Scaling Meteor Applications: Strategies and Best Practices
As your Meteor application gains popularity and attracts a growing user base, it’s crucial to ensure that your system can handle the increased load and maintain optimal performance. Scaling your Meteor application requires careful planning, implementation of effective strategies, and adherence to best practices. In this blog, we will explore various techniques and tools to help you scale your Meteor applications and keep them running smoothly under heavy traffic.
Understanding the Need for Scaling
When your Meteor application experiences an increase in user traffic, it can result in slow response times, performance degradation, and even downtime. Scaling your application involves ensuring that it can handle the increased load efficiently without compromising user experience. This typically involves distributing the workload across multiple servers or optimizing the existing infrastructure.
Horizontal Scaling
Horizontal scaling involves adding more servers to handle increased traffic. It enables you to distribute the load across multiple instances, improving performance and scalability. Two common strategies for horizontal scaling in Meteor applications are load balancing and adopting a microservices architecture.
Load Balancing
Load balancing is a technique that evenly distributes incoming requests across multiple server instances. By using load balancers, you can ensure that the workload is distributed efficiently, preventing any single server from becoming overwhelmed. The following code snippet demonstrates how to configure a simple load balancer using the meteorhacks:cluster package:
javascript // server/main.js import { Cluster } from 'meteor/meteorhacks:cluster'; Cluster.allowPublicAccess('worker'); if (Cluster.isMaster) { // Set up the load balancer Cluster.setupMaster({ exec: 'path/to/worker.js', args: ['--worker'], }); // Fork worker processes based on the number of available CPU cores const numWorkers = require('os').cpus().length; for (let i = 0; i < numWorkers; i++) { Cluster.fork(); } } else { // Code for the worker process }
With load balancing in place, your Meteor application can handle more concurrent users by distributing the workload across multiple instances.
Microservices Architecture
Another approach to horizontal scaling is adopting a microservices architecture. In this architecture, your application is divided into small, independent services that can be deployed and scaled individually. Each microservice focuses on a specific functionality and can communicate with other services via APIs. This approach provides flexibility, scalability, and easier maintenance. To implement a microservices architecture in Meteor, you can use tools like ddp-server and ddp-client to establish communication between the services.
Vertical Scaling
Vertical scaling involves increasing the resources (CPU, RAM, etc.) of your existing servers to handle increased load. While horizontal scaling focuses on distributing the load across multiple servers, vertical scaling aims to improve the performance of individual servers. Here are two key areas to focus on when vertically scaling your Meteor application.
Optimizing Database Performance
Database queries can be a significant bottleneck in application performance. To optimize database performance, consider the following techniques:
Indexes: Ensure that the frequently accessed fields in your collections have appropriate indexes defined. This helps speed up query execution.
Avoiding N+1 queries: Use the replacelater:publish-with-relations package or similar techniques to fetch related documents efficiently in a single query instead of multiple queries.
javascript // server/publications.js import { Meteor } from 'meteor/meteor'; import { publishWithRelations } from 'meteor/replacelater:publish-with-relations'; Meteor.publish('usersWithPosts', function () { publishWithRelations({ handle: this, collection: Meteor.users, filter: {}, options: { sort: { createdAt: -1 }, limit: 10 }, mappings: [ { reverse: true, key: 'posts', collection: 'posts', filter: {}, options: { sort: { createdAt: -1 }, limit: 5 }, }, ], }); });
Data denormalization: In some cases, duplicating data across multiple collections can improve read performance by avoiding complex joins. However, this approach should be used judiciously and only when updates to denormalized data can be efficiently managed.
Caching Data
Caching data can significantly improve the performance of your Meteor application by reducing the load on the database. Consider using caching mechanisms like Redis or Memcached to store frequently accessed data in memory. This can be particularly useful for static or less frequently updated data.
javascript // server/main.js import { Meteor } from 'meteor/meteor'; import { Cache } from 'meteor/peerlibrary:redis-cache'; Meteor.methods({ 'getDataFromCache': function (key) { const cachedData = Cache.get(key); if (cachedData) { return cachedData; } else { const data = fetchFromDatabase(); // Fetch data from the database Cache.set(key, data); return data; } }, });
By caching data, you can reduce the number of database queries, resulting in improved application performance and reduced server load.
Managing Real-Time Updates
Meteor is known for its real-time capabilities, enabling seamless data synchronization between the server and clients. However, as your application scales, handling real-time updates efficiently becomes crucial. Consider the following strategies.
Using a Reactive Pub/Sub System
Leverage Meteor’s reactive data system to efficiently manage real-time updates. Use the observeChanges method to reactively monitor changes in the database and publish only the necessary updates to the clients.
javascript // server/main.js import { Meteor } from 'meteor/meteor'; import { Posts } from '/imports/api/posts'; Meteor.publish('latestPosts', function () { const handle = Posts.find({}, { sort: { createdAt: -1 }, limit: 10 }).observeChanges({ added: (id, fields) => this.added('posts', id, fields), changed: (id, fields) => this.changed('posts', id, fields), removed: (id) => this.removed('posts', id), }); this.ready(); this.onStop(() => handle.stop()); });
By using a reactive publication, you can ensure that the clients receive real-time updates efficiently, even under high load.
Optimizing Publication and Subscription
Review your publications and subscriptions to ensure that you are only sending the necessary data to the clients. Avoid sending large datasets that clients do not require, as this can impact performance. Use techniques like field projection and pagination to limit the amount of data transmitted.
javascript // client/main.js import { Meteor } from 'meteor/meteor'; Meteor.subscribe('latestPosts', { limit: 10 }); // ... // In your template or component const posts = Posts.find({}, { sort: { createdAt: -1 }, limit: 10 });
By optimizing your publications and subscriptions, you can reduce network traffic and improve the overall efficiency of real-time updates.
Optimizing Frontend Performance
Improving frontend performance is crucial for providing a smooth user experience, especially as your application scales. Here are some best practices to consider.
Code Splitting
Break down your application code into smaller chunks and load them asynchronously on demand. This technique, known as code splitting, helps reduce the initial load time by only fetching the code required for the current page or feature.
javascript // Using dynamic imports import { Meteor } from 'meteor/meteor'; const loadComponent = async () => { const { default: Component } = await import('/imports/components/MyComponent'); return Component; }; Meteor.startup(() => { const Component = loadComponent(); // ... });
By code splitting, you can ensure that only the necessary code is loaded, resulting in faster page loads and improved performance.
Minification and Compression
Minify and compress your JavaScript and CSS files to reduce their size. This helps improve the load time of your application, especially for users with slower internet connections.
javascript // In your build configuration (e.g., webpack) module.exports = { // ... optimization: { minimize: true, }, // ... };
Additionally, enable GZIP compression on your server to further reduce the size of transferred assets.
Monitoring and Performance Testing
To ensure your application is performing well and identify potential bottlenecks, it’s essential to monitor its performance regularly. Use tools like Kadira, Meteor APM, or your preferred monitoring solution to gain insights into your application’s behavior under different loads. Conduct performance tests to simulate high traffic scenarios and identify areas that require optimization.
Conclusion
Scaling your Meteor applications requires careful planning and implementation of effective strategies. By adopting horizontal and vertical scaling techniques, optimizing database performance, managing real-time updates efficiently, optimizing frontend performance, and monitoring your application, you can ensure that it can handle increased user load while providing a seamless user experience. Implement the strategies and best practices outlined in this blog to scale your Meteor applications successfully.
Table of Contents
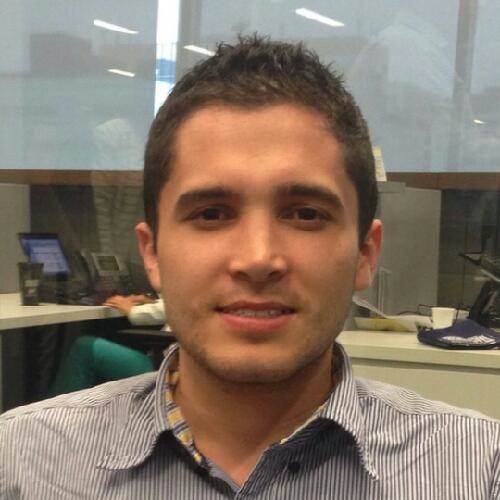
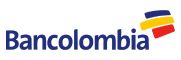