Optimizing NEXT.js Apps for Accessibility: A11y Best Practices
In today’s digital landscape, creating web applications that are accessible to all users is not just a best practice; it’s a legal requirement in many regions. Accessibility, often referred to as A11y (short for “accessibility”), ensures that your web content is usable by people with disabilities, including those who use screen readers, keyboard navigation, or other assistive technologies.
If you’re building web applications with NEXT.js, you’re already on the right track toward creating a fast and responsive user experience. However, optimizing NEXT.js apps for accessibility requires additional considerations and actions. In this guide, we’ll explore the best practices and techniques to make your NEXT.js apps inclusive and user-friendly for everyone.
1. Why Accessibility Matters
Before we dive into the specifics of optimizing NEXT.js apps for accessibility, it’s essential to understand why accessibility matters.
1.1. Inclusivity
Accessibility ensures that your web application can be used by everyone, regardless of their abilities or disabilities. By making your app accessible, you extend your reach to a broader audience, including people with disabilities who rely on assistive technologies.
1.2. Legal Compliance
Many countries have established laws and regulations that mandate web accessibility. Failing to comply with these regulations can lead to legal consequences, including lawsuits and fines.
1.3. Improved User Experience
Accessibility enhancements often lead to a better overall user experience for all users, not just those with disabilities. Well-structured and navigable apps are easier for everyone to use.
2. Getting Started with NEXT.js and Accessibility
Let’s start with the basics of making your NEXT.js app accessible. These initial steps will lay the foundation for a more inclusive user experience.
2.1. Use Semantic HTML
Semantic HTML tags provide meaning and structure to your web content. When building your app, use HTML elements like <header>, <nav>, <main>, <article>, and <footer> appropriately. These tags help screen readers and other assistive technologies understand the content’s hierarchy and purpose.
jsx // Bad: Using <div> instead of <nav> for navigation <div class="nav">...</div> // Good: Using <nav> for navigation <nav>...</nav>
2.2. Provide Descriptive Alt Text
Images are a crucial part of web content, but they can be challenging for users with visual impairments. Always include descriptive alt text for images to convey their meaning.
jsx // Bad: Missing alt text <img src="image.jpg" /> // Good: Descriptive alt text <img src="image.jpg" alt="A red bicycle parked next to a river." />
2.3. Keyboard Navigation
Ensure that all interactive elements, such as buttons and links, are navigable using the keyboard. Users should be able to tab through your app and interact with all components without relying on a mouse.
jsx // Bad: Unfocusable element <div onClick={handleClick}>Click me</div> // Good: Focusable button <button onClick={handleClick}>Click me</button>
2.4. Focus Styles
Make sure interactive elements have clear and visible focus styles. This helps users understand which element they are currently interacting with when navigating using the keyboard.
jsx /* Bad: Default focus style is unclear */ button:focus { outline: none; } /* Good: Clear and visible focus style */ button:focus { outline: 2px solid blue; }
3. Testing for Accessibility
Once you’ve implemented the fundamental accessibility features, it’s crucial to test your NEXT.js app to ensure it meets accessibility standards. Here are some tools and techniques to help you with testing:
3.1. Lighthouse
Lighthouse is an open-source tool for auditing web pages. It includes an accessibility audit that checks your app for common accessibility issues. To use Lighthouse, you can run it as a browser extension or through the command line.
bash # Run Lighthouse from the command line npx lighthouse https://your-website-url.com
3.2. Screen Readers
Testing your app with screen readers is essential because it simulates the experience of users with visual impairments. Popular screen readers include JAWS, NVDA, and VoiceOver (for macOS and iOS).
3.3. Keyboard Testing
Test your app using only the keyboard to ensure that all interactive elements are navigable and usable. This includes checking tab order and ensuring that keyboard focus remains visible.
jsx // Test your app using only the keyboard
4. Advanced A11y Techniques for NEXT.js
Beyond the basics, there are advanced techniques to further enhance the accessibility of your NEXT.js app.
4.1. ARIA (Accessible Rich Internet Applications)
WAI-ARIA (Web Accessibility Initiative – Accessible Rich Internet Applications) is a set of attributes you can add to your HTML elements to make them more accessible. ARIA roles and attributes help define the roles and properties of UI elements for assistive technologies.
jsx // Example: Adding an ARIA role to a button <button aria-label="Close" aria-hidden="true" role="button" onClick={closeModal}> ? </button>
4.2. Skip Links
Skip links are hidden links that allow users to skip repetitive content and navigate directly to the main content or other essential parts of your app. They are especially helpful for users who rely on screen readers.
jsx // Example: Skip link <a href="#main-content" className="skip-link"> Skip to main content </a> <main id="main-content"> {/* Main content */} </main>
4.3. Managing Focus
Managing focus is crucial to providing a seamless keyboard navigation experience. You can use JavaScript to control focus when opening and closing modal dialogs or navigation menus.
jsx // Example: Managing focus when opening a modal function openModal() { // Save current focus const focusedElement = document.activeElement; // Open modal and set focus to its first element modalElement.style.display = 'block'; modalElement.querySelector('input').focus(); // When modal is closed, return focus to the previously focused element modalElement.addEventListener('close', () => { focusedElement.focus(); }); }
Conclusion
Optimizing NEXT.js apps for accessibility (A11y) is not just a nice-to-have feature; it’s an essential part of building a modern, inclusive web application. By following these best practices and incorporating accessibility into your development process, you can ensure that your app is usable by a broader audience, complies with legal requirements, and provides a better user experience for all.
Remember that accessibility is an ongoing effort. Regularly test and improve your app’s accessibility features to ensure that it remains inclusive and user-friendly. By doing so, you contribute to a more accessible and equitable digital environment for everyone.
Table of Contents
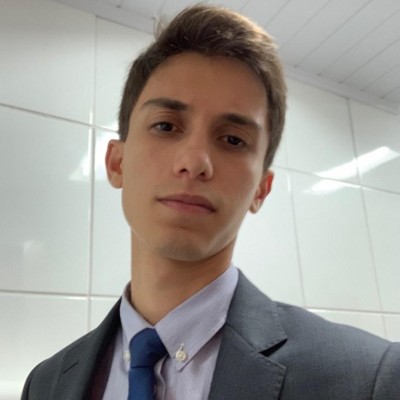
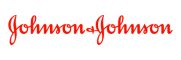