Meteor Security Best Practices: Protecting Your Application
Meteor is a powerful full-stack JavaScript framework that enables rapid application development. While its simplicity and convenience make it an excellent choice for building web applications, it’s essential to prioritize security to protect your application and its users. In this blog, we will explore crucial Meteor security best practices that can help you identify potential vulnerabilities, implement secure coding practices, and protect your application from malicious attacks. Let’s dive in!
1. Understanding Meteor Application Security
Before diving into specific security practices, it’s crucial to grasp the basic principles of Meteor application security. Understanding potential threats and attack vectors will help you design a robust security strategy.
Code Example:
javascript // Allow/deny rules for a Meteor collection MyCollection.allow({ insert: function(userId, doc) { // Implement your custom logic for write access here return false; // Return true to allow, false to deny }, update: function(userId, doc, fields, modifier) { // Implement your custom logic for update access here return false; // Return true to allow, false to deny }, remove: function(userId, doc) { // Implement your custom logic for delete access here return false; // Return true to allow, false to deny } });
2. Secure Data Handling and Validation
2.1 Sanitizing User Input
One of the most common sources of security vulnerabilities is inadequate user input validation. Always sanitize and validate user input to prevent various attacks like Cross-Site Scripting (XSS) and SQL injection.
Code Example:
javascript // Insecure example const userInput = '<script>alert("XSS Attack!");</script>'; MyCollection.insert({ content: userInput }); // Vulnerable to XSS // Secure example const sanitizedInput = sanitizeHTML(userInput); // Implement sanitizeHTML function MyCollection.insert({ content: sanitizedInput }); // Safe from XSS
2.2 Validating Data on the Server
Client-side validation is helpful for better user experience, but server-side validation is a must to ensure data integrity and security. Always validate data on the server, even if you’ve already done it on the client side.
Code Example:
javascript // Server-side validation Meteor.methods({ 'addItem': function(item) { check(item, { name: String, quantity: Match.Integer }); // Insert the item into the collection } });
2.3 Implementing HTTPS
Secure communication between clients and the server is crucial. Always use HTTPS to encrypt data in transit and prevent man-in-the-middle attacks.
Code Example:
javascript // Set up HTTPS in Meteor (using npm 'meteor-node-stubs') import { WebApp } from 'meteor/webapp'; import fs from 'fs'; const privateKey = fs.readFileSync('/path/to/privateKey'); const certificate = fs.readFileSync('/path/to/certificate'); WebApp.connectHandlers.use((req, res, next) => { res.setHeader('Strict-Transport-Security', 'max-age=63072000; includeSubDomains; preload'); next(); });
3. Authentication and Authorization
3.1 User Authentication
User authentication is a fundamental security measure to verify the identity of users accessing your application. Use Meteor’s built-in authentication system or implement a widely-used package like accounts-password for secure authentication.
Code Example:
javascript // Configuring user accounts import { Accounts } from 'meteor/accounts-base'; Accounts.config({ forbidClientAccountCreation: true // Disable client-side account creation });
3.2 Role-Based Authorization
Implement role-based authorization to control access to different parts of your application based on user roles. This can help protect sensitive data and functionalities.
Code Example:
javascript // Assigning roles to users import { Roles } from 'meteor/alanning:roles'; const userId = 'someUserId'; Roles.addUsersToRoles(userId, ['admin', 'editor']); // Assign roles to the user
3.3 Two-Factor Authentication (2FA)
Enhance security by implementing Two-Factor Authentication (2FA) for user accounts. This adds an extra layer of protection against unauthorized access.
Code Example:
javascript // Enabling 2FA for a user import { Accounts } from 'meteor/accounts-base'; const userId = 'someUserId'; Accounts.sendTwoFactorSecret(userId); // Sends a 2FA secret to the user's email/phone
4. Cross-Site Scripting (XSS) Prevention
4.1 Using Blaze Autoescaping
Blaze, Meteor’s default templating engine, automatically escapes HTML to prevent XSS attacks. However, you must remain vigilant and avoid using triple curly braces ({{{}}} ) unless you explicitly trust the content.
Code Example:
javascript // Insecure example const unsafeHTML = '<script>alert("XSS Attack!");</script>'; Template.myTemplate.helpers({ content: () => unsafeHTML // Vulnerable to XSS }); // Secure example const safeHTML = '<p>This is safe HTML content.</p>'; Template.myTemplate.helpers({ content: () => new Spacebars.SafeString(safeHTML) // Safe from XSS });
4.2 Applying Content Security Policy (CSP)
Implementing a Content Security Policy (CSP) is an additional layer of defense against XSS attacks by controlling the sources from which content can be loaded.
Code Example:
html <!-- HTML meta tag for CSP --> <meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self' 'unsafe-inline'; style-src 'self' 'unsafe-inline';">
5. Cross-Site Request Forgery (CSRF) Mitigation
5.1 Synchronizer Token Pattern
Prevent Cross-Site Request Forgery (CSRF) attacks by using the synchronizer token pattern. Generate a unique token for each user session and include it in requests that modify data.
Code Example:
javascript // Server-side route to generate CSRF token import { Random } from 'meteor/random'; Meteor.methods({ generateCSRFToken: function() { const token = Random.secret(); this.setUserId(token); // Store the token in the user session return token; } });
5.2 Using Meteor’s WebApp Package
Meteor’s WebApp package provides built-in CSRF protection. Ensure you are using the latest version of Meteor and the webapp package to take advantage of this protection.
Code Example:
javascript // No additional code needed. Meteor's webapp package handles CSRF protection automatically.
6. Denial of Service (DoS) Protection
6.1 Rate Limiting
Implement rate limiting to restrict the number of requests from a single client within a specific time window. This prevents brute-force attacks and DoS attempts.
Code Example:
javascript // Add the 'nimble:restivus' package (npm 'meteor-restivus' not needed for Meteor 1.12+) import { Restivus } from 'meteor/nimble:restivus'; // Rate-limiting for API endpoint const Api = new Restivus({ useDefaultAuth: true, apiPath: 'my-api', version: 'v1' }); Api.addRoute('someEndpoint', { get: { action: function() { // Implement your API logic here }, rateLimit: { numRequests: 10, timeInterval: 1000 // 10 requests per second } } });
6.2 Method Invocation Restrictions
Control access to expensive or sensitive methods to prevent DoS attacks. Limit the invocation rate for certain methods to maintain application performance and security.
Code Example:
javascript // Server-side method with rate limiting import { DDPRateLimiter } from 'meteor/ddp-rate-limiter'; Meteor.methods({ expensiveMethod: function() { // Expensive operation } }); // Rate limiting for the expensiveMethod const limitExpensiveMethod = 5; // Allow 5 invocations per connection per second DDPRateLimiter.addRule({ name: 'expensiveMethod', type: 'method' }, limitExpensiveMethod);
7. Protecting Sensitive Data
7.1 Hashing and Salting Passwords
Always hash and salt user passwords before storing them in the database. This ensures that even if the database is compromised, attackers cannot easily retrieve plaintext passwords.
Code Example:
javascript // Insecure example (DO NOT USE) const userPassword = 'password123'; const hashedPassword = md5(userPassword); // DO NOT use MD5 for password hashing // Secure example import { Accounts } from 'meteor/accounts-base'; const userPassword = 'password123'; const userId = Accounts.createUser({ username: 'username', password: userPassword });
7.2 Securing API Keys and Secrets
If your application uses external APIs or services, avoid hardcoding API keys or secrets in your code. Use environment variables or a secure configuration management system to store and retrieve sensitive information.
Code Example:
javascript // Insecure example (DO NOT USE) const apiKey = 'YOUR_API_KEY'; // Secure example (using npm 'settings' package) import { Meteor } from 'meteor/meteor'; import settings from 'meteor/rocketchat:settings'; const apiKey = Meteor.settings.private.apiKey;
8. Keeping Packages and Dependencies Updated
Regularly update your Meteor packages and dependencies to ensure you have the latest security patches. Use tools like npm audit to identify and address vulnerable packages.
Code Example:
bash # Check for vulnerabilities in your Meteor project meteor npm audit
9. Secure Deployment and Hosting
9.1 Proper File Permissions
Ensure that file permissions are set correctly on your server to prevent unauthorized access to sensitive files and directories.
Code Example:
bash # Set appropriate file permissions (example for Unix-based systems) chmod 600 private/key.pem # Restrict access to private key chmod 700 /path/to/project # Restrict access to project directory
9.2 Firewall Configuration
Configure your server’s firewall to allow only necessary incoming and outgoing traffic, minimizing the attack surface.
Code Example:
bash # Example for UFW (Uncomplicated Firewall) on Ubuntu sudo ufw allow OpenSSH # Allow SSH access sudo ufw enable # Enable the firewall
10. Logging and Monitoring
10.1 Centralized Logging
Implement centralized logging to monitor and analyze application logs effectively. This helps in identifying suspicious activities and potential security breaches.
Code Example:
javascript // Using npm 'winston' for centralized logging import { Meteor } from 'meteor/meteor'; import winston from 'winston'; const logger = winston.createLogger({ level: 'info', format: winston.format.simple(), transports: [ new winston.transports.File({ filename: 'app.log' }) ] }); // Usage example logger.info('User login attempt', { username: 'john_doe' });
10.2 Real-time Monitoring and Alerts
Set up real-time monitoring and alerts to respond promptly to security incidents. Tools like New Relic or Sentry can help you achieve this.
Code Example:
bash # Example for setting up Sentry (using npm 'sentry' package) meteor add sentry:meteor
Conclusion
Implementing robust security practices is essential for safeguarding your Meteor application and protecting your users’ data and privacy. By following these best practices, you can significantly reduce the risk of common security vulnerabilities and ensure a more secure and trustworthy application. Always stay vigilant, keep your dependencies up to date, and continuously monitor your application’s security to defend against emerging threats. Secure coding is not a one-time task; it’s an ongoing process that requires consistent effort and attention.
Table of Contents
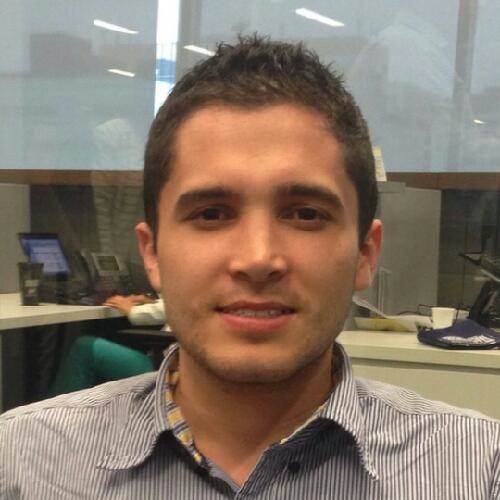
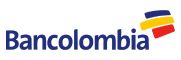