or modify within your application. Meteor’s role-based access control packages, like alanning:roles, can be used to implement fine-grained access control.
7. Best Practices for Performance Optimization
To ensure your Meteor-MongoDB application performs well, consider the following best practices:
7.1 Indexing and Query Optimization:
Create appropriate indexes on your MongoDB collections to improve query performance. Analyze the queries executed by your application and optimize them using appropriate query operators and projection techniques.
7.2 Data Caching:
Cache frequently accessed data to reduce the load on your MongoDB database. Tools like Redis or in-memory caching libraries can be used to implement data caching in Meteor.
7.3 Minimizing Network Latency:
Reduce the time taken to transfer data between the client and the server by minimizing network latency. Techniques like data compression, client-side caching, and optimizing resource loading can significantly improve your application’s performance.
Conclusion
Meteor and MongoDB together provide a powerful full-stack development platform. With Meteor’s real-time capabilities and MongoDB’s scalability, developers can build modern and responsive applications with ease. By following best practices for security, scalability, and performance optimization, you can unleash the full potential of the Meteor-MongoDB duo and deliver exceptional user experiences. So, start exploring the power of the full stack today and build amazing applications with Meteor and MongoDB!
Table of Contents
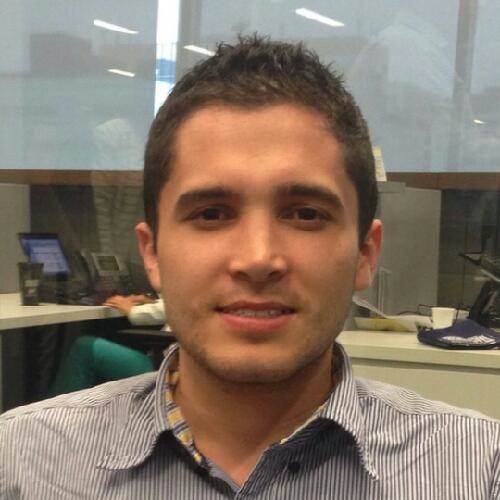
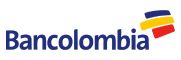