Testing Meteor Applications: Ensuring Quality and Reliability
In today’s fast-paced digital world, web applications play a vital role in delivering seamless user experiences. Meteor, a full-stack JavaScript framework, has gained popularity for its real-time capabilities and rapid development features. However, building complex applications without proper testing can lead to numerous issues, from minor bugs to catastrophic failures. Testing Meteor applications is crucial to ensure quality and reliability, which ultimately leads to happy users and satisfied stakeholders.
In this blog, we will delve into the world of testing Meteor applications. We will explore various testing methodologies, tools, and best practices to help you build robust and bug-free apps. Whether you’re a seasoned developer or a newcomer to Meteor, this guide will equip you with the knowledge and confidence to deliver high-quality applications.
1. Why Test Meteor Applications?
Testing is an essential part of the software development lifecycle. Proper testing provides several benefits for Meteor applications:
1.1. Bug Detection and Prevention
Testing allows you to identify bugs early in the development process, preventing them from reaching production. This reduces the likelihood of critical issues and enhances the overall stability of your application.
1.2. Improved Code Maintainability
Writing testable code often leads to better-structured and modular applications. This, in turn, improves code maintainability, making it easier for developers to understand and extend the codebase.
1.3. Regression Testing
As your application evolves with new features and bug fixes, regression testing ensures that existing functionalities continue to work as expected. It helps prevent the introduction of new bugs while addressing existing ones.
1.4. Enhanced User Experience
Testing ensures that your application behaves as intended, providing users with a seamless and error-free experience. A reliable application builds trust and loyalty among your user base.
1.5. Increased Developer Confidence
Knowing that your code is thoroughly tested gives developers confidence in their work. This confidence translates to quicker deployments and reduces the fear of introducing breaking changes.
2. Testing Methodologies for Meteor Applications
Before diving into testing tools and techniques, it’s essential to understand the different types of testing methodologies available for Meteor applications. Each serves a specific purpose in ensuring the overall quality of your codebase.
2.1. Unit Testing
Unit testing involves testing individual units or components of your application in isolation. In Meteor applications, this typically means testing functions, methods, or modules without relying on external dependencies. By isolating units, you can easily identify and fix issues within specific parts of your codebase.
Example of a unit test using the tinytest package:
javascript // Import the module or function to be tested import { sum } from '/imports/utils/math'; Tinytest.add('Math - Sum Function', (test) => { // Test the function with various inputs and expected outputs test.equal(sum(2, 2), 4); test.equal(sum(-1, 5), 4); test.equal(sum(0, 0), 0); });
2.2. Integration Testing
Integration testing evaluates how different parts of your Meteor application work together. It ensures that the integration points, such as communication between components or modules, function correctly and produce the expected outcomes.
Example of an integration test using practicalmeteor:mocha and chai packages:
javascript // Import the module to be tested import { getDataFromAPI } from '/imports/api/data'; describe('Data API Integration', () => { it('should fetch data from the API', () => { // Call the function and assert the response const result = getDataFromAPI(); chai.assert.isArray(result); chai.assert.isNotEmpty(result); }); });
2.3. End-to-End (E2E) Testing
End-to-End testing examines your Meteor application as a whole, simulating real user interactions to verify its behavior. E2E testing helps ensure that the entire application functions correctly, including user interfaces and backend services.
Example of an E2E test using cypress package:
javascript // Write Cypress E2E test to verify login functionality describe('Login Functionality', () => { it('should log in successfully with valid credentials', () => { cy.visit('/login'); cy.get('input[name="username"]').type('testuser'); cy.get('input[name="password"]').type('testpassword'); cy.get('button[type="submit"]').click(); cy.url().should('include', '/dashboard'); }); });
3. Testing Tools for Meteor Applications
Meteor provides a wide array of testing tools that simplify the testing process and seamlessly integrate with your development workflow. Here are some popular testing packages used in Meteor applications:
3.1. tinytest
tinytest is a lightweight testing framework that comes built-in with Meteor. It allows you to write simple and fast unit tests for your application. While it lacks some advanced features, it remains an excellent choice for testing core functionalities.
3.2. practicalmeteor:mocha
Mocha is a feature-rich testing framework that provides support for writing unit and integration tests in Meteor applications. The practicalmeteor:mocha package offers Meteor-specific enhancements and simplifies test setup.
3.3. chai
chai is an assertion library that works seamlessly with Mocha and other testing frameworks. It provides several styles of assertions, allowing you to choose the one that best fits your testing needs.
3.4. cypress
Cypress is a powerful E2E testing framework that enables developers to write fast, easy-to-understand tests. It comes with a user-friendly interface and real-time reloading, making it an ideal choice for testing modern web applications, including Meteor projects.
4. Best Practices for Testing Meteor Applications
To ensure effective testing, consider the following best practices when writing tests for your Meteor applications:
4.1. Test Coverage
Strive for comprehensive test coverage by testing all essential parts of your application. Focus on testing critical functionality, edge cases, and any potential points of failure.
4.2. Use Mocks and Stubs
When testing components that rely on external services or APIs, use mocks or stubs to simulate their behavior. This ensures that your tests remain isolated and do not depend on external factors.
4.3. Continuous Integration
Integrate testing into your continuous integration (CI) pipeline to automatically run tests whenever code changes are pushed. This ensures that tests are executed consistently, and any issues are detected early in the development process.
4. Regular Test Maintenance
As your application evolves, regularly update and maintain your tests. New features and code changes may require corresponding test adjustments to remain effective.
4.5. Test in Real Environments
Besides local development environments, test your application in production-like settings to identify potential issues that might arise in the real world.
Conclusion
Testing is an indispensable aspect of building reliable and high-quality Meteor applications. By employing appropriate testing methodologies, leveraging the power of testing tools, and following best practices, you can ensure that your application performs optimally and meets users’ expectations.
Remember, testing is not a one-time effort but an ongoing process that evolves with your application. Embrace testing as an integral part of your development workflow to deliver robust, bug-free, and delightful user experiences with your Meteor applications.
Table of Contents
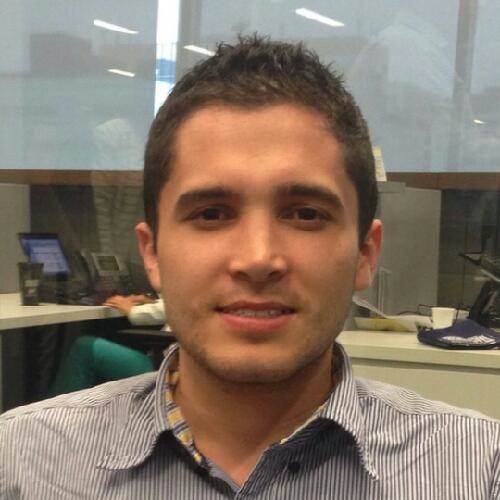
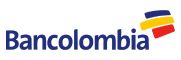