Implementing Authentication and Authorization in NEXT.js with Auth0
In the world of web development, security is of paramount importance. Protecting user data and ensuring the right people have access to specific parts of your application are key considerations. This is where authentication and authorization come into play. In this tutorial, we will explore how to implement authentication and authorization in a NEXT.js application using Auth0, a powerful and user-friendly authentication and authorization platform.
1. Understanding Authentication and Authorization
Authentication and authorization are two fundamental concepts in web security. Authentication is the process of verifying the identity of a user, typically through the use of credentials like usernames and passwords. Once a user’s identity is verified, authorization comes into play. Authorization determines what actions and resources a user is allowed to access based on their roles and permissions.
2. Introducing Auth0
Auth0 is a powerful authentication and authorization platform that provides developers with tools to add secure login, sign-up, and user management functionalities to their applications. It simplifies the process of integrating third-party authentication providers like Google, Facebook, and more. Additionally, Auth0 allows you to define user roles and permissions for fine-grained access control.
3. Setting Up Your NEXT.js Application
Before we dive into the implementation, make sure you have a NEXT.js application up and running. You can create a new NEXT.js project using the following commands:
bash npx create-next-app my-auth-app cd my-auth-app
4. Implementing Authentication with Auth0
4.1. Creating an Auth0 Account
To get started, you’ll need an Auth0 account. Go to the Auth0 website and sign up for an account. Once you’re logged in, you’ll need to create a new application in the Auth0 Dashboard. This application will represent your NEXT.js app.
4.2. Configuring Auth0 for Your Application
After creating the application in Auth0, you’ll receive credentials such as the Client ID and Client Secret. These credentials are required to configure Auth0 in your NEXT.js app. Create a .env.local file in the root of your project and add the following lines:
env AUTH0_DOMAIN=your-auth0-domain AUTH0_CLIENT_ID=your-auth0-client-id AUTH0_CLIENT_SECRET=your-auth0-client-secret AUTH0_REDIRECT_URI=http://localhost:3000/api/callback AUTH0_LOGOUT_REDIRECT_URI=http://localhost:3000
Replace your-auth0-domain, your-auth0-client-id, and your-auth0-client-secret with the corresponding values from your Auth0 application settings.
4.3. Adding Auth0 SDK to Your NEXT.js App
Auth0 provides an SDK that makes it easy to integrate authentication into your NEXT.js app. Install the Auth0 SDK package using the following command:
bash npm install @auth0/auth0-react
4.4. Creating Authentication Components
Now it’s time to create the necessary authentication components. Create a folder named auth in your project’s root directory. Inside the auth folder, create a file named auth0.js. This file will contain the configuration for the Auth0Provider. Here’s a basic example of what the auth0.js file might look like:
jsx // auth/auth0.js import { Auth0Provider } from '@auth0/auth0-react'; import { useRouter } from 'next/router'; const AuthProvider = ({ children }) => { const router = useRouter(); const onRedirectCallback = (appState) => { router.push(appState?.returnTo || '/'); }; return ( <Auth0Provider domain={process.env.AUTH0_DOMAIN} clientId={process.env.AUTH0_CLIENT_ID} redirectUri={process.env.AUTH0_REDIRECT_URI} onRedirectCallback={onRedirectCallback} > {children} </Auth0Provider> ); }; export default AuthProvider;
In this component, we’re using the Auth0Provider from the Auth0 SDK. We’re also using the useRouter hook from Next.js to handle redirection after authentication.
5. Implementing Authorization with Auth0
5.1. Defining User Roles and Permissions
Auth0 allows you to define roles and permissions for your users. Roles represent different user groups, and permissions define what actions each role can perform. You can define roles and permissions in the Auth0 Dashboard under the “Users & Roles” section.
5.2. Securing Routes Based on Roles
With roles and permissions defined, you can now secure your routes based on user roles. For example, if you have a dashboard page that should only be accessible to users with the “admin” role, you can create a Higher Order Component (HOC) to handle this:
jsx // components/requireAdmin.js import { useAuth0 } from '@auth0/auth0-react'; const RequireAdmin = ({ children }) => { const { user, isAuthenticated } = useAuth0(); if (!isAuthenticated) { return <div>Loading...</div>; } if (user && user['https://your-auth0-namespace/roles'].includes('admin')) { return children; } return <div>Access denied.</div>; }; export default RequireAdmin;
In this example, we’re using the useAuth0 hook to check if the user is authenticated and whether they have the “admin” role in their claims. If both conditions are met, the children components (i.e., the protected content) are rendered. Otherwise, an “Access denied” message is shown.
6. Adding a Dashboard Page
Now that you have implemented authentication and authorization, let’s create a simple dashboard page that is accessible only to users with the “admin” role.
Create a file named dashboard.js in your pages directory:
jsx // pages/dashboard.js import { useAuth0 } from '@auth0/auth0-react'; import RequireAdmin from '../components/requireAdmin'; const Dashboard = () => { const { user, isAuthenticated } = useAuth0(); return ( <div> <h1>Dashboard</h1> {isAuthenticated && ( <RequireAdmin> <p>Welcome, {user.name}!</p> <p>This content is only visible to admins.</p> </RequireAdmin> )} </div> ); }; export default Dashboard;
In this example, we’re using the RequireAdmin component we created earlier to wrap the content that should only be accessible to admins.
Conclusion
In this tutorial, we’ve explored how to implement authentication and authorization in a NEXT.js application using Auth0. By integrating Auth0, you can enhance the security of your application while providing a seamless and user-friendly authentication experience. We covered setting up an Auth0 account, configuring Auth0 for your app, and using the Auth0 SDK to create authentication components. Additionally, we demonstrated how to define user roles, permissions, and secure routes based on roles. With these techniques in your toolkit, you’re well-equipped to build secure and access-controlled applications with NEXT.js and Auth0.
Table of Contents
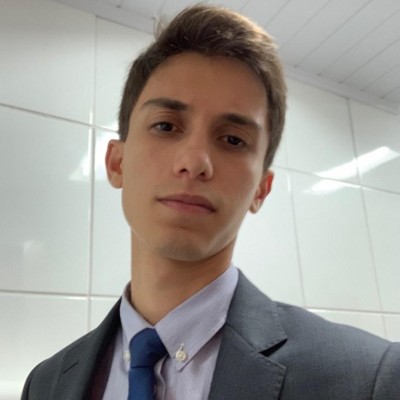
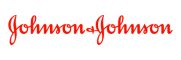