Reactive Programming with Meteor: Harnessing Real-time Data Updates
In the world of web development, user expectations have evolved to demand real-time updates and dynamic content. Users no longer want to manually refresh a webpage to see new data; they expect applications to update in real-time as changes occur. Reactive programming is a paradigm that enables developers to build highly responsive and interactive web applications by propagating data changes automatically. One framework that excels in reactive programming is Meteor. In this blog post, we will explore how to harness the power of reactive programming with Meteor to create web applications that provide real-time data updates.
What is Reactive Programming?
Reactive programming is a programming paradigm that focuses on propagating changes and reacting to them automatically. It enables developers to build applications that respond immediately to data changes without explicit instructions. In reactive programming, the system automatically updates the output whenever the input data changes, providing a seamless and dynamic user experience.
Introduction to Meteor
Meteor is an open-source full-stack JavaScript framework that enables rapid application development. It embraces the reactive programming paradigm and simplifies the process of building real-time web applications. Meteor allows developers to write both client-side and server-side code using JavaScript, providing a consistent development experience. It also includes a built-in data synchronization system that makes it easy to propagate changes to connected clients in real-time.
Setting Up a Meteor Project
Before we dive into reactive programming with Meteor, let’s start by setting up a new Meteor project. Follow these steps:
- Step 1: Install Meteor by visiting the Meteor website and following the installation instructions for your operating system.
- Step 2: Open a terminal or command prompt and navigate to the desired directory where you want to create your project.
- Step 3: Run the following command to create a new Meteor project:
shell meteor create my-app
- Step 4: Once the project is created, navigate into the project directory:
shell cd my-app
- Step 5: Start the Meteor development server:
shell meteor
Now you have a Meteor project set up and running locally. Open your browser and visit http://localhost:3000 to see the default Meteor application.
Working with Reactive Data Sources
In Meteor, the core building block of reactivity is the concept of reactive data sources. These data sources are objects that can be used to store and manage reactive data. Meteor provides several built-in reactive data sources, such as ReactiveVar, ReactiveDict, and ReactiveVarDict. Let’s explore each of them:
1. ReactiveVar:
ReactiveVar is a simple reactive data source that can hold a single value. It provides methods to get and set the value, and any reactive computations that depend on the ReactiveVar will automatically re-run when the value changes. Here’s an example:
javascript // Create a new ReactiveVar const count = new ReactiveVar(0); // Get the current value console.log(count.get()); // Output: 0 // Set a new value count.set(5); // The reactive computation will automatically re-run Tracker.autorun(() => { console.log(count.get()); // Output: 5 });
2. ReactiveDict:
ReactiveDict is a reactive data source that can hold key-value pairs. It allows you to store and manage multiple reactive values within a single object. Here’s an example:
javascript // Create a new ReactiveDict const user = new ReactiveDict(); // Set values user.set('name', 'John'); user.set('age', 25); // Get values console.log(user.get('name')); // Output: John console.log(user.get('age')); // Output: 25 // The reactive computations will automatically re-run Tracker.autorun(() => { console.log(user.get('name')); // Output: John (when name changes) });
3. ReactiveVarDict:
ReactiveVarDict is a combination of ReactiveVar and ReactiveDict. It allows you to store multiple reactive values, each with its own key. Here’s an example:
javascript // Create a new ReactiveVarDict const person = new ReactiveVarDict(); // Set values person.set('name', new ReactiveVar('John')); person.set('age', new ReactiveVar(25)); // Get values console.log(person.get('name').get()); // Output: John console.log(person.get('age').get()); // Output: 25 // The reactive computations will automatically re-run Tracker.autorun(() => { console.log(person.get('name').get()); // Output: John (when name changes) });
Building Real-Time Applications with Meteor
Now that we have a basic understanding of reactive data sources in Meteor, let’s see how we can leverage them to build real-time applications. Meteor provides a powerful data synchronization system called DDP (Distributed Data Protocol) that enables real-time communication between the client and the server.
To demonstrate real-time updates, let’s create a simple chat application where messages are instantly synchronized between connected clients. Follow these steps:
- Step 1: Create a new Meteor template to display the chat messages:
html <template name="chat"> <ul> {{#each messages}} <li>{{this}}</li> {{/each}} </ul> </template>
- Step 2: Define a ReactiveVar to store the chat messages:
javascript // client/main.js const messages = new ReactiveVar([]); Template.chat.helpers({ messages() { return messages.get(); }, });
- Step 3: Subscribe to the chat collection on the server:
javascript // server/main.js Meteor.publish('chat', function () { return Messages.find(); });
- Step 4: Define methods to insert new messages:
javascript // server/main.js Meteor.methods({ 'chat.insert'(message) { Messages.insert({ text: message }); }, });
- Step 5: Add an event handler to the chat template to handle form submission:
javascript // client/main.js Template.chat.events({ 'submit .chat-form'(event) { event.preventDefault(); const message = event.target.message.value; Meteor.call('chat.insert', message); event.target.message.value = ''; }, });
- Step 6: Finally, display the chat template in the main layout:
html <!-- client/main.html --> <body> {{> chat}} </body>
Now, when multiple users open the chat application and send messages, the messages will instantly appear on all connected clients, providing a real-time chatting experience.
Conclusion
Reactive programming with Meteor allows developers to build dynamic and responsive web applications by harnessing real-time data updates. By leveraging reactive data sources and the powerful data synchronization system provided by Meteor, developers can create applications that automatically update in real-time as data changes. Whether you’re building a chat application, a collaborative editing tool, or a dashboard that displays real-time analytics, Meteor’s reactive programming capabilities will empower you to deliver an exceptional user experience. Start exploring the world of reactive programming with Meteor today and unlock the full potential of real-time web applications.
Table of Contents
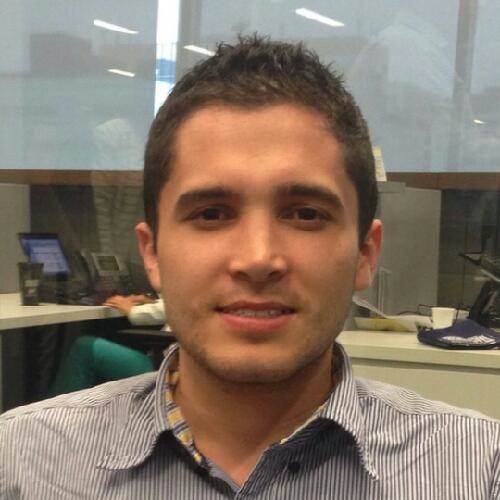
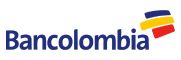