Using NEXT.js with GraphQL APIs: Apollo Server Integration
In today’s fast-paced world of web development, creating efficient and dynamic web applications is essential. GraphQL has gained popularity as a powerful tool for building APIs that allow clients to request exactly the data they need. To leverage the benefits of GraphQL in your web applications, it’s crucial to choose the right tools and frameworks. In this blog post, we’ll explore how to use NEXT.js with GraphQL APIs by seamlessly integrating Apollo Server.
1. Why Use NEXT.js with Apollo Server for GraphQL?
Before diving into the integration process, let’s understand why NEXT.js and Apollo Server make a great combination for building web applications with GraphQL.
1.1. Efficient Server-Side Rendering (SSR)
NEXT.js is a popular React framework that offers server-side rendering out of the box. SSR not only improves SEO but also enhances the initial load time of your web application. When combined with Apollo Server, you can fetch data efficiently on the server side, reducing client-side load times and improving overall performance.
1.2. Real-Time Data Fetching with GraphQL
Apollo Server is a robust GraphQL server implementation that allows you to define a single data schema for your application and fetch data in real-time. With GraphQL, you can request only the data you need, eliminating over-fetching and under-fetching issues commonly associated with REST APIs.
1.3. Strong Community Support
Both NEXT.js and Apollo Server have active and supportive communities. This means you’ll have access to a wealth of resources, tutorials, and packages to streamline your development process and troubleshoot any issues that may arise.
Now that we understand the advantages of using NEXT.js with Apollo Server for GraphQL, let’s dive into the integration process.
2. Setting Up a NEXT.js Project
If you don’t already have a NEXT.js project, you can set one up using the following steps:
2.1. Create a New NEXT.js Project
bash npx create-next-app my-nextjs-graphql-app
This command will create a new NEXT.js project with all the necessary files and dependencies.
2.2. Navigate to Your Project Folder
bash cd my-nextjs-graphql-app
2.3. Install Dependencies
Install the required dependencies, including Apollo Client and GraphQL:
bash npm install @apollo/client graphql
3. Integrating Apollo Client
Apollo Client is a powerful tool for making GraphQL queries and mutations in your React application. To integrate Apollo Client with your NEXT.js project, follow these steps:
3.1. Create an Apollo Client Instance
In your project directory, create a file named apolloClient.js to configure your Apollo Client instance:
javascript // apolloClient.js import { ApolloClient, InMemoryCache } from "@apollo/client"; const client = new ApolloClient({ uri: "https://your-graphql-api-url.com", // Replace with your GraphQL API URL cache: new InMemoryCache(), }); export default client;
Make sure to replace “https://your-graphql-api-url.com” with the actual URL of your GraphQL API.
3.2. Wrap Your App with ApolloProvider
In your pages/_app.js file, wrap your application with the ApolloProvider and pass the client you created as a prop:
javascript // pages/_app.js import { ApolloProvider } from "@apollo/client"; import client from "../apolloClient"; function MyApp({ Component, pageProps }) { return ( <ApolloProvider client={client}> <Component {...pageProps} /> </ApolloProvider> ); } export default MyApp;
With these steps, you’ve successfully integrated Apollo Client into your NEXT.js project.
4. Creating GraphQL Queries and Mutations
Now that you have Apollo Client set up, you can start creating GraphQL queries and mutations to fetch and manipulate data in your NEXT.js application. Here’s a basic example of how to do this:
4.1. Create a GraphQL Query
In a new file, let’s say queries.js, define a GraphQL query using the gql tag from graphql-tag:
javascript // queries.js import { gql } from "@apollo/client"; export const GET_ALL_POSTS = gql` query { getAllPosts { id title content } } `;
4.2. Use the Query in a Component
Now, you can use the useQuery hook from Apollo Client to fetch data in your React component. For example, in a file called Posts.js:
javascript // Posts.js import { useQuery } from "@apollo/client"; import { GET_ALL_POSTS } from "./queries"; function Posts() { const { loading, error, data } = useQuery(GET_ALL_POSTS); if (loading) return <p>Loading...</p>; if (error) return <p>Error: {error.message}</p>; return ( <div> {data.getAllPosts.map((post) => ( <div key={post.id}> <h2>{post.title}</h2> <p>{post.content}</p> </div> ))} </div> ); } export default Posts;
Here, we’re fetching a list of posts and displaying them in a component.
4.3. Fetching Data on Page Load
To fetch data on page load, you can use NEXT.js’s getServerSideProps or getStaticProps functions in your page components. For example, in a file called index.js:
javascript // pages/index.js import Posts from "../components/Posts"; function Home() { return ( <div> <h1>Latest Posts</h1> <Posts /> </div> ); } export default Home;
With this setup, your NEXT.js application will fetch and display the latest posts when a user visits the homepage.
5. Handling GraphQL Mutations
In addition to queries, you can use GraphQL mutations to modify data on the server. Here’s how you can handle mutations in your NEXT.js application:
5.1. Create a Mutation
In a new file, such as mutations.js, define a GraphQL mutation using the gql tag:
javascript // mutations.js import { gql } from "@apollo/client"; export const CREATE_POST = gql` mutation createPost($title: String!, $content: String!) { createPost(title: $title, content: $content) { id title content } } `;
This mutation allows you to create a new post with a title and content.
5.2. Use the Mutation in a Component
Now, you can use the useMutation hook from Apollo Client to perform the mutation in your React component. For example, in a file called CreatePost.js:
javascript // CreatePost.js import { useState } from "react"; import { useMutation } from "@apollo/client"; import { CREATE_POST } from "./mutations"; function CreatePost() { const [title, setTitle] = useState(""); const [content, setContent] = useState(""); const [createPost] = useMutation(CREATE_POST); const handleCreatePost = async () => { try { const { data } = await createPost({ variables: { title, content }, }); console.log("Post created:", data.createPost); } catch (error) { console.error("Error creating post:", error); } }; return ( <div> <h2>Create a New Post</h2> <input type="text" placeholder="Title" value={title} onChange={(e) => setTitle(e.target.value)} /> <textarea placeholder="Content" value={content} onChange={(e) => setContent(e.target.value)} /> <button onClick={handleCreatePost}>Create Post</button> </div> ); } export default CreatePost;
This component provides a form to create a new post and sends a mutation to the server when the “Create Post” button is clicked.
Conclusion
In this blog post, we’ve explored how to seamlessly integrate Apollo Server with NEXT.js to build powerful and efficient web applications using GraphQL APIs. We’ve covered the advantages of using NEXT.js and Apollo Server, setting up a NEXT.js project, integrating Apollo Client, and creating GraphQL queries and mutations.
With this knowledge, you have a solid foundation for building modern web applications that leverage the benefits of GraphQL for efficient data fetching and manipulation. The combination of NEXT.js and Apollo Server empowers you to create high-performance web apps with real-time capabilities while enjoying the flexibility and simplicity of GraphQL.
Start building your own GraphQL-powered web application today and unlock a new level of efficiency and interactivity for your users. Happy coding!
Table of Contents
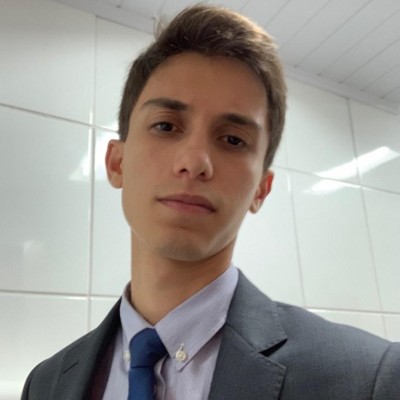
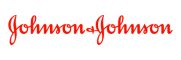