Adding PWA Capabilities to NEXT.js Apps: Offline Support and Push Notifications
Progressive Web Apps (PWAs) have transformed the way we build and deliver web applications. They provide a seamless experience by blending the best of web and mobile applications, allowing users to interact with your app even when offline and receiving timely push notifications. In this tutorial, we’ll explore how to integrate these powerful PWA capabilities into your NEXT.js apps. Specifically, we’ll cover offline support and push notifications, enhancing the reliability and engagement of your application.
1. Understanding Progressive Web Apps (PWAs)
Before diving into the technical aspects, let’s briefly understand what Progressive Web Apps (PWAs) are and why they matter. PWAs are a hybrid of traditional web pages and mobile applications, designed to provide an engaging and reliable experience to users. They leverage modern web technologies to offer features like offline access, smooth animations, and push notifications.
PWAs are built on three key principles:
- Responsive: PWAs are designed to work seamlessly on any device or screen size, ensuring a consistent user experience across various platforms.
- Connectivity-independent: One of the standout features of PWAs is their ability to function offline or in low-network conditions. This is achieved through caching strategies and service workers.
- App-like Experience: PWAs offer an app-like experience, including smooth animations and navigation, making them feel more native than traditional web apps.
2. Enabling Offline Support
2.1. Service Workers: The Key to Offline Capabilities
Service workers are at the core of enabling offline capabilities in PWAs. They are scripts that run in the background, separate from the web page, and act as a proxy between the web application and the network. Service workers can intercept and cache network requests, allowing your app to function even when the user is offline.
In a NEXT.js app, setting up a service worker involves a few steps:
1. Create a Service Worker File:
Create a new JavaScript file, e.g., service-worker.js, in your public directory. This file will contain the logic for caching and handling offline requests.
2. Register the Service Worker:
In your application code, register the service worker using the navigator.serviceWorker.register() method. This is typically done in the main JavaScript file of your app.
Here’s an example of how the registration might look:
javascript // main.js (or any other entry point) if ('serviceWorker' in navigator) { window.addEventListener('load', () => { navigator.serviceWorker.register('/service-worker.js') .then(registration => { console.log('Service Worker registered with scope:', registration.scope); }) .catch(error => { console.error('Service Worker registration failed:', error); }); }); }
3. Implement Service Worker Logic:
Inside your service-worker.js file, you’ll need to define how requests are cached and handled. You can use strategies like cache-first or network-first, depending on your app’s requirements. Here’s a simple example of caching with the cache-first strategy using the Cache API:
javascript // service-worker.js const CACHE_NAME = 'my-cache'; self.addEventListener('install', event => { event.waitUntil( caches.open(CACHE_NAME).then(cache => { return cache.addAll([ '/', '/index.html', '/styles.css', // Add more assets to cache ]); }) ); }); self.addEventListener('fetch', event => { event.respondWith( caches.match(event.request).then(response => { return response || fetch(event.request); }) ); });
With these steps, your NEXT.js app will now have offline support through the service worker. Users will be able to access the cached version of your app’s assets even when they are not connected to the internet.
3. Implementing Push Notifications
Push notifications are a powerful tool to engage users with timely updates and reminders, even when they are not actively using your app. To add push notification support to your NEXT.js app, follow these steps:
3.1. Request Notification Permissions
Before you can send push notifications, you need to request permission from the user. This is typically done when the user visits your app for the first time. Here’s how you can request notification permissions:
javascript // main.js if ('Notification' in window) { // Check if notifications are supported if (Notification.permission !== 'granted') { // Request permission Notification.requestPermission().then(permission => { if (permission === 'granted') { console.log('Notification permission granted'); // You can now subscribe to push notifications } else { console.warn('Notification permission denied'); } }); } }
3.2. Set Up a Push Notification Server
Push notifications require a server to send notifications to subscribed users. You’ll need to set up a server that can communicate with push notification APIs, such as the Web Push API. This server will handle the process of sending notifications to users’ devices.
3.3. Subscribe to Push Notifications
Once you have the server in place, you can subscribe users to push notifications when they grant permission. The subscription object contains information needed to send notifications to a specific user’s device.
javascript // main.js if ('serviceWorker' in navigator && 'PushManager' in window) { navigator.serviceWorker.ready.then(serviceWorkerRegistration => { serviceWorkerRegistration.pushManager.subscribe({ userVisibleOnly: true, applicationServerKey: 'your-application-server-key' }) .then(subscription => { console.log('Subscribed to push notifications:', subscription); // Send the subscription to your server }) .catch(error => { console.error('Error subscribing to push notifications:', error); }); }); }
3.4. Sending Push Notifications
With the user subscribed to push notifications, you can now send notifications from your server. This usually involves making an HTTP request to the push notification service’s API with the user’s subscription information and the notification content.
javascript // Example of sending push notifications from the server (Node.js) const webpush = require('web-push'); webpush.setVapidDetails( 'mailto:your@email.com', 'your-public-key', 'your-private-key' ); const subscription = /* User's subscription object */; const notificationPayload = { title: 'New Update', body: 'Check out the latest features!', icon: '/path/to/icon.png' }; webpush.sendNotification(subscription, JSON.stringify(notificationPayload)) .catch(error => { console.error('Error sending push notification:', error); });
Conclusion
Progressive Web Apps bring the best of both worlds, combining the reach of web applications with the engagement of native mobile apps. By adding offline support and push notifications to your NEXT.js app, you can enhance user experience and keep them engaged even in challenging network conditions. Service workers enable seamless offline access, ensuring your app remains functional when users are offline. Push notifications, on the other hand, provide a way to communicate with users beyond their active usage of the app.
As you implement these PWA capabilities, remember to consider user privacy and provide clear opt-in options for notifications. By following these best practices, you can create a more reliable, engaging, and user-friendly experience for your NEXT.js app users.
Table of Contents
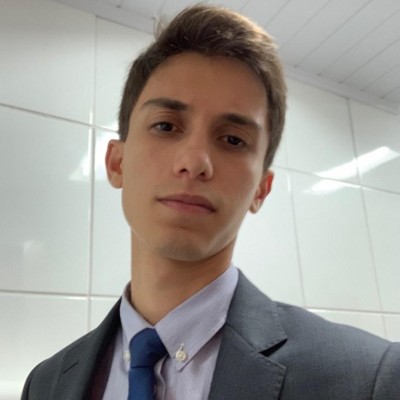
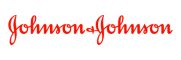