Optimizing Meteor Performance: Tips and Techniques
Meteor is a powerful and efficient full-stack JavaScript framework that allows developers to build real-time web applications with ease. However, as your app grows in complexity, you may start noticing performance bottlenecks that hinder its responsiveness. In this blog, we will explore essential tips and techniques to optimize your Meteor application for better performance.
1. Minimize Data Transfers
Sending excessive data over the network can significantly impact the performance of your Meteor app. Minimizing data transfers can be achieved through the following strategies:
1.1 Use Proper Publications and Subscriptions
Take advantage of Meteor’s publication and subscription mechanism to control the data sent to the client. Be selective in what data you publish and subscribe to, and avoid unnecessary data that might not be immediately required.
javascript // Server-side publication Meteor.publish('userData', function () { // Return only necessary fields for the current user return Meteor.users.find({ _id: this.userId }, { fields: { emails: 1, profile: 1 } }); }); // Client-side subscription Meteor.subscribe('userData');
1.2 Optimize Data Loading
Use pagination and lazy-loading techniques when dealing with large datasets. Fetching only a limited amount of data initially and loading more as the user scrolls can lead to faster load times and a smoother user experience.
2. Improve Database Performance
Efficient database usage is crucial for optimal Meteor app performance. Follow these tips to enhance your database performance:
2.1 Create Indexes
Ensure that your MongoDB collections have appropriate indexes on frequently queried fields. Indexes help speed up the database queries and improve overall performance.
javascript // Example: Creating an index on the 'createdAt' field Posts.rawCollection().createIndex({ createdAt: -1 });
2.2 Use Oplog Tail for Production Deployment
In a production environment, enable oplog tailing for MongoDB. Oplog tailing allows Meteor to directly listen to the database’s oplog, reducing the latency of data updates and ensuring real-time reactivity.
javascript // Enable oplog tailing in the Meteor settings { ... "mongo": { "oplog": true } ... }
2.3 Batch Database Operations
When performing multiple database updates, use bulk operations instead of individual updates. This reduces the overhead associated with network communication and database transactions.
javascript // Example: Using bulkWrite for multiple updates const bulk = MyCollection.rawCollection().initializeUnorderedBulkOp(); bulk.find({ status: 'new' }).update({ $set: { status: 'processed' } }); bulk.find({ status: 'draft' }).remove(); bulk.execute();
3. Optimize Front-End Rendering
A well-optimized front-end can significantly enhance the user experience. Consider the following techniques to improve front-end rendering performance:
3.1 Use Virtual DOM and Reactive UI
Meteor uses a virtual DOM, which efficiently updates the UI without directly manipulating the real DOM. This approach results in faster rendering and better performance. Additionally, leverage Meteor’s reactivity to update UI elements automatically when data changes.
javascript // Example: Using reactive data source Template.myTemplate.helpers({ data() { return MyCollection.find({ status: 'published' }); } });
3.2 Code Splitting
Divide your front-end code into smaller, manageable chunks. Code splitting allows users to load only the necessary components when required, reducing the initial load time of your app.
javascript // Use dynamic imports to split code into separate chunks const MyComponent = dynamic(() => import('../components/MyComponent'));
3.3 Optimize Images and Assets
Compress and optimize images and other assets to reduce their size without compromising quality. Smaller assets lead to faster load times and better overall performance.
4. Implement Caching Strategies
Caching can significantly improve the responsiveness of your Meteor app by reducing the need to regenerate data on every request. Consider these caching strategies:
4.1 Client-Side Caching
Use client-side caching to store data that doesn’t change frequently, such as static content or user preferences. LocalStorage and SessionStorage are great options for client-side caching.
javascript // Example: Storing user preferences in localStorage localStorage.setItem('theme', 'dark');
4.2 Server-Side Caching
For dynamic data that is expensive to compute, utilize server-side caching using solutions like Redis or in-memory caching. This reduces the load on your database and speeds up responses.
javascript // Example: Using Redis for server-side caching const cachedData = RedisClient.get('cachedData'); if (!cachedData) { // Fetch data from the database and cache it const data = fetchDataFromDatabase(); RedisClient.set('cachedData', data); return data; } return cachedData;
5. Optimize JavaScript Code
Efficient JavaScript code is crucial for better Meteor app performance. Pay attention to the following aspects:
5.1 Profile and Benchmark
Regularly profile your app to identify performance bottlenecks and areas that need improvement. Tools like meteor –profile and browser DevTools can help you identify slow code.
5.2 Code Splitting (Again!)
Divide your JavaScript code into smaller modules and load them only when needed. This reduces the initial loading time and helps users access essential functionality faster.
javascript // Use dynamic imports for code splitting import('some-module').then((module) => { // Use the module });
Optimize Loops and Iterations
Be cautious with nested loops and large iterations, as they can consume significant processing power. Look for opportunities to optimize or parallelize these operations.
javascript // Example: Using map instead of forEach for improved performance const arr = [1, 2, 3]; const doubledArr = arr.map((item) => item * 2);
Conclusion
Optimizing the performance of your Meteor application is a continuous process that involves fine-tuning various aspects of your codebase, database, and front-end rendering. By minimizing data transfers, improving database usage, optimizing front-end rendering, implementing caching strategies, and writing efficient JavaScript code, you can ensure that your Meteor app delivers a seamless user experience even as it scales.
Keep monitoring your app’s performance regularly and be open to making further improvements as your app evolves. Happy optimizing!
Table of Contents
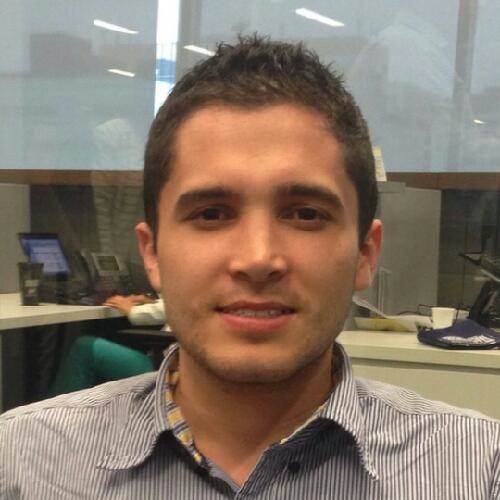
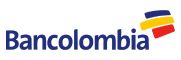