Meteor and WebRTC: Building Real-time Video Chat Applications
In today’s interconnected world, real-time communication has become an essential part of our daily lives. Video chat applications allow us to connect with friends, family, colleagues, and clients, enabling seamless face-to-face conversations regardless of physical distance. Meteor and WebRTC, when combined, form a powerful duo for building robust real-time video chat applications. In this blog, we will explore how to leverage Meteor’s capabilities and WebRTC’s peer-to-peer communication to create an immersive and efficient video chat experience.
What is Meteor?
Before diving into the specifics of building real-time video chat applications, let’s briefly introduce Meteor. Meteor is an open-source, full-stack JavaScript framework that simplifies the process of developing modern web and mobile applications. It offers an integrated development environment, real-time data synchronization, and seamless client-server communication. With its reactive nature, Meteor makes it easier to create real-time applications with automatic updates to the user interface as the data changes on the server.
What is WebRTC?
WebRTC, which stands for Web Real-Time Communication, is a set of technologies that enables peer-to-peer audio, video, and data communication between web browsers and mobile applications. It allows developers to add real-time communication features to their applications without the need for third-party plugins or extensions. WebRTC uses a secure and efficient protocol to establish direct connections between users, ensuring low latency and high-quality communication.
Getting Started with Meteor:
Before delving into WebRTC integration, let’s set up a basic Meteor application:
1. Install Meteor:
First, make sure you have Node.js installed. Then, install Meteor by running the following command in your terminal:
ruby $ curl https://install.meteor.com/ | sh
2. Create a new Meteor project:
To create a new Meteor project, use the following command:
shell $ meteor create video-chat-app $ cd video-chat-app
3. Run the application:
Start your Meteor application by executing the following command:
ruby $ meteor
Now, you have a basic Meteor application up and running. Let’s move on to integrating WebRTC for real-time video chat functionality.
Integrating WebRTC with Meteor:
Step 1: Installing necessary packages
To enable WebRTC functionality in our Meteor application, we need to install some packages. Meteor’s package management system makes this process easy. In your project directory, run the following commands:
csharp $ meteor add gwendall:webrtc $ meteor add session
The gwendall:webrtc package provides the necessary WebRTC integration, while the session package allows us to handle reactive data across the application.
Step 2: Setting up WebRTC configuration
Next, we need to configure WebRTC settings in our Meteor application. Create a new JavaScript file named webrtc.js in the client folder and add the following code:
javascript // client/webrtc.js Meteor.startup(() => { WebRTC.options.receiveMedia = { mandatory: { OfferToReceiveAudio: true, OfferToReceiveVideo: true } }; });
This configuration ensures that our application can both send and receive audio and video streams.
Step 3: Implementing video chat UI
Now, let’s create the user interface for our video chat application. In your client folder, create a new HTML file called video-chat.html and include the following code:
html <!-- client/video-chat.html --> <template name="videoChat"> <div class="container"> <video id="localVideo" autoplay></video> <video id="remoteVideo" autoplay></video> <button id="startButton">Start Call</button> <button id="hangupButton">Hang Up</button> </div> </template>
In this UI, we have two video elements for displaying the local video stream and the remote video stream, along with buttons to start and end the video call.
Step 4: Implementing video chat functionality
Now, let’s add the necessary JavaScript code to handle video chat functionality. Create a new JavaScript file called video-chat.js in the client folder and include the following code:
javascript // client/video-chat.js let localStream; let remoteStream; let peerConnection; function getLocalStream() { navigator.mediaDevices.getUserMedia({ video: true, audio: true }) .then(stream => { localStream = stream; document.getElementById('localVideo').srcObject = localStream; }) .catch(error => { console.error('Error accessing media devices:', error); }); } Template.videoChat.onCreated(function () { getLocalStream(); }); Template.videoChat.events({ 'click #startButton': function () { peerConnection = new webrtc.RTCPeerConnection(); peerConnection.addStream(localStream); peerConnection.onicecandidate = event => { if (event.candidate) { Meteor.call('sendIceCandidate', event.candidate); } }; peerConnection.onaddstream = event => { remoteStream = event.stream; document.getElementById('remoteVideo').srcObject = remoteStream; }; Meteor.call('createOffer', (error, offer) => { if (error) { console.error('Error creating offer:', error); } else { peerConnection.setLocalDescription(offer); Meteor.call('receiveOffer', offer); } }); }, 'click #hangupButton': function () { peerConnection.close(); remoteStream = null; document.getElementById('remoteVideo').srcObject = null; } });
In this code, we access the user’s media devices (camera and microphone) using navigator.mediaDevices.getUserMedia and display the local video stream on the page. We then create a new RTCPeerConnection and add the local stream to it. The onicecandidate event is used to send ICE candidates to the remote user, and the onaddstream event is used to display the remote video stream.
Step 5: Implementing server-side methods
Now, let’s implement the server-side methods to handle WebRTC signaling. Create a new JavaScript file called webrtc-server.js in the server folder and include the following code:
javascript // server/webrtc-server.js Meteor.methods({ sendIceCandidate: function (candidate) { // Send ICE candidate to the remote user }, createOffer: function () { // Create and return an offer to the calling user }, receiveOffer: function (offer) { // Receive and handle the offer from the calling user } });
In these methods, we will implement the logic to send and receive ICE candidates and offers between users to establish a peer-to-peer connection.
Conclusion
In this blog, we explored how to build real-time video chat applications using Meteor and WebRTC. Meteor’s reactivity and WebRTC’s peer-to-peer communication capabilities combine to create a powerful and efficient solution for seamless video communication. By following the steps outlined above, you can start developing your own real-time video chat application and enhance it further with additional features like chat messaging, screen sharing, and more. Now, go ahead and create the next generation of real-time video chat applications that bring people closer together, regardless of their geographical locations. Happy coding!
Table of Contents
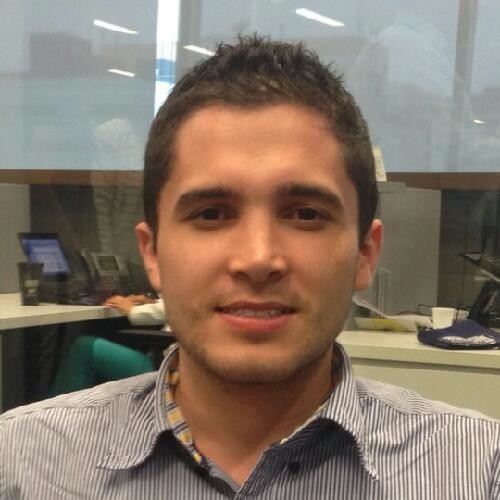
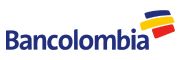